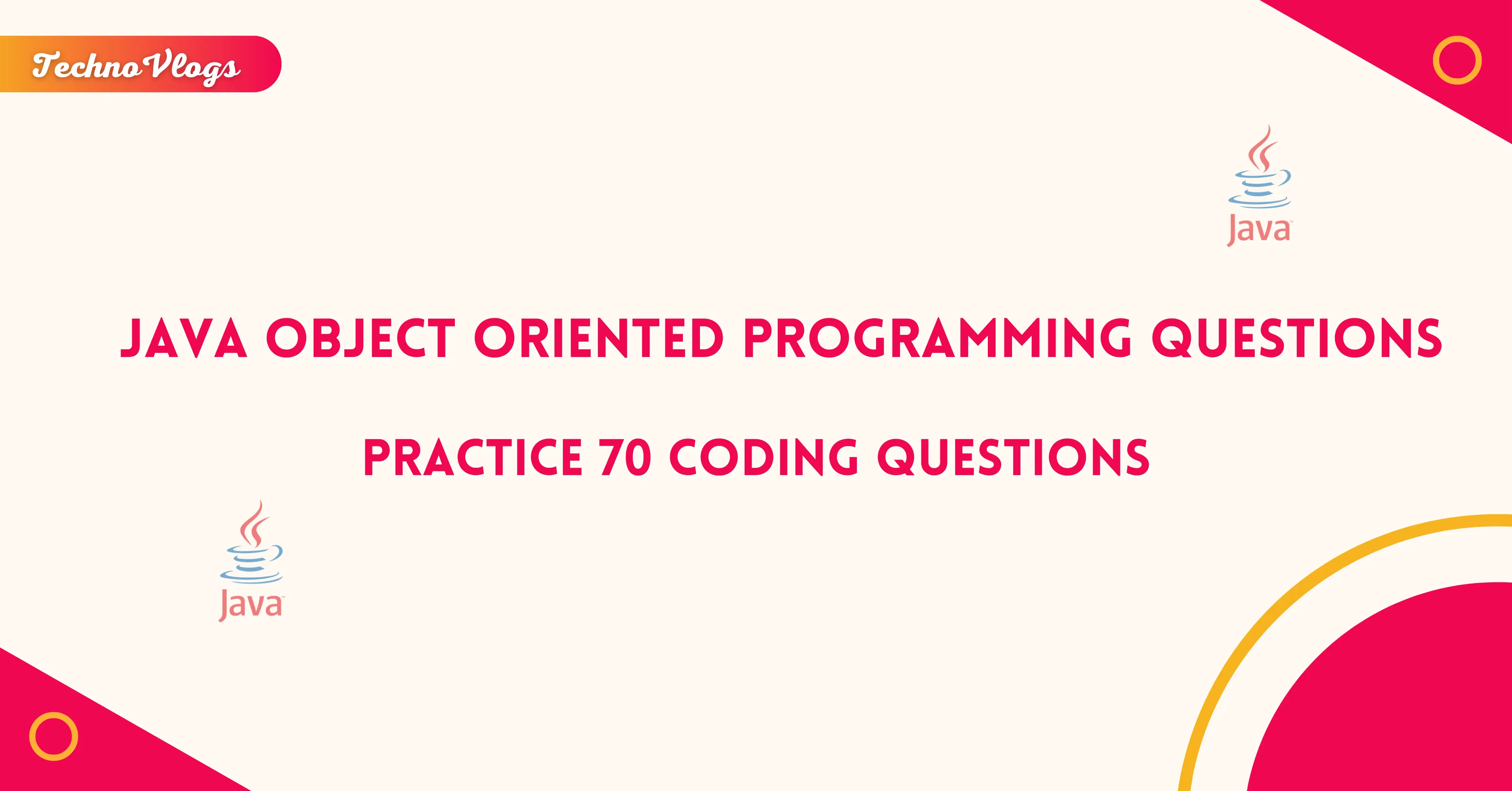
Practice 70 Java Object Oriented Programming Questions
Q1. Write a Java program to create a class Student with properties name, age, and rollNumber. Implement a constructor to initialize these properties and display them.
Input:
Name: John, Age: 20, Roll Number: 101
Expected Output:
Student Name: John
Age: 20
Roll Number: 101
Q2. Write a Java program to create a class Book with properties title, author, and price. Initialize these properties using the constructor and display them.
Input:
Title: Java Basics, Author: Alice, Price: 500
Expected Output:
Book Title: Java Basics
Author: Alice
Price: 500
Q3. Write a Java program to create a class Rectangle with properties length and width. Implement a method calculateArea() to compute the area of the rectangle.
