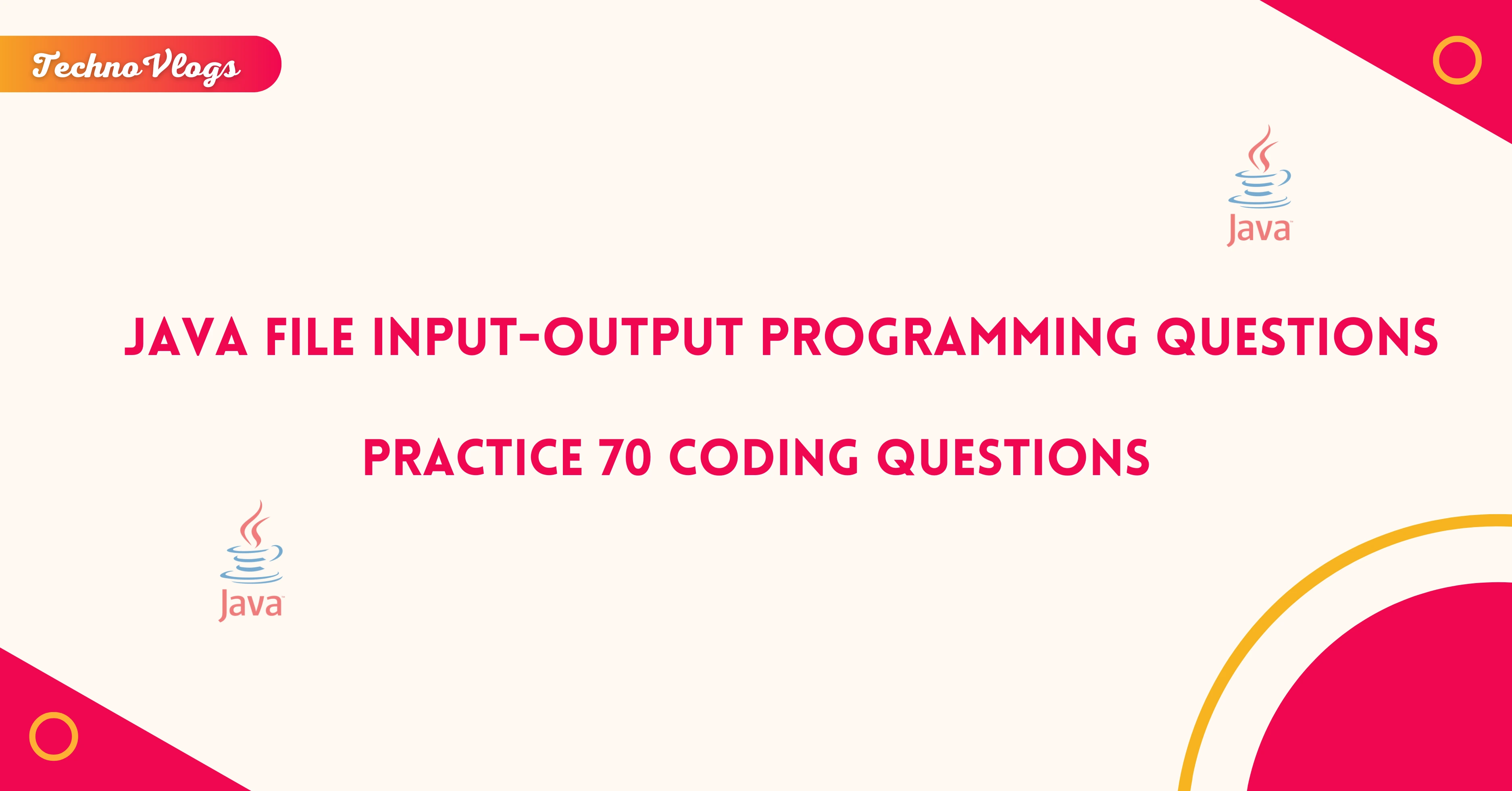
Practice 70 Java File Input-Output Programming Questions
Q1. Write a Java program to create a new file.
- Input: File name: "testfile.txt"
- Expected Output: "testfile.txt" created.
Q2. Write a Java program to write some text to a file.
- Input: "Hello, Java!"
- Expected Output: "Hello, Java!" written to "file.txt"
Q3. Write a Java program to read the contents of a file.
- Input: File: "testfile.txt"
- Expected Output: Content: "Hello, Java!"
Q4. Write a Java program to check if a file exists.
- Input: File: "testfile.txt"
- Expected Output: True
Q5. Write a Java program to delete a file.
- Input: File: "testfile.txt"
- Expected Output: "testfile.txt" deleted
Q6. Write a Java program to rename a file.
- Input: Old name: "testfile.txt", New name: "renamedfile.txt"
- Expected Output: File renamed to "renamedfile.txt"
Q7. Write a Java program to append text to an existing file.
- Input: Text to append: "Welcome to Java"
- Expected Output: "Welcome to Java" appended to "testfile.txt"
Q8. Write a Java program to copy a file from one location to another.
