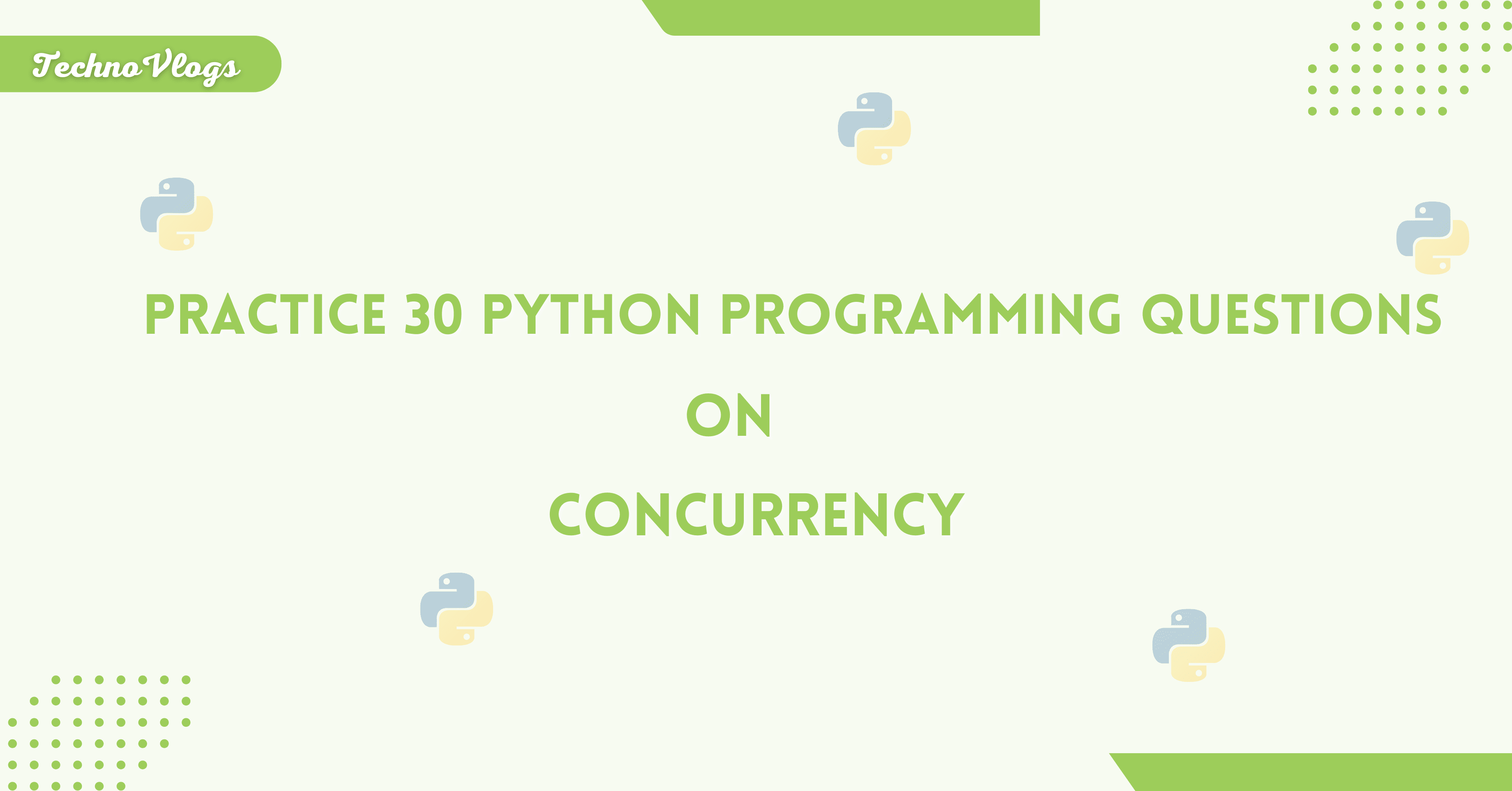
Practice Top 30 Python Programming Questions on Concurrency
Q1. Write a Python program to create a thread that prints "Hello from thread!".
Expected Output:
Hello from thread!
Q2. Write a Python program to create a thread that executes a function calculating the square of a given number 5.