Input:
Length: 5, Width: 4
Expected Output:
Area of Rectangle: 20
Q4. Write a Java program to create a class Employee with properties name, age, and salary. Write a method displayDetails() to display the details of an employee.
Input:
Name: Sarah, Age: 30, Salary: 40000
Expected Output:
Employee Name: Sarah
Age: 30
Salary: 40000
Q5. Write a Java program to create a class Car with properties brand, model, and year. Implement a constructor to initialize these properties.
Input:
Brand: Toyota, Model: Camry, Year: 2020
Expected Output:
Car Brand: Toyota
Model: Camry
Year: 2020
Q6. Write a Java program to create a class Person with properties firstName and lastName. Implement a method fullName() that returns the full name.
Input:
First Name: John, Last Name: Doe
Expected Output:
Full Name: John Doe
Q7. Write a Java program to create a class BankAccount with properties accountNumber and balance. Implement a method deposit() to deposit money into the account and a method withdraw() to withdraw money.
Input:
Account Number: 12345, Initial Balance: 10000, Deposit: 5000, Withdrawal: 3000
Expected Output:
Balance after deposit: 15000
Balance after withdrawal: 12000
Q8. Write a Java program to create a class Book with properties title, author, and price. Create an object of Book and display its details.
Input:
Title: Data Structures, Author: Bob, Price: 600
Expected Output:
Book Title: Data Structures
Author: Bob
Price: 600
Q9. Write a Java program to create a class Circle with a method calculateArea(). Use a constructor to initialize the radius.
Input:
Radius: 7
Expected Output:
Area of Circle: 153.94
Q10. Write a Java program to create a class Person with properties name, age, and address. Implement a constructor to initialize these properties and display them.
Input:
Name: Emma, Age: 25, Address: 123 Street, City
Expected Output:
Name: Emma
Age: 25
Address: 123 Street, City
Q11. Write a Java program to create a class Account with properties accountHolderName and balance. Create a method withdraw() and deposit() to manage the account balance.
Input:
Account Holder: Alex, Initial Balance: 10000, Deposit: 2000, Withdrawal: 3000
Expected Output:
Balance after deposit: 12000
Balance after withdrawal: 9000
Q12. Write a Java program to create a class Product with properties productName and price. Implement a method to display the product's details.
Input:
Product Name: Laptop, Price: 50000
Expected Output:
Product Name: Laptop
Price: 50000
Q13. Write a Java program to create a class Book with properties title, author, and price. Write a method applyDiscount() to apply a discount to the book price.
Input:
Title: Java Programming, Author: Alice, Price: 700, Discount: 10%
Expected Output:
Discounted Price: 630
Q14. Write a Java program to create a class Student with properties name, age, and marks. Create a method calculateGrade() based on the marks.
Input:
Name: Mark, Age: 20, Marks: 85
Expected Output:
Grade: A
Q15. Write a Java program to create a class Employee with properties name and salary. Implement a method increaseSalary() to increase the salary of an employee.
Input:
Name: Linda, Salary: 30000, Increment: 5000
Expected Output:
Salary after increment: 35000
Q16. Write a Java program to create a class Rectangle with methods setLength() and setWidth(). Implement a method calculatePerimeter() to calculate the perimeter of the rectangle.
Input:
Length: 5, Width: 3
Expected Output:
Perimeter of Rectangle: 16
Q17. Write a Java program to create a class Book with properties title, author, and price. Create a method applyTax() to apply a tax to the book price.
Input:
Title: Java Basics, Author: John, Price: 500, Tax: 15%
Expected Output:
Price after tax: 575
Q18. Write a Java program to create a class Circle with properties radius and a method calculateArea().
Input:
Radius: 5
Expected Output:
Area of Circle: 78.54
Q19. Write a Java program to create a class Person with properties name, age, and city. Implement a constructor to initialize these properties.
Input:
Name: Jack, Age: 28, City: New York
Expected Output:
Name: Jack
Age: 28
City: New York
Q20. Write a Java program to create a class Rectangle with properties length and width. Implement a method calculateArea() to calculate and display the area.
Input:
Length: 7, Width: 4
Expected Output:
Area of Rectangle: 28
Q21. Write a Java program to create a class Student with properties name, rollNo, and marks. Implement a method to display student details.
Input:
Name: Tom, Roll No: 10, Marks: 90
Expected Output:
Student Name: Tom
Roll Number: 10
Marks: 90
Q22. Write a Java program to create a class Product with properties productName and price. Write a method to display the product's details.
