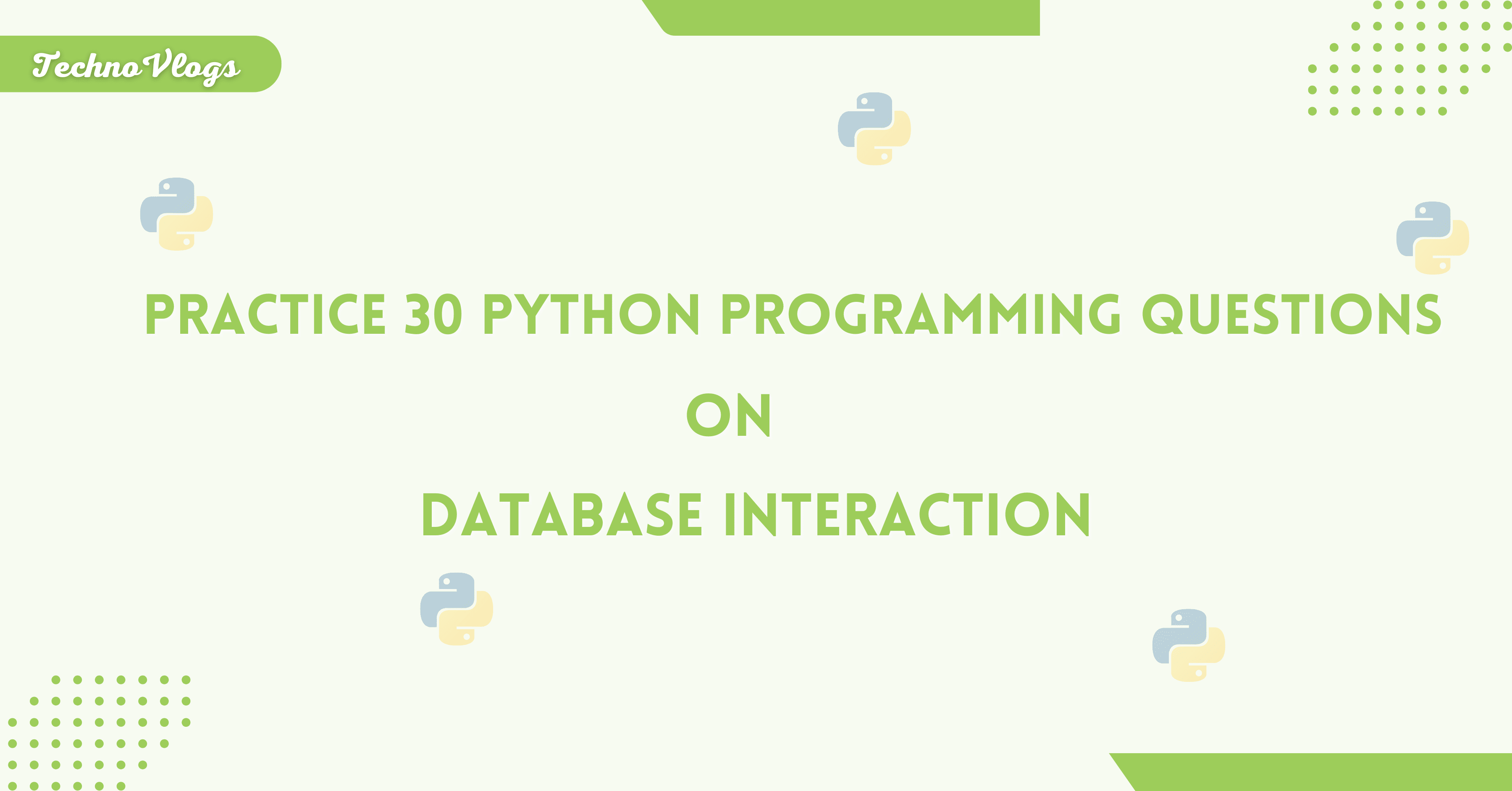
Practice Top 30 Python Programming Questions on Database Interaction
Q1. Write a Python program to connect to an SQLite database named example.db. Create a connection and print "Connected to SQLite database successfully!".
