
30 Python Questions on Loop Control (break, continue, pass)
Q 1. Print numbers from 1 to 10 but stop when the number is 5.
Expected Output:
1
2
3
4
Q 2. Print numbers from 1 to 10 but skip the number 5.
Expected Output:
1
2
3
4
6
7
8
9
10
Q 3. Use pass in a loop where no action is required.
Q 4. Print numbers from 1 to 10, but stop the loop if a number is greater than 7.
Expected Output:
1
2
3
4
5
6
7
Q 5. Print numbers from 1 to 10 but skip even numbers.
Expected Output:
1
3
5
7
9
Q 6. Use pass to create an empty loop.
Q 7. Print numbers from 1 to 10, but stop the loop if a number is divisible by 4.
Expected Output:
1
2
3
Q 8. Print numbers from 1 to 10 but skip numbers that are multiples of 3.
Expected Output:
1
2
4
5
7
8
10
Q 9. Print "Even" for even numbers and "Odd" for odd numbers from 1 to 5.
Expected Output:
Odd
Even
Odd
Even
Odd
Q 10. Use break to exit a loop when a specific condition is met.
Q 11. Print numbers from 1 to 10 but skip numbers that are less than 5.
Expected Output:
5
6
7
8
9
10
Q 12. Use pass in a conditional statement within a loop.
Q 13. Print numbers from 1 to 10 but stop if a number is equal to 7.
Expected Output:
1
2
3
4
5
6
Q 14. Print numbers from 1 to 10 but skip numbers divisible by 2.
Expected Output:
1
3
5
7
9
Q 15. Use pass in a loop to maintain structure.
Q 16. Print numbers from 1 to 10, but stop and exit the loop when reaching 8.
Expected Output:
1
2
3
4
5
6
7
Q 17. Print all characters in a string but skip vowels.
string = "hello world"
Expected Output:
hll wrld
Q 18. Use break to stop a loop when a user inputs "exit".
Q 19. Skip printing numbers divisible by 4 in a range from 1 to 15.
Expected Output:
1
2
3
5
6
7
9
10
11
13
14
15
Q 20. Use pass in a loop
Q 21. Print numbers from 1 to 10, but if a number is equal to 3 or 7, skip it.
Expected Output:
1
2
4
5
6
8
9
10
Q 22. Print the first 5 multiples of 3, but skip the number 9.
Expected Output:
3
6
12
15
18
Q 23. Print the sum of numbers from 1 to 10, but stop if the sum exceeds 25.
Expected Output:
30
Q 24. Print numbers from 1 to 10, but skip the loop iteration if a number is greater than 5.
Expected Output:
1
2
3
4
5
Q 25. Use break to exit a loop when the loop has executed 3 times.
Expected Output:
1
2
3
Q 26. Print numbers from 1 to 10, but use pass for even numbers.
Expected Output:
1
3
5
7
9
Q 27. Print all characters in a string, but stop if a space is encountered.
Q 28. Print numbers from 1 to 10, but skip numbers that are not divisible by 2 or 3.
Expected Output:
2
3
4
6
8
9
10
Q 29. Print numbers from 1 to 10, but use pass for numbers greater than 8.
Expected Output:
1
2
3
4
5
6
7
8
Q 30. Print the first 10 numbers of the sequence, but skip numbers that are less than 5.
Expected Output:
5
6
7
8
9
10
11
12
13
14
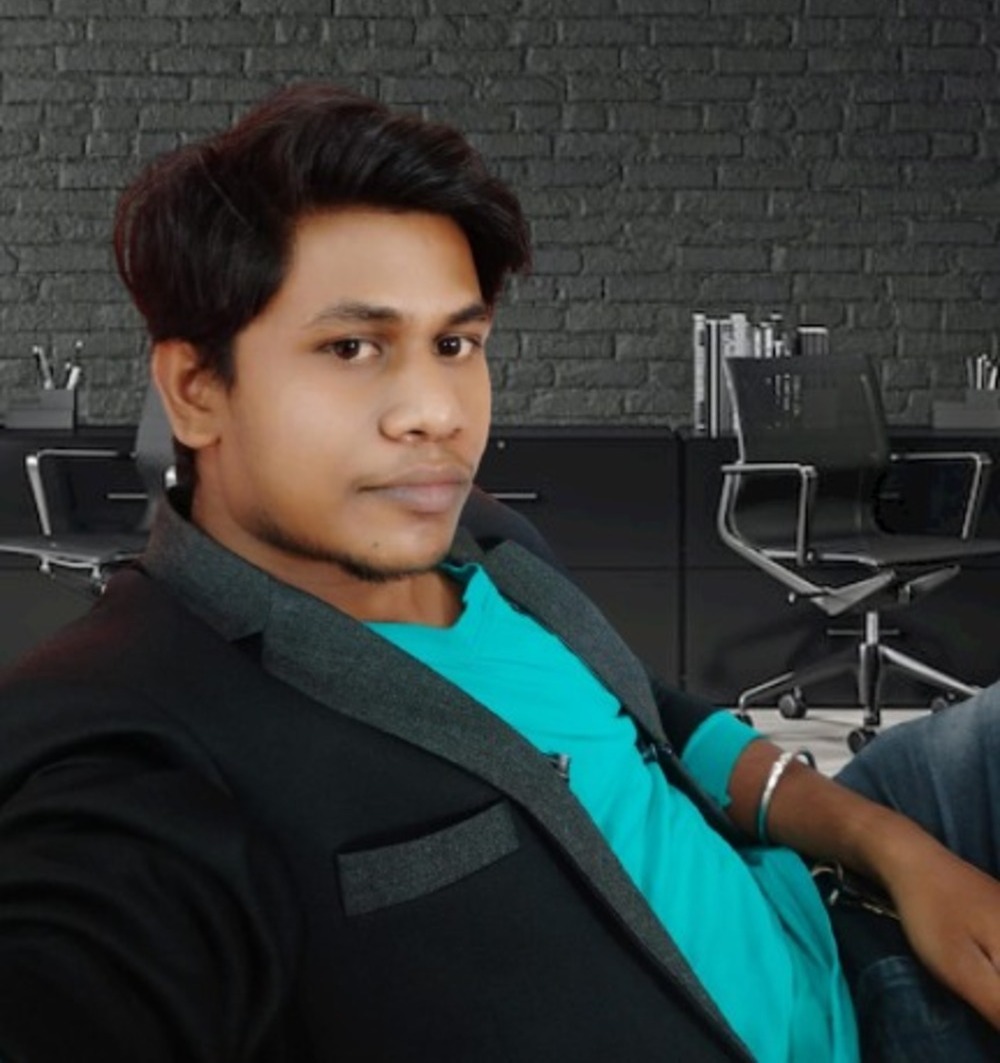
Bikki Singh
Hi, I am the instructor of TechnoVlogs. I have a strong love for programming and enjoy teaching through practical examples. I made this site to help people improve their coding skills by solving real-world problems. With years of experience, my goal is to make learning programming easy and fun for everyone. Let's learn and grow together!