
30 Easy Python programming questions on Basic Syntax and Structure
Q 1. Write a Program to print "Hello, World!" in Python.
Expected Output:
Hello, World!
Q 2. Write a Program to assign the value 5 to a variable x and print it.
Expected Output:
5
Q 3. Write a Python program to swap two variables.
Q 4. Write a Python program to add two numbers.
Q 5. Write a Python program to check if a number is positive or negative.
Q 6. Write a Python program to print the current year.
Expected Output:
2024
Q 7. Write a Python program to calculate the area of a rectangle.
Input length = 5 and width = 3
Expected Output
15
Q 8. Write a Python program to print the square of a number.
Q 9. Write a Python program to print the cube of a number.
Q 10. Write a Python program to print the remainder when a number is divided by another.
Q 11. Write a Python program to print the type of a variable.
Expected Output:
Q 12. Write a Python program to print the sum of three numbers.
Inputs: a = 2, b = 3, c = 5
Expected Output:
10
Q 13. Write a Python program to check if a number is even or odd.
Q 14. Write a Python program to print the first 5 natural numbers using a loop.
Expected Output:
1
2
3
4
5
Q 15. Write a Python program to print "Python" 3 times.
Expected Output:
Python
Python
Python
Q 16. Write a Python program to find the maximum of two numbers.
Q 17. Write a Python program to find the length of a string.
Input: text = "Hello"
Expected Output:
5
Q 18. Write a Python program to check if a string contains a specific letter.
Q 19. Write a Python program to concatenate two strings.
Q 20. Write a Python program to repeat a string 3 times.
Expected Output:
HiHiHi
Q 21. Write a Python program to find the smallest of three numbers.
Input: a = 7, b = 4, c = 9
Q 22. Write a Python program to print the first character of a string.
Input: text = "Python"
Q 23. Write a Python program to print the last character of a string.
Input: text = "Python"
Q 24. Write a Python program to check if a number is divisible by 5.
Q 25. Write a Python program to calculate the square root of a number.
Q 26. Write a Python program to print all even numbers from 1 to 10.
Expected Output:
2
4
6
8
10
Q 27. Write a Python program to print the sum of all numbers from 1 to 5.
Expected Output:
15
Q 28. Write a Python program to reverse a string.
Input: text = "Technovlogs"
Q 29. Write a Python program to convert a string to uppercase.
Input: text = "technovlogs"
Q 30. Write a Python program to convert a string to lowercase.
Input: text = "TECHNOVLOGS"
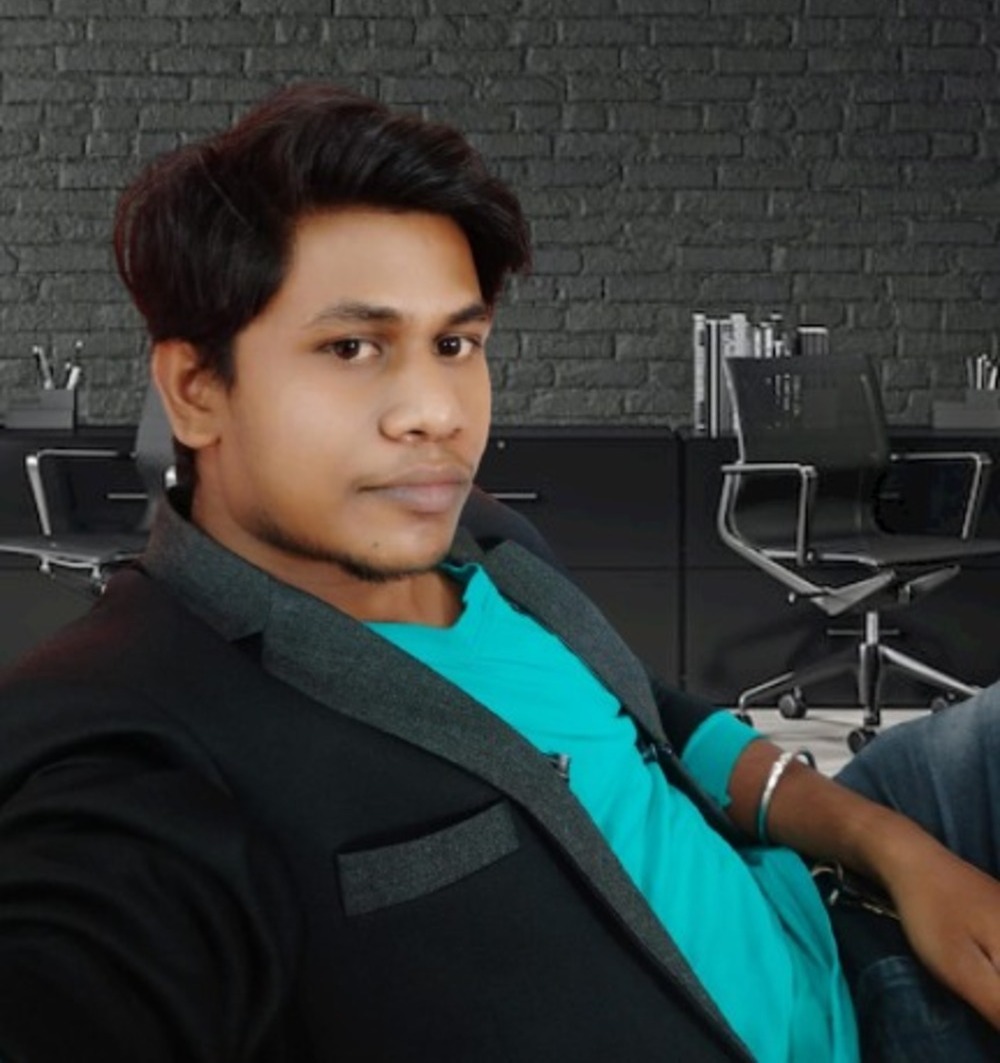
Bikki Singh
Hi, I am the instructor of TechnoVlogs. I have a strong love for programming and enjoy teaching through practical examples. I made this site to help people improve their coding skills by solving real-world problems. With years of experience, my goal is to make learning programming easy and fun for everyone. Let's learn and grow together!