Expected Output:
Connected to SQLite database successfully!
Q2. Write a program to create a table users with columns id (integer primary key) and name (text) in example.db.
Expected Output:
Table 'users' created successfully.
Q3. Write a Python program to check whether the connection to an SQLite database is active and print "Connection is active!".
Expected Output:
Connection is active!
Q4. Write a Python program to connect to a MySQL database named testdb using MySQLdb. Print "Connected to MySQL database successfully!".
Expected Output:
Connected to MySQL database successfully!
Q5. Write a Python program to retrieve the schema of a table named products from an SQLite database and display the column names.
Expected Output:
Columns: id, name, price
Q6. Write a program to insert a user with name='Alice' into the users table in example.db.
Expected Output:
Row inserted successfully.
Q7. Write a program to fetch and display all rows from the users table.
Expected Output:
ID: 1, Name: Alice
Q8. Write a program to update the name of the user with id=1 to "Bob".
Expected Output:
Row updated successfully.
Q9. Write a program to delete the user with id=1 from the users table.
Expected Output:
Row deleted successfully.
Q10. Write a program to insert multiple users into the users table: ("Charlie") and ("David").
Expected Output:
2 rows inserted successfully.
Q11. Write a program to insert a user into the users table using a parameterized query with a variable user_name = "Eve".
Expected Output:
User 'Eve' inserted successfully.
Q12. Write a program to insert two rows into the users table within a transaction, and commit the changes.
Expected Output:
Transaction completed successfully.
Q13. Write a program to fetch all users whose names start with 'C'.
Expected Output:
ID: 3, Name: Charlie
Q14. Write a program to fetch rows where the name contains the letter 'a'.
Expected Output:
ID: 2, Name: Charlie
ID: 3, Name: David
Q15. Write a program to count the total number of rows in the users table.
Expected Output:
Total users: 2
Q16. Write a program to join two tables, users and orders, to fetch user names along with their order details.
Expected Output:
Name: Charlie, Order: Laptop
Name: David, Order: Phone
Q17. Write a program to fetch all users in alphabetical order.
Expected Output:
ID: 3, Name: Charlie
ID: 4, Name: David
Q18. Write a program to handle exceptions while inserting data into a table that doesn’t exist. Print "Table not found!".
Expected Output:
Table not found!
Q19. Write a program to drop the users table.
Expected Output:
Table 'users' dropped successfully.
Q20. Write a program to back up an SQLite database to backup.db.
Expected Output:
Database backed up successfully to backup.db.
Q21. Define a model for a table products with columns id, name, and price.
Expected Output:
Model 'Product' defined successfully.
Q22. Write a Python program to create the products table using SQLAlchemy.
Expected Output:
Table 'products' created successfully.
Q23. Write a program to insert a product ("Laptop", 1200) into the products table using SQLAlchemy.
Expected Output:
Product added successfully.
Q24. Write a program to fetch and display all products using SQLAlchemy.
Expected Output:
ID: 1, Name: Laptop, Price: 1200
Q25. Write a program to update the price of the product with id=1 to 1500 using SQLAlchemy.
Expected Output:
Product updated successfully.
Q26. Write a program to delete a product with id=1 from the products table using SQLAlchemy.
Expected Output:
Product deleted successfully.
Q27. Write a program to fetch all products with a price greater than 1000.
Expected Output:
ID: 1, Name: Laptop, Price: 1200
Q28. Define a relationship between users and orders tables using SQLAlchemy ORM and fetch user names along with their orders.
Expected Output:
Name: Alice, Order: Book
Q29. Write a program to add two products to the products table within a single transaction.
Expected Output:
Transaction completed successfully.
Q30. Write a program to demonstrate how to use SQLAlchemy’s session management for querying and committing data.
Expected Output:
Session completed successfully.

Bikki Singh
Hi, I am the instructor of TechnoVlogs. I have a strong love for programming and enjoy teaching through practical examples. I made this site to help people improve their coding skills by solving real-world problems. With years of experience, my goal is to make learning programming easy and fun for everyone. Let's learn and grow together!