Input:
Product Name: Smartphone, Price: 20000
Expected Output:
Product Name: Smartphone
Price: 20000
Q23. Write a Java program to create a class Account with properties accountHolderName and balance. Write a method transferMoney() to transfer money from one account to another.
Input:
Account Holder 1: Steve, Initial Balance: 10000, Transfer Amount: 2000
Account Holder 2: Rachel, Initial Balance: 5000
Expected Output:
Balance after transfer: Steve - 8000, Rachel - 7000
Q24. Write a Java program to create a class Person with properties firstName, lastName, and address. Implement a method to display full name and address.
Input:
First Name: Amy, Last Name: Johnson, Address: 12 Baker Street
Expected Output:
Full Name: Amy Johnson
Address: 12 Baker Street
Q25. Write a Java program to create a class Book with properties title, author, and price. Write a method to calculate the discounted price after applying a percentage discount.
Input:
Title: Data Science, Author: Clara, Price: 900, Discount: 20%
Expected Output:
Discounted Price: 720
Q26. Write a Java program to create a class Employee with properties employeeId and salary. Write a method giveBonus() to give a bonus to the employee's salary.
Input:
Employee ID: E123, Salary: 30000, Bonus: 5000
Expected Output:
Salary after Bonus: 35000
Q27. Write a Java program to create a class Teacher with properties name and subject. Create an object and display the teacher's details.
Input:
Name: Dr. Smith, Subject: Math
Expected Output:
Teacher Name: Dr. Smith
Subject: Math
Q28. Write a Java program to create a class Student with properties name and marks. Create a method determineGrade() based on the student's marks.
Input:
Name: Lucy, Marks: 75
Expected Output:
Grade: B
Q29. Write a Java program to create a class Movie with properties title and rating. Implement a method displayInfo() to show the movie details.
Input:
Title: Inception, Rating: 9
Expected Output:
Movie Title: Inception
Rating: 9
Q30. Write a Java program to create a class Employee with properties name, age, and salary. Create a method to calculate the annual salary.
Input:
Name: Alice, Age: 35, Salary: 50000
Expected Output:
Annual Salary: 600000
Q31. Write a Java program to create a class Laptop with properties brand, model, and price. Create a method displayDetails() to display the laptop's details.
Input:
Brand: Dell, Model: XPS, Price: 120000
Expected Output:
Laptop Brand: Dell
Model: XPS
Price: 120000
Q32. Write a Java program to create a class Circle with properties radius. Implement a method calculateCircumference() to compute the circumference.
Input:
Radius: 10
Expected Output:
Circumference of Circle: 62.83
Q33. Write a Java program to create a class Student with properties name, age, and marks. Create a method isPassed() to determine whether the student passed or failed based on marks.
Input:
Name: Emma, Marks: 65
Expected Output:
Emma passed
Q34. Write a Java program to create a class Account with properties accountHolderName and balance. Create a method transferAmount() to transfer money from one account to another.
Input:
Account Holder: Alice, Balance: 5000, Transfer Amount: 1000
Expected Output:
Balance after transfer: 4000
Q35. Write a Java program to create a class Person with properties firstName, lastName, and age. Write a method displayInfo() to display the person's details.
Input:
First Name: John, Last Name: Doe, Age: 25
Expected Output:
Name: John Doe
Age: 25
Q36. Write a Java program to create a class Rectangle with properties length and width. Create a method isSquare() to check whether the rectangle is a square.
Input:
Length: 4, Width: 4
Expected Output:
It is a square.
Q37. Write a Java program to create a class Movie with properties title, genre, and rating. Create a method displayInfo() to display the movie information.
Input:
Title: Avatar, Genre: Action, Rating: 8.5
Expected Output:
Movie Title: Avatar
Genre: Action
Rating: 8.5
Q38. Write a Java program to create a class Person with properties firstName, lastName, and age. Implement a method isAdult() to check if the person is an adult (age >= 18).
Input:
First Name: Tim, Last Name: Brown, Age: 20
Expected Output:
Tim Brown is an adult.
Q39. Write a Java program to create a class Car with properties brand, model, and year. Implement a method to display car details.
Input:
Brand: Audi, Model: A6, Year: 2022
Expected Output:
Car Brand: Audi
Model: A6
Year: 2022
Q40. Write a Java program to create a class Course with properties courseName and duration. Write a method to display the course details.
Input:
Course Name: Java Programming, Duration: 3 months
Expected Output:
Course Name: Java Programming
Duration: 3 months
Q41. Write a Java program to create a class Person with properties name, age, and city. