
30 Python Programming Questions on Defining and Calling Function
Q 1. Define a function that prints "Hello, World!" and call it.
Expected Output:
Hello, World!
Q 2. Define a function that takes a number and prints it. Call it with the number 10.
Expected Output:
10
Q 3. Define a function that returns the square of a number. Call it with the number 4.
Expected Output:
16
Q 4. Define a function that adds two numbers and returns the result. Call it with numbers 3 and 5.
Expected Output:
8
Q 5. Define a function that prints a greeting message with a name passed as an argument. Call it with the name "Alice".
Expected Output:
Hello, Alice!
Q 6. Define a function that calculates the factorial of a number and call it with the number 5.
Expected Output:
120
Q 7. Define a function that checks if a number is even or odd and call it with the number 7.
Expected Output:
Odd
Q 8. Define a function that returns the maximum of three numbers and call it with numbers 1, 4, and 2.
Expected Output:
4
Q 9. Define a function that concatenates two strings and call it with the strings "Hello" and "World".
Expected Output:
Hello World
Q 10. Define a function that takes a list and returns its length. Call it with a list [1, 2, 3, 4, 5].
Expected Output:
5
Q 11. Define a function that converts a temperature from Celsius to Fahrenheit and call it with the temperature 25°C.
Expected Output:
77.0
Q 12. Define a function that prints a multiplication table for a given number up to 10. Call it with the number 3.
Expected Output:
3 x 1 = 3
3 x 2 = 6
3 x 3 = 9
...
3 x 10 = 30
Q 13. Define a function that returns whether a given year is a leap year or not. Call it with the year 2024.
Expected Output:
True
Q 14. Define a function that calculates the sum of numbers in a list. Call it with [1, 2, 3, 4, 5].
Expected Output:
15
Q 15. Define a function that checks if a number is positive, negative, or zero. Call it with the number -8.
Expected Output:
Negative
Q 16. Define a function that takes two arguments and returns their product. Call it with 6 and 7.
Expected Output:
42
Q 17. Define a function that reverses a string. Call it with "Python".
Expected Output:
nohtyP
Q 18. Define a function that calculates the area of a rectangle given its width and height. Call it with width 4 and height 5.
Expected Output:
20
Q 19. Define a function that returns the length of a string. Call it with the string "Hello".
Expected Output:
5
Q 20. Define a function that returns the smaller of two numbers. Call it with 8 and 12.
Expected Output:
8
Q 21. Define a function that counts the number of vowels in a string. Call it with "OpenAI".
Expected Output:
3
Q 22. Define a function that returns the first element of a list. Call it with [10, 20, 30].
Expected Output:
10
Q 23. Define a function that returns the last element of a list. Call it with [1, 2, 3, 4].
Expected Output:
4
Q 24. Define a function that returns whether a given number is a multiple of 3. Call it with 9.
Expected Output:
True
Q 25. Define a function that returns the average of a list of numbers. Call it with [4, 8, 15, 16, 23, 42].
Expected Output:
18.0
Q 26. Define a function that returns a string in uppercase. Call it with "hello".
Expected Output:
HELLO
Q 27. Define a function that replaces spaces in a string with underscores. Call it with "hello world".
Expected Output:
hello_world
Q 28. Define a function that checks if a string is a palindrome. Call it with "madam".
Expected Output:
True
Q 29. Define a function that returns the number of characters in a string. Call it with "Python".
Expected Output:
6
Q 30. Define a function that converts a string to a list of characters. Call it with "abc".
Expected Output:
['a', 'b', 'c']
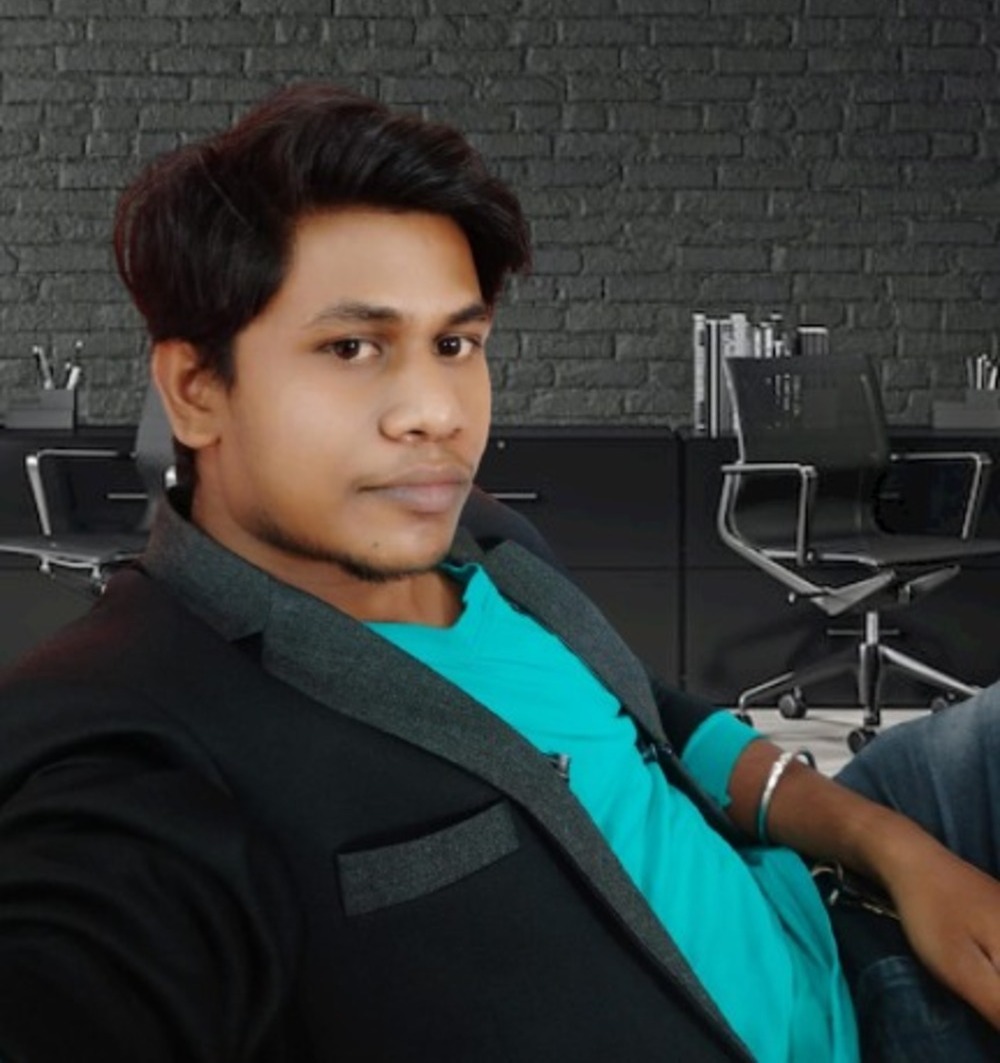
Bikki Singh
Hi, I am the instructor of TechnoVlogs. I have a strong love for programming and enjoy teaching through practical examples. I made this site to help people improve their coding skills by solving real-world problems. With years of experience, my goal is to make learning programming easy and fun for everyone. Let's learn and grow together!