
30 Python Programming Questions on Returning values from Functions
Q 1. Define a function that returns a fixed value and call it.
Expected Output:
5
Q 2. Define a function that returns the sum of two numbers.
Expected Output:
7
Q 3. Define a function that returns the maximum of two numbers.
Expected Output:
20
Q 4. Define a function that returns the length of a string.
Input: Python
Expected Output
6
Q 5. Define a function that returns the average of a list of numbers.
Input: [1, 2, 3, 4, 5]
Expected Output:
3.0
Q 6. Define a function that returns the result of a multiplication of two numbers.
Q 7. Define a function that returns whether a number is even or odd.
Q 8. Define a function that returns the first element of a list.
List: [1, 2, 3, 4]
Expected Output:
1
Q 9. Define a function that returns a string in uppercase.
Expected Output:
HELLO
Q 10. Define a function that returns a concatenated string of two inputs.
Expected Output:
Hello World
Q 11. Define a function that returns the factorial of a number.
Q 12. Define a function that returns the last element of a list.
List: [1, 2, 3, 4]
Expected Output:
4
Q 13. Define a function that returns whether a string contains a specific substring.
Q 14. Define a function that returns the square of a number.
Q 15. Define a function that returns the length of the longest string in a list.
Q 16. Define a function that returns the average of numbers in a list but excludes the lowest number.
Q 17. Define a function that returns a reversed version of a string.
Q 18. Define a function that returns a list of all even numbers from a given list.
Q 19. Define a function that returns a dictionary with the count of each character in a string.
Q 20. Define a function that returns whether a number is prime.
Q 21. Define a function that returns a list of the first n Fibonacci numbers.
Q 22. Define a function that returns a list of squares of numbers from 1 to n.
Q 23. Define a function that returns the number of vowels in a string.
Q 24. Define a function that returns the reversed list of numbers.
Q 25. Define a function that returns a list of the factorials of numbers from 1 to n.
Q 26. Define a function that returns a dictionary where keys are numbers and values are their squares.
Q 27. Define a function that returns a boolean indicating if a string is a palindrome.
Q 28. Define a function that returns the smallest number from a list.
Q 29. Define a function that returns a list of all unique elements from a list.
Q 30. Define a function that returns a tuple with the count of even and odd numbers from a list.
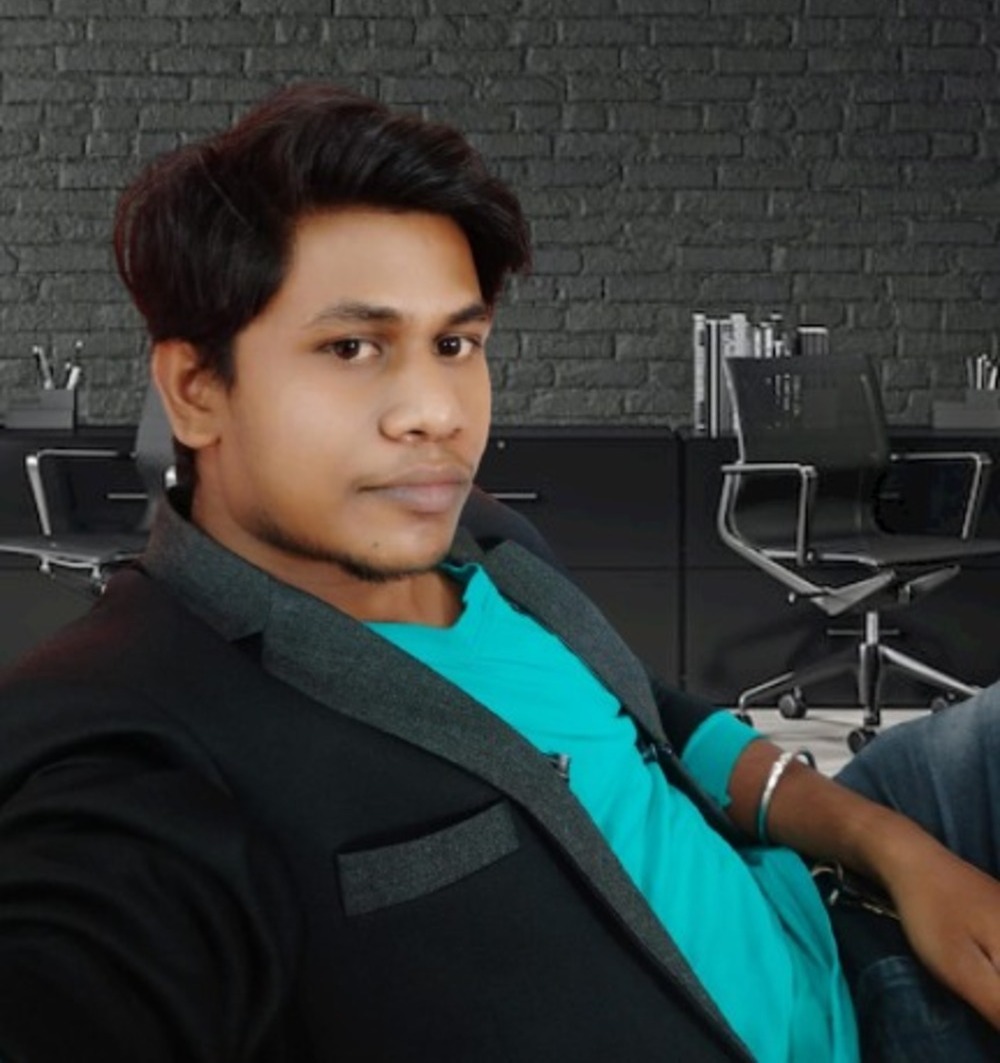
Bikki Singh
Hi, I am the instructor of TechnoVlogs. I have a strong love for programming and enjoy teaching through practical examples. I made this site to help people improve their coding skills by solving real-world problems. With years of experience, my goal is to make learning programming easy and fun for everyone. Let's learn and grow together!