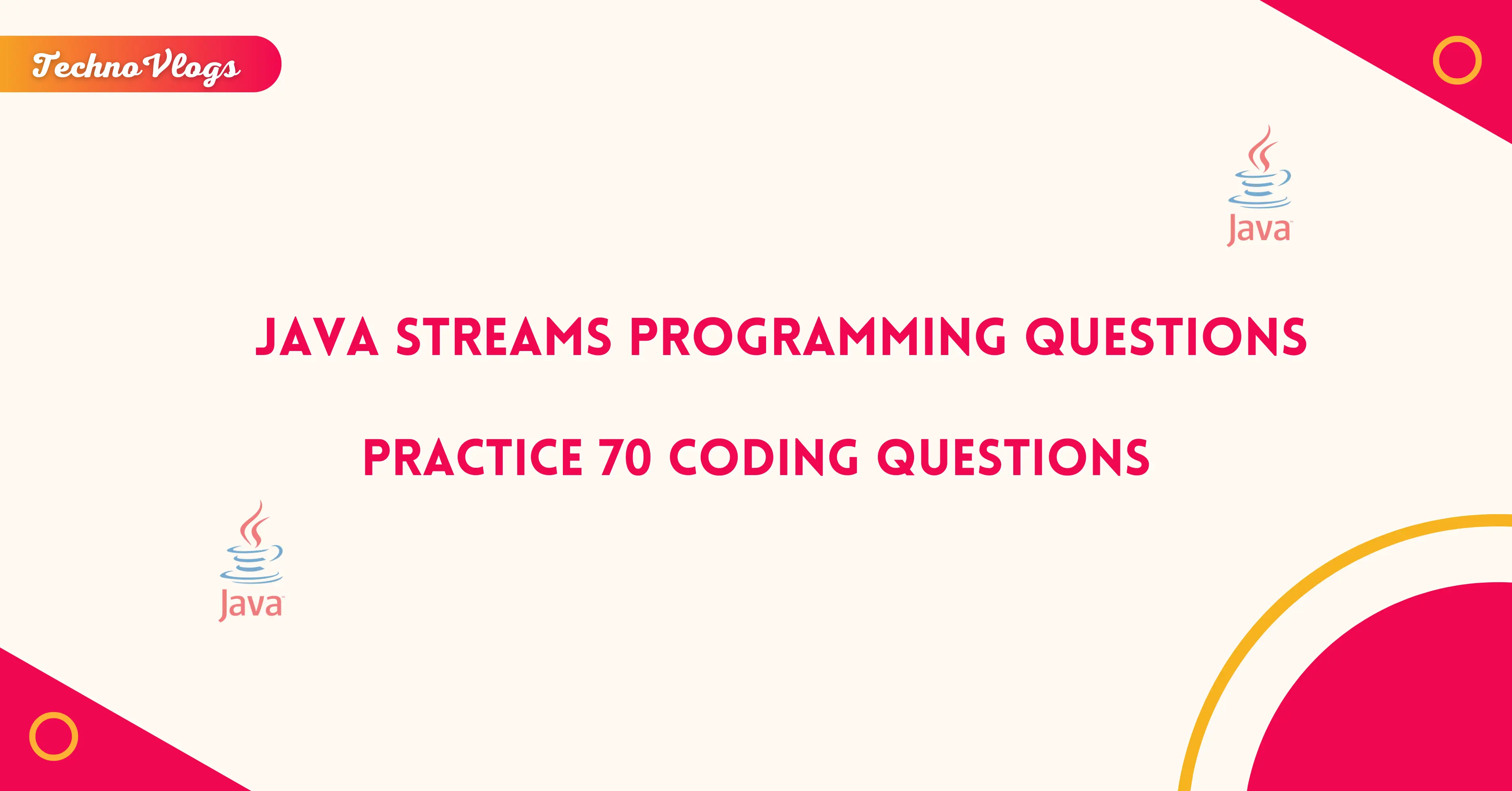
Practice 70 Java Streams Programming Questions
Q1. Write a Java program using streams to calculate the sum of a list of integers.
