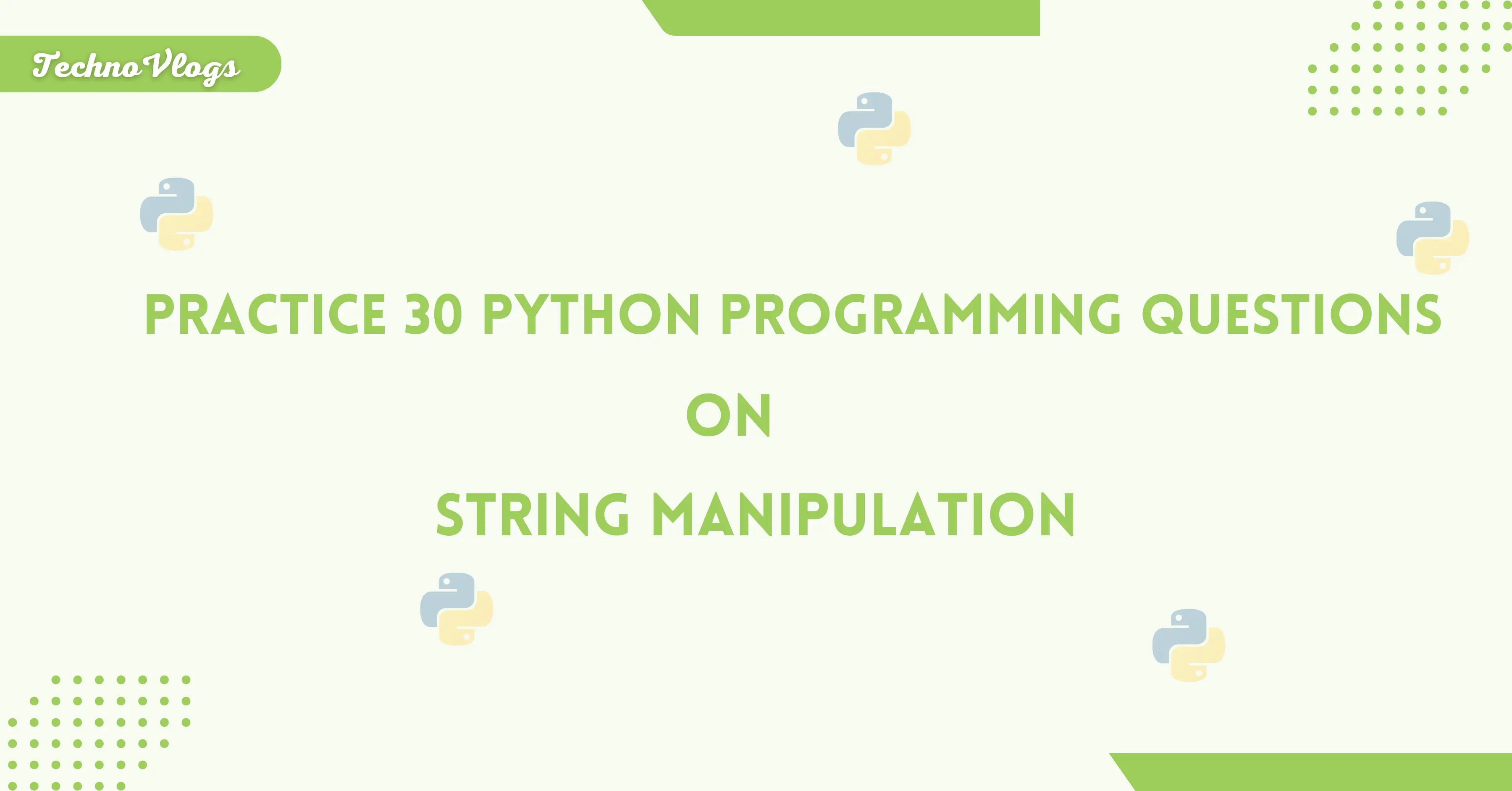
Practice Top 30 Python Programming Questions on String Manipulation
Q1. Write a program to take a string as input and convert all its characters to uppercase using a string method.
- Input: "hello world"
- Expected Output: "HELLO WORLD"
Q2. Write a program to count the number of times a specific substring appears in a given string.
- Input: "banana", "a"
- Expected Output: 3
Q3. Write a program to find the position of the first occurrence of a given character in a string.