Expected Output:
Square of 5: 25
Q3. Write a program to create two threads, one printing "Thread 1" and the other printing "Thread 2".
Expected Output:
Thread 1
Thread 2
Thread 1
Thread 2
Q4. Write a program to pass the name of a user ("Alice") to a thread function and greet them.
Expected Output:
Hello, Alice!
Q5. Write a program where two threads increment a shared counter. Use a lock to ensure thread-safe access.
Expected Output:
Final counter value: 200
Q6. Create a program where a background thread prints "Daemon running..." every second, while the main thread exits after 5 seconds.
Expected Output:
Daemon running...
Daemon running...
...
Main thread exits.
Q7. Write a Python program to use ThreadPoolExecutor to calculate squares of numbers [1, 2, 3, 4, 5].
Expected Output:
[1, 4, 9, 16, 25]
Q8. Write a program that uses the threading.Timer to print "Time's up!" after 5 seconds.
Expected Output:
Time's up!
Q9. Write a Python program where a thread runs an infinite loop but exits when the main program sets a flag.
Expected Output:
Thread exited.
Q10. Create a program where one thread adds items to a queue and another thread processes them.
Expected Output:
Processed: item1
Processed: item2
Q11. Write a Python program to create a process that prints "Hello from process!".
Expected Output:
Hello from process!
Q12. Write a program to create two processes: one that calculates squares and another that calculates cubes of numbers [1, 2, 3].
Expected Output:
Square: 1, Cube: 1
Square: 4, Cube: 8
Q13. Write a program to pass a name ("Bob") to a process and print a greeting.
Expected Output:
Hello, Bob!
Q14. Use a multiprocessing.Pool to calculate the factorial of numbers [3, 4, 5].
Expected Output:
[6, 24, 120]
Q15. Create a program where two processes increment a shared value using Value from multiprocessing.
Expected Output:
Final value: 200
Q16. Write a program where one process adds items to a queue, and another process consumes them.
Expected Output:
Consumed: item1
Consumed: item2
Q17. Write a program where multiple processes increment a shared counter with a lock for synchronization.
Expected Output:
Final counter value: 100
Q18. Write a program where a process runs a long computation, but the main process waits only 5 seconds.
Expected Output:
Process terminated due to timeout.
Q19. Write a program using ProcessPoolExecutor to compute the sum of numbers in each sublist of [[1, 2], [3, 4], [5, 6]].
Expected Output:
[3, 7, 11]
Q20. Write a program where multiple processes calculate the length of strings ["apple", "banana", "cherry"].
Expected Output:
[5, 6, 6]
Q21. Write an async function that prints "Async function running!".
Expected Output:
Async function running!
Q22. Write a program to call an async function that waits for 2 seconds before printing "Waited 2 seconds!".
Expected Output:
Waited 2 seconds!
Q23. Write a program to run two async tasks concurrently, one printing "Task 1" and the other "Task 2".
Expected Output:
Task 1
Task 2
Q24. Write a program to run multiple async functions using asyncio.gather. Each function should print a unique message.
Expected Output:
Function 1 running
Function 2 running
Function 3 running
Q25. Write a program where an async task runs for a long time, but the program exits after 5 seconds.
Expected Output:
Task timed out.
Q26. Use aiohttp to make an async HTTP GET request to https://jsonplaceholder.typicode.com/posts/1.
Expected Output:
json
{
"userId": 1,
"id": 1,
"title": "...",
"body": "..."
}
Q27. Create an async producer-consumer model using an asyncio.Queue.
Expected Output:
Produced: item1
Consumed: item1
Produced: item2
Consumed: item2
Q28. Write a program where an async function runs every 2 seconds and prints "Periodic task running...".
Expected Output:
Periodic task running...
Periodic task running...
Q29. Write an async function to read the contents of a text file using aiofiles.
Expected Output:
File content: Hello, asyncio!
Q30. Write a program where an async task runs indefinitely but gets canceled after 5 seconds.
Expected Output:
Task canceled.

Bikki Singh
Hi, I am the instructor of TechnoVlogs. I have a strong love for programming and enjoy teaching through practical examples. I made this site to help people improve their coding skills by solving real-world problems. With years of experience, my goal is to make learning programming easy and fun for everyone. Let's learn and grow together!