Input:
List = [1, 2, 3, 4, 5]
Expected Output:
Sum = 15
Q2. Write a Java program using streams to find the maximum number from a list of integers.
Input:
List = [10, 20, 30, 40, 50]
Expected Output:
Max = 50
Q3. Write a Java program using streams to filter out all even numbers from a list.
Input:
List = [1, 2, 3, 4, 5, 6, 7]
Expected Output:
[1, 3, 5, 7]
Q4. Write a Java program using streams to find the average of a list of integers.
Input:
List = [10, 20, 30, 40]
Expected Output:
Average = 25.0
Q5. Write a Java program using streams to check if all elements in a list are positive.
Input:
List = [1, 2, 3, 4]
Expected Output:
true
Q6. Write a Java program using streams to check if any element in a list is greater than 10.
Input:
List = [1, 2, 5, 8]
Expected Output:
false
Q7. Write a Java program using streams to sort a list of integers in ascending order.
Input:
List = [5, 3, 7, 2]
Expected Output:
[2, 3, 5, 7]
Q8. Write a Java program using streams to find the first element in a list of integers greater than 5.
Input:
List = [1, 2, 3, 4, 6, 7]
Expected Output:
6
Q9. Write a Java program using streams to remove duplicates from a list of integers.
Input:
List = [1, 2, 3, 1, 4, 5, 2]
Expected Output:
[1, 2, 3, 4, 5]
Q10. Write a Java program using streams to convert a list of strings to uppercase.
Input:
List = ["java", "streams", "example"]
Expected Output:
[JAVA, STREAMS, EXAMPLE]
Q11. Write a Java program using streams to count the number of even numbers in a list.
Input:
List = [1, 2, 3, 4, 5]
Expected Output:
2
Q12. Write a Java program using streams to concatenate all strings in a list into one string.
Input:
List = ["Java", "Streams", "Example"]
Expected Output:
JavaStreamsExample
Q13. Write a Java program using streams to find the longest string in a list.
Input:
List = ["Java", "Streams", "Example"]
Expected Output:
Streams
Q14. Write a Java program using streams to map a list of integers to their square values.
Input:
List = [1, 2, 3, 4]
Expected Output:
[1, 4, 9, 16]
Q15. Write a Java program using streams to get all strings from a list that start with the letter 'J'.
Input:
List = ["Java", "Streams", "Jungle", "Python"]
Expected Output:
[Java, Jungle]
Q16. Write a Java program using streams to find the product of all integers in a list.
Input:
List = [2, 3, 4]
Expected Output:
24
Q17. Write a Java program using streams to get the first 3 elements from a list.
Input:
List = [1, 2, 3, 4, 5]
Expected Output:
[1, 2, 3]
Q18. Write a Java program using streams to find the sum of even numbers in a list.
Input:
List = [1, 2, 3, 4, 5]
Expected Output:
6
Q19. Write a Java program using streams to get the distinct characters from a string.
Input:
str = "java"
Expected Output:
[j, a, v]
Q20. Write a Java program using streams to sort a list of strings in descending order.
Input:
List = ["apple", "banana", "cherry"]
Expected Output:
[cherry, banana, apple]
Q21. Write a Java program using streams to check if any element in a list is a palindrome.
Input:
List = ["madam", "hello", "level"]
Expected Output:
true
Q22. Write a Java program using streams to find the sum of all odd numbers in a list.
Input:
List = [1, 2, 3, 4, 5]
Expected Output:
9
Q23. Write a Java program using streams to find the maximum length string in a list.
Input:
List = ["java", "streams", "example"]
Expected Output:
streams
Q24. Write a Java program using streams to get the square of each number in a list greater than 5.
Input:
List = [2, 6, 7, 3]
Expected Output:
[36, 49]
Q25. Write a Java program using streams to sort a list of integers in descending order.
