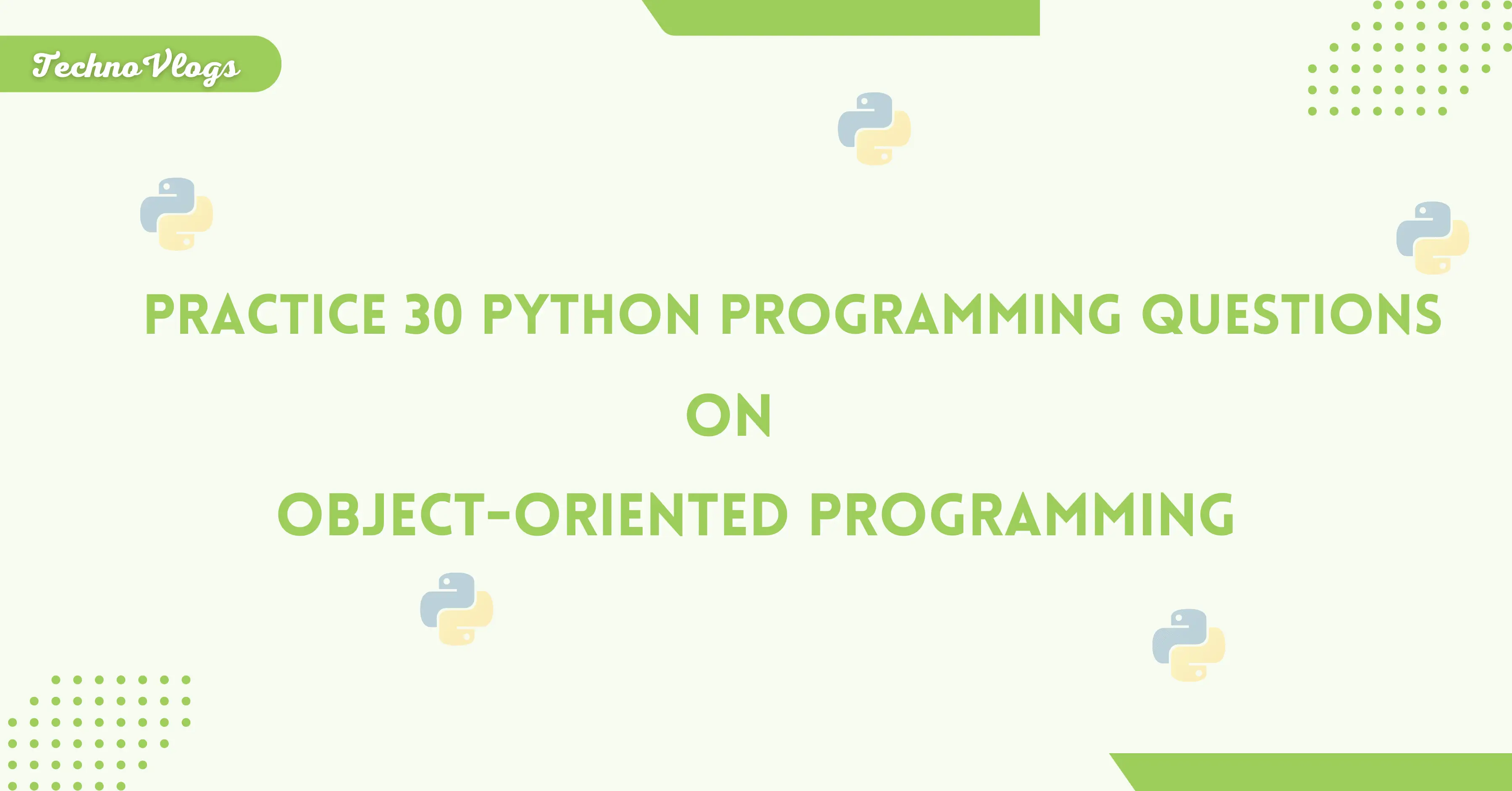
Practice Top 30 Python Programming Questions on Object Oriented Programming
Q1. Create a class Person with an attribute name. Instantiate it with the name "Alice" and display the name.
- Input: None
- Expected Output: "Alice"
Q2. Create a class Calculator with a method add(a, b) that returns the sum of two numbers. Use it to calculate 2 + 3.
- Input: None
- Expected Output: 5
Q3. Create a class Student with attributes name and age. Modify the age attribute after object creation.
- Input: name="John", age=20, then age=21
- Expected Output: 21
Q4. Create a class Car with an attribute brand that defaults to "Toyota". Create an object and display its brand.