- Input: Source: "sourcefile.txt", Destination: "destinationfile.txt"
- Expected Output: "sourcefile.txt" copied to "destinationfile.txt"
Q9. Write a Java program to read a file line by line.
- Input: File: "testfile.txt"
- Expected Output: Prints each line of "testfile.txt"
Q10. Write a Java program to write an array of strings to a file.
- Input: ["Hello", "Java", "Programming"]
- Expected Output: Each string written to "file.txt" on a new line.
Q11. Write a Java program to create a directory.
- Input: Directory name: "testdirectory"
- Expected Output: Directory "testdirectory" created.
Q12. Write a Java program to list all files in a directory.
- Input: Directory: "testdirectory"
- Expected Output: List of files in "testdirectory"
Q13. Write a Java program to check if a file is readable.
- Input: File: "testfile.txt"
- Expected Output: True
Q14. Write a Java program to check if a file is writable.
- Input: File: "testfile.txt"
- Expected Output: True
Q15. Write a Java program to get the size of a file.
- Input: File: "testfile.txt"
- Expected Output: Size of "testfile.txt" in bytes
Q16. Write a Java program to read the first 5 characters from a file.
- Input: File: "testfile.txt"
- Expected Output: First 5 characters of "testfile.txt"
Q17. Write a Java program to write data to a file using FileWriter.
- Input: Text: "Java is fun!"
- Expected Output: "Java is fun!" written to "testfile.txt"
Q18. Write a Java program to read data from a file using FileReader.
- Input: File: "testfile.txt"
- Expected Output: Content of "testfile.txt"
Q19. Write a Java program to check the length of a file.
- Input: File: "testfile.txt"
- Expected Output: Length of "testfile.txt" in bytes
Q20. Write a Java program to write and then read from a file.
- Input: Text: "Java programming"
- Expected Output: Write "Java programming" to the file and read it back.
Q21. Write a Java program to write a list of strings to a file.
- Input: List: ["Hello", "World"]
- Expected Output: Each string written to a new line in "outputfile.txt"
Q22. Write a Java program to read content from a file and print the number of lines.
- Input: File: "testfile.txt"
- Expected Output: Number of lines in "testfile.txt"
Q23. Write a Java program to read and print all the characters from a file.
- Input: File: "testfile.txt"
- Expected Output: Print all characters from "testfile.txt"
Q24. Write a Java program to create a file and check if it is created successfully.
- Input: File name: "newfile.txt"
- Expected Output: File "newfile.txt" created successfully
Q25. Write a Java program to find the absolute path of a file.
- Input: File: "testfile.txt"
- Expected Output: Absolute path of "testfile.txt"
Q26. Write a Java program to create a file and write multiple lines to it.
- Input: Lines: ["Hello", "Java", "Programming"]
- Expected Output: Write each line to "newfile.txt"
Q27. Write a Java program to read the contents of a file and print each word.
- Input: File: "testfile.txt"
- Expected Output: Print each word from "testfile.txt"
Q28. Write a Java program to read a file and store its content into an array of strings.
- Input: File: "testfile.txt"
- Expected Output: Array containing each line of "testfile.txt"
Q29. Write a Java program to write numbers 1-10 to a file.
- Input: Numbers 1, 2, 3, ..., 10
- Expected Output: Numbers 1-10 written to "numbers.txt"
Q30. Write a Java program to read a file using BufferedReader.
- Input: File: "testfile.txt"
- Expected Output: Read content of "testfile.txt" using BufferedReader
Q31. Write a Java program to write a string to a file using BufferedWriter.
- Input: String: "Buffered writing example"
- Expected Output: Write the string to "bufferedfile.txt"
Q32. Write a Java program to check if a directory exists.
- Input: Directory: "testdirectory"
- Expected Output: True
Q33. Write a Java program to list all directories in a parent directory.
- Input: Parent Directory: "parentdirectory"
- Expected Output: List of subdirectories in "parentdirectory"
Q34. Write a Java program to copy contents of one directory to another directory.
- Input: Source directory: "dir1", Destination directory: "dir2"
- Expected Output: Copy all files from "dir1" to "dir2"
Q35. Write a Java program to find the last modified time of a file.
- Input: File: "testfile.txt"
- Expected Output: Last modified time of "testfile.txt"
Q36. Write a Java program to find the file extension of a file.
- Input: File: "testfile.txt"
- Expected Output: ".txt"
Q37. Write a Java program to create a new file if it doesn't exist.
- Input: File: "newfile.txt"
- Expected Output: "newfile.txt" created if not already present
Q38. Write a Java program to get the parent directory of a file.
- Input: File: "testfile.txt"
- Expected Output: Parent directory of "testfile.txt"
Q39. Write a Java program to check if a directory is empty.
- Input: Directory: "testdirectory"
- Expected Output: True or False depending on contents
Q40. Write a Java program to get the name of a file.