Input:
List = [10, 5, 20, 15]
Expected Output:
[20, 15, 10, 5]
Q26. Write a Java program using streams to check if all strings in a list have a length greater than 3.
Input:
List = ["java", "streams", "example"]
Expected Output:
true
Q27. Write a Java program using streams to find the sum of squares of all numbers in a list.
Input:
List = [1, 2, 3, 4]
Expected Output:
30
Q28. Write a Java program using streams to filter out all strings from a list that have length greater than 5.
Input:
List = ["java", "streams", "example", "python"]
Expected Output:
[python]
Q29. Write a Java program using streams to create a new list where each number in the original list is doubled.
Input:
List = [1, 2, 3, 4]
Expected Output:
[2, 4, 6, 8]
Q30. Write a Java program using streams to find the first 2 even numbers in a list.
Input:
List = [1, 2, 3, 4, 5, 6]
Expected Output:
[2, 4]
Q31. Write a Java program using streams to return the first string that contains the letter 'e' from a list.
Input:
List = ["java", "streams", "example", "hello"]
Expected Output:
java
Q32. Write a Java program using streams to filter a list of strings that contains the substring "Java".
Input:
List = ["Java", "JavaScript", "Python", "JavaBeans"]
Expected Output:
[Java, JavaScript, JavaBeans]
Q33. Write a Java program using streams to find the count of strings in a list that contain the letter 'a'.
Input:
List = ["apple", "banana", "cherry", "date"]
Expected Output:
3
Q34. Write a Java program using streams to get the sum of the first 3 elements in a list.
Input:
List = [1, 2, 3, 4, 5]
Expected Output:
6
Q35. Write a Java program using streams to find the largest number less than 50 in a list.
Input:
List = [10, 20, 30, 40, 50, 60]
Expected Output:
40
Q36. Write a Java program using streams to find if there is any string that starts with 'J'.
Input:
List = ["Java", "python", "JavaScript", "C++"]
Expected Output:
true
Q37. Write a Java program using streams to convert a list of integers into a list of their binary representations.
Input:
List = [2, 3, 4]
Expected Output:
[10, 11, 100]
Q38. Write a Java program using streams to remove all elements that are greater than 10 from a list.
Input:
List = [5, 12, 3, 8, 15]
Expected Output:
[5, 3, 8]
Q39. Write a Java program using streams to check if there is any number divisible by both 3 and 5 in a list.
Input:
List = [2, 3, 5, 15, 7]
Expected Output:
true
Q40. Write a Java program using streams to create a list of the squares of all numbers less than 5.
Input:
List = [1, 2, 3, 4, 5]
Expected Output:
[1, 4, 9, 16]
Q41. Write a Java program using streams to reverse the order of elements in a list.
Input:
List = [1, 2, 3, 4, 5]
Expected Output:
[5, 4, 3, 2, 1]
Q42. Write a Java program using streams to check if all numbers in a list are even.
Input:
List = [2, 4, 6, 8]
Expected Output:
true
Q43. Write a Java program using streams to find the sum of all numbers divisible by 3 in a list.
Input:
List = [1, 2, 3, 4, 5, 6]
Expected Output:
9
Q44. Write a Java program using streams to find the second largest number in a list.
Input:
List = [2, 3, 5, 7, 6]
Expected Output:
6
Q45. Write a Java program using streams to filter and get all strings that end with the letter 'e'.
Input:
List = ["apple", "banana", "cherry", "orange"]
Expected Output:
[apple, orange]
Q46. Write a Java program using streams to find the average of odd numbers in a list.
Input:
List = [1, 2, 3, 4, 5]
Expected Output:
3.0
Q47. Write a Java program using streams to flatten a list of lists into a single list.
Input:
List = [[1, 2], [3, 4], [5, 6]]
Expected Output:
[1, 2, 3, 4, 5, 6]
Q48. Write a Java program using streams to find the sum of all odd numbers less than 20 in a list.