- Input: "python programming", "p"
- Expected Output: 0
Q4. Write a program to replace all occurrences of a substring in a string with another substring.
- Input: "I love apples", "apples", "oranges"
- Expected Output: "I love oranges"
Q5. Write a program to check if the given string contains only alphanumeric characters (letters and numbers).
- Input: "Python123"
- Expected Output: True
Q6. Write a program to split a string into a list of words using spaces as the delimiter.
- Input: "Learn Python Programming"
- Expected Output: ['Learn', 'Python', 'Programming']
Q7. Write a program to join a list of strings into a single string, separated by a given delimiter.
- Input: ["a", "b", "c"], "-"
- Expected Output: "a-b-c"
Q8. Write a program to format a string using f-strings to display a person's name and age.
- Input: "Alice", 25
- Expected Output: "Alice is 25 years old"
Q9. Write a program to check if a string starts with a specific prefix.
- Input: "banana", "ban"
- Expected Output: True
Q10. Write a program to remove leading and trailing whitespace from a string.
- Input: " hello "
- Expected Output: "hello"
Q11. Write a program to validate if the given string is a valid email address using regular expressions.
- Input: "example@mail.com"
- Expected Output: True
Q12. Write a program to extract all numeric sequences from a given string using regular expressions.
- Input: "abc123xyz456"
- Expected Output: ['123', '456']
Q13. Write a program to check if a string contains only alphabetic characters using regular expressions.
- Input: "PythonProgramming"
- Expected Output: True
Q14. Write a program to find all words in a string that start with a given letter using regular expressions.
- Input: "apple banana apricot berry", "a"
- Expected Output: ['apple', 'apricot']
Q15. Write a program to replace all digits in a string with a specified symbol using regular expressions.
- Input: "I have 2 cats and 3 dogs", "#"
- Expected Output: "I have # cats and # dogs"
Q16. Write a program to search for the first occurrence of a numeric sequence in a given string using regular expressions.
- Input: "hello123world", r'\d+'
- Expected Output: "123"
Q17. Write a program to split a string into a list of words using multiple delimiters (spaces, commas, or semicolons).
- Input: "one,two;three", r'[;,\s]+'
- Expected Output: ['one', 'two', 'three']
Q18. Write a program to validate if the given string is a valid URL using regular expressions.
- Input: "https://example.com"
- Expected Output: True
Q19. Write a program to extract the domain name from a given email address using regular expressions.
- Input: "user@example.com"
- Expected Output: "example.com"
Q20. Write a program to check if a string starts with a specific pattern using regular expressions.
- Input: "123abc", r'^\d+'
- Expected Output: "123"
Q21. Write a program to check if a string is in a specific Unicode Normal Form (NFC or NFD).
- Input: "café", "NFC"
- Expected Output: True
Q22. Write a program to remove accents and convert a Unicode string to its ASCII equivalent.
- Input: "naïve"
- Expected Output: "naive"
Q23. Write a program to decode a Unicode string from its byte representation.
- Input: b'\xc3\xa9'
- Expected Output: "é"
Q24. Write a program to count the number of characters in a Unicode string, including special characters like emojis.
- Input: "hello 🌎"
- Expected Output: 7
Q25. Write a program to convert a string into a list of its Unicode code points.
- Input: "hello"
- Expected Output: [104, 101, 108, 108, 111]
Q26. Write a program to generate a string from a list of Unicode code points.
- Input: [104, 101, 108, 108, 111]
- Expected Output: "hello"
Q27. Write a program to remove all accents from a given Unicode string.
- Input: "café"
- Expected Output: "cafe"
Q28. Write a program to check if the given string contains any emoji characters.
- Input: "Hello 🌎"
- Expected Output: True
Q29. Write a program to normalize a Unicode string into a specific form (e.g., NFC or NFD).
- Input: "café", "NFD"
- Expected Output: "café"
Q30. Write a program to compare two Unicode strings that might be in different normalized forms.
- Input: "café", "cafe\u0301"
- Expected Output: True

Bikki Singh
Hi, I am the instructor of TechnoVlogs. I have a strong love for programming and enjoy teaching through practical examples. I made this site to help people improve their coding skills by solving real-world problems. With years of experience, my goal is to make learning programming easy and fun for everyone. Let's learn and grow together!