
30 Python Programming Questions on Recursive Functions
Q 1. Calculate the factorial of a number using recursion.
Input: 5
Expected Output:
120
Q 2. Calculate the nth Fibonacci number using recursion.
Input: 6
Expected Output:
8
Q 3. Compute the sum of digits of a number using recursion.
Input: 123
Expected Output:
6
Q 4. Reverse a string using recursion.
Input: hello
Expected Output:
olleh
Q 5. Check if a string is a palindrome using recursion.
Input: racecar, hello
Expected Output:
True
False
Q 6. Compute the power of a number using recursion.
Input: 2, 3
Expected Output:
8
Q 7. Find the greatest common divisor (GCD) using recursion.
Input: 48, 18
Expected Output:
6
Q 8. Compute the sum of a list of numbers using recursion.
Input: [1, 2, 3, 4, 5]
Expected Output:
15
Q 9. Compute the product of a list of numbers using recursion.
Input: [1, 2, 3, 4]
Expected Output:
24
Q 10. Find the length of a list using recursion.
Input: [1, 2, 3, 4, 5]
Expected Output:
5
Q 11. Flatten a nested list using recursion.
Input: 1, [2, [3, 4]], 5]
Expected Output:
[1, 2, 3, 4, 5]
Q 12. Compute the nth triangular number using recursion.
Input: 5
Expected Output:
15
Q 13. Find the minimum value in a list using recursion.
Input: [3, 5, 1, 9, 2]
Expected Output:
1
Q 14. Compute the sum of all even numbers in a list using recursion.
Input: [1, 2, 3, 4, 5, 6]
Expected Output:
12
Q 15. Compute the sum of all odd numbers in a list using recursion.
Input: [1, 2, 3, 4, 5, 6]
Expected Output:
9
Q 16. Find the nth power of a number using recursion.
Input: 5, 3
Expected Output:
125
Q 17. Find the index of a value in a list using recursion.
Input: [10, 20, 30, 40], 30
[10, 20, 30, 40], 50
Expected Output:
2
-1
Q 18. Count the number of occurrences of an element in a list using recursion.
Input: [1, 2, 2, 3, 2, 4], 2
Expected Output:
3
Q 19. Generate a list of numbers from 1 to n using recursion.
Input: 5
Expected Output:
[1, 2, 3, 4, 5]
Q 20. Compute the sum of all numbers from 1 to n using recursion.
Input: 4
Expected Output:
10
Q 21. Compute the nth number in the Tribonacci sequence using recursion.
Input: 4
Expected Output:
4
Q 22. Find the maximum value in a list using recursion.
Input: [3, 5, 1, 9, 2]
Expected Output:
9
Q 23. Print all permutations of a string using recursion.
Input: abc
Expected Output:
['abc', 'acb', 'bac', 'bca', 'cab', 'cba']
Q 24. Print all subsets of a set using recursion.
Input: [1, 2, 3]
Expected Output:
[[], [1], [2], [1, 2], [3], [1, 3], [2, 3], [1, 2, 3]]
Q 25. Compute the sum of a geometric series using recursion.
Input: 2, 3, 4
Expected Output:
80
Q 26. Print the sequence of natural numbers up to n using recursion.
Input: 5
Expected Output:
1
2
3
4
5
Q 27. Print the sequence of natural numbers in reverse up to n using recursion.
Input: 5
Expected Output:
5
4
3
2
1
Q 28. Find the sum of elements in a nested list using recursion.
Input: [1, [2, [3, 4]], 5]
Expected Output:
15
Q 29. Find the number of elements in a nested list using recursion.
Input: [1, [2, [3, 4]], 5]
Expected Output:
5
Q 30. Find the maximum depth of nested lists using recursion.
Input: [1, [2, [3, 4]], 5])
Expected Output:
3
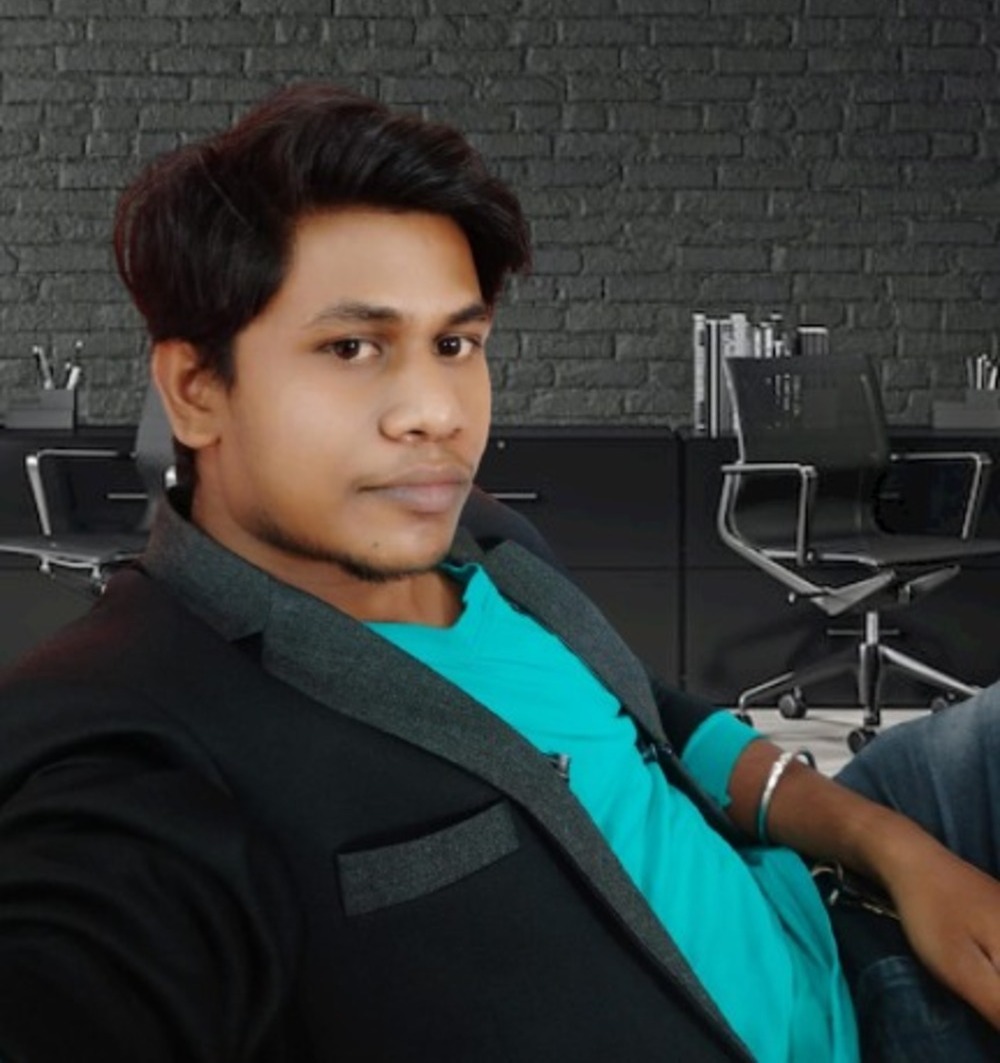
Bikki Singh
Hi, I am the instructor of TechnoVlogs. I have a strong love for programming and enjoy teaching through practical examples. I made this site to help people improve their coding skills by solving real-world problems. With years of experience, my goal is to make learning programming easy and fun for everyone. Let's learn and grow together!