- Input: File: "testfile.txt"
- Expected Output: "testfile.txt"
Q41. Write a Java program to write a number to a file.
- Input: Number: 123
- Expected Output: Number 123 written to "numberfile.txt"
Q42. Write a Java program to check if a file is hidden.
- Input: File: "testfile.txt"
- Expected Output: True or False based on file properties
Q43. Write a Java program to read a file and print its contents in reverse order.
- Input: File: "testfile.txt"
- Expected Output: Print content of "testfile.txt" in reverse
Q44. Write a Java program to create a file and write user input to it.
- Input: User Input: "Hello User!"
- Expected Output: "Hello User!" written to "userinputfile.txt"
Q45. Write a Java program to append text to an existing file if it exists.
- Input: Text to append: "New line added"
- Expected Output: Text appended to "existingfile.txt"
Q46. Write a Java program to get the absolute path of a directory.
- Input: Directory: "testdirectory"
- Expected Output: Absolute path of "testdirectory"
Q47. Write a Java program to check if a file is a directory.
- Input: File: "testfile.txt"
- Expected Output: False
Q48. Write a Java program to read a file and count the number of characters.
- Input: File: "testfile.txt"
- Expected Output: Number of characters in "testfile.txt"
Q49. Write a Java program to read a file and count the number of words.
- Input: File: "testfile.txt"
- Expected Output: Number of words in "testfile.txt"
Q50. Write a Java program to check if a file can be executed.
- Input: File: "testfile.txt"
- Expected Output: True or False depending on file properties
Q51. Write a Java program to create a file and write some data from an array.
- Input: Array: ["Hello", "World"]
- Expected Output: Data written from the array to "outputfile.txt"
Q52. Write a Java program to read a file and print each line with line numbers.
- Input: File: "testfile.txt"
- Expected Output: Line 1: "Hello", Line 2: "Java"
Q53. Write a Java program to list files in a directory that match a pattern.
- Input: Directory: "testdirectory", Pattern: "*.txt"
- Expected Output: List of files in "testdirectory" with ".txt" extension
Q54. Write a Java program to create a file and check if the file can be deleted.
- Input: File: "deletablefile.txt"
- Expected Output: File "deletablefile.txt" can be deleted
Q55. Write a Java program to read and print the last line of a file.
- Input: File: "testfile.txt"
- Expected Output: Last line of "testfile.txt"
Q56. Write a Java program to list the contents of a directory in a sorted order.
- Input: Directory: "testdirectory"
- Expected Output: Sorted list of files in "testdirectory"
Q57. Write a Java program to check if a file is a symbolic link.
- Input: File: "testfile.txt"
- Expected Output: True or False depending on file type
Q58. Write a Java program to check if a file is a regular file.
- Input: File: "testfile.txt"
- Expected Output: True or False
Q59. Write a Java program to find the number of directories in a given path.
- Input: Directory: "testdirectory"
- Expected Output: Number of directories in "testdirectory"
Q60. Write a Java program to create a file and then delete it after some time.
- Input: File: "testfile.txt"
- Expected Output: File "testfile.txt" created and deleted after a delay
Q61. Write a Java program to read all lines from a file using Files class.
- Input: File: "testfile.txt"
- Expected Output: All lines read from "testfile.txt"
Q62. Write a Java program to write data to a file using PrintWriter.
- Input: Text: "Hello World"
- Expected Output: "Hello World" written to "printfile.txt"
Q63. Write a Java program to create a file if it does not exist and write to it.
- Input: File: "newfile.txt", Text: "Welcome"
- Expected Output: "Welcome" written to "newfile.txt"
Q64. Write a Java program to check the last modified time of a file.
- Input: File: "testfile.txt"
- Expected Output: Last modified time of "testfile.txt"
Q65. Write a Java program to read and print the contents of a file in chunks.
- Input: File: "testfile.txt"
- Expected Output: Print content of "testfile.txt" in chunks
Q66. Write a Java program to read a file and store its contents into a string.
- Input: File: "testfile.txt"
- Expected Output: Store the content of "testfile.txt" into a string
Q67. Write a Java program to write data from a byte array to a file.
- Input: Byte Array: [65, 66, 67]
- Expected Output: Byte data written to "file.txt"
Q68. Write a Java program to create a temporary file.
- Input: File name: "tempfile.txt"
- Expected Output: Temporary file "tempfile.txt" created
Q69. Write a Java program to get the last modified time of a directory.
- Input: Directory: "testdirectory"
- Expected Output: Last modified time of "testdirectory"
Q70. Write a Java program to delete a directory and its contents recursively.
- Input: Directory: "testdirectory"
- Expected Output: "testdirectory" deleted along with its contents

Bikki Singh
Hi, I am the instructor of TechnoVlogs. I have a strong love for programming and enjoy teaching through practical examples. I made this site to help people improve their coding skills by solving real-world problems. With years of experience, my goal is to make learning programming easy and fun for everyone. Let's learn and grow together!