Input:
List = [5, 10, 15, 20]
Expected Output:
20
Q49. Write a Java program using streams to get the smallest number greater than 10 from a list.
Input:
List = [3, 8, 12, 5]
Expected Output:
12
Q50. Write a Java program using streams to remove all null values from a list.
Input:
List = [1, null, 2, null, 3]
Expected Output:
[1, 2, 3]
Q51. Write a Java program using streams to group a list of integers based on whether they are even or odd.
Input:
List = [1, 2, 3, 4, 5]
Expected Output:
{odd=[1, 3, 5], even=[2, 4]}
Q52. Write a Java program using streams to convert a list of strings into a list of their lengths.
Input:
List = ["java", "streams", "example"]
Expected Output:
[4, 7, 7]
Q53. Write a Java program using streams to find if there is any number greater than 100 in a list.
Input:
List = [50, 80, 120, 30]
Expected Output:
true
Q54. Write a Java program using streams to partition a list of integers into odd and even numbers.
Input:
List = [1, 2, 3, 4, 5]
Expected Output:
{false=[2, 4], true=[1, 3, 5]}
Q55. Write a Java program using streams to find the average of all even numbers greater than 10 in a list.
Input:
List = [5, 12, 8, 20]
Expected Output:
16.0
Q56. Write a Java program using streams to check if all strings in a list contain the letter 'a'.
Input:
List = ["apple", "banana", "cherry"]
Expected Output:
false
Q57. Write a Java program using streams to find the first string that contains more than 5 characters.
Input:
List = ["java", "streams", "example"]
Expected Output:
streams
Q58. Write a Java program using streams to find the sum of the lengths of all strings in a list.
Input:
List = ["java", "streams", "example"]
Expected Output:
16
Q59. Write a Java program using streams to filter out all strings that start with 'j' from a list.
Input:
List = ["java", "javaScript", "python"]
Expected Output:
[python]
Q60. Write a Java program using streams to check if a list of integers contains any number divisible by both 3 and 5.
Input:
List = [10, 15, 30]
Expected Output:
true
Q61. Write a Java program using streams to find the first string with more than 3 characters.
Input:
List = ["a", "bb", "abc", "def"]
Expected Output:
abc
Q62. Write a Java program using streams to filter and return only positive numbers from a list.
Input:
List = [-1, 2, -3, 4, 5]
Expected Output:
[2, 4, 5]
Q63. Write a Java program using streams to find the count of words that contain the letter 'e' in a list.
Input:
List = ["apple", "banana", "cherry", "date"]
Expected Output:
3
Q64. Write a Java program using streams to get the distinct characters from a string in a list.
Input:
str = "apple"
Expected Output:
[a, p, l, e]
Q65. Write a Java program using streams to create a new list of strings with all elements in uppercase.
Input:
List = ["java", "streams", "example"]
Expected Output:
[JAVA, STREAMS, EXAMPLE]
Q66. Write a Java program using streams to find the sum of the square roots of all elements in a list.
Input:
List = [4, 9, 16]
Expected Output:
9.0
Q67. Write a Java program using streams to find the product of all odd numbers in a list.
Input:
List = [1, 3, 5]
Expected Output:
15
Q68. Write a Java program using streams to find the index of the first element greater than 10 in a list.
Input:
List = [1, 5, 8, 12, 3]
Expected Output:
3
Q69. Write a Java program using streams to get a list of numbers in a range of 1 to 10 (inclusive).
Input:
No input
Expected Output:
[1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
Q70. Write a Java program using streams to check if any number in a list is a prime number.
Input:
List = [1, 4, 5, 6]
Expected Output:
true

Bikki Singh
Hi, I am the instructor of TechnoVlogs. I have a strong love for programming and enjoy teaching through practical examples. I made this site to help people improve their coding skills by solving real-world problems. With years of experience, my goal is to make learning programming easy and fun for everyone. Let's learn and grow together!