- Input: None
- Expected Output: "Toyota"
Q5. Create a class Rectangle with methods area(length, width) and perimeter(length, width). Call both methods.
- Input: length=4, width=3
- Expected Output: Area: 12, Perimeter: 14
Q6. Create a class Circle with a constructor to initialize the radius and a method area() to calculate the area.
- Input: radius=5
- Expected Output: 78.5
Q7. Create a class Demo with a destructor that prints "Object destroyed" when an object is deleted.
- Input: Create and delete the object.
- Expected Output: "Object destroyed"
Q8. Create a class Employee with a constructor to initialize name and salary. Display both attributes.
- Input: name="Alice", salary=50000
- Expected Output: Name: Alice, Salary: 50000
Q9. Create a class Book with a default constructor that sets the title to "Unknown". Display the title.
- Input: None
- Expected Output: "Unknown"
Q10. Create a class Player with a parameterized constructor. If no name is passed, default to "Guest".
- Input: Name provided: "John", Name not provided: None
- Expected Output: "John", "Guest"
Q11. Create a class Animal with a method speak(). Inherit it in Dog and override the method to print "Bark!".
- Input: None
- Expected Output: "Bark!"
Q12. Use super() in the child class to call the speak() method of the parent Animal class.
- Input: None
- Expected Output: "Animal sound"
Q13. Create three classes A, B, and C where B inherits A and C inherits B. Call a method from A using an object of C.
- Input: None
- Expected Output: "Method from class A"
Q14. Create a base class Shape and derive Rectangle and Triangle. Add unique methods in both derived classes.
- Input: None
- Expected Output: Rectangle and Triangle methods executed.
Q15. Create a class Vehicle with a method speed(). Override it in Car to print "Car speed is 100 km/h".
- Input: None
- Expected Output: "Car speed is 100 km/h"
Q16. Write a program where the same function area() behaves differently in Circle and Rectangle.
- Input: Circle: radius=5, Rectangle: length=4, width=3
- Expected Output: Circle area: 78.5, Rectangle area: 12
Q17. Create a class Account with a private attribute _balance and a public method get_balance(). Access the balance through the method.
- Input: _balance=500
- Expected Output: 500
Q18. Write a function describe() that prints different messages for objects of Dog and Cat.
- Input: Object type: Dog, Cat
- Expected Output: "This is a Dog", "This is a Cat"
Q19. Write a program to demonstrate public, private, and protected attributes in a class.
- Input: None
- Expected Output: Access behavior for public, protected, and private attributes.
Q20. Create a class Person with attributes name and age. Use getter and setter methods to access and modify them.
- Input: name="Alice", age=25, then modify to age=26
- Expected Output: 26
Q21. Create a class Person and override the __str__ method to return "Person: [Name]".
- Input: name="Alice"
- Expected Output: "Person: Alice"
Q22. Override the __repr__ method in a class Book to display "Book(title)".
- Input: title="Python"
- Expected Output: "Book('Python')"
Q23. Create a class Number and override the __add__ method to add two objects.
- Input: Number(5) + Number(10)
- Expected Output: 15
Q24. Create a class Collection and define a __len__ method that returns the number of items.
- Input: Collection with 3 items
- Expected Output: 3
Q25. Create a class Point and override __eq__ to compare two points.
- Input: Point(2, 3) == Point(2, 3)
- Expected Output: True
Q26. Create a class Person with the attributes name and age. Instantiate the class with name="Tom" and age=30.
- Input: name="Tom", age=30
- Expected Output: "Tom, 30"
Q27. Create a class Greeting with a method greet(name="User") that prints "Hello, name". Call the method with and without an argument.
- Input: "Alice", None
- Expected Output: "Hello, Alice", "Hello, User"
Q28. Create a class Book with attributes title, author, and year. Instantiate the class with the values "Python Basics", "John", and 2021.
- Input: title="Python Basics", author="John", year=2021
- Expected Output: "Python Basics, John, 2021"
Q29. Create a class Math with a static method add(a, b) to add two numbers. Call the static method without creating an object.
- Input: add(3, 4)
- Expected Output: 7
Q30. Create a class Car with a class attribute wheels set to 4. Access the class attribute using the class name and object.
- Input: Car.wheels
- Expected Output: 4

Bikki Singh
Hi, I am the instructor of TechnoVlogs. I have a strong love for programming and enjoy teaching through practical examples. I made this site to help people improve their coding skills by solving real-world problems. With years of experience, my goal is to make learning programming easy and fun for everyone. Let's learn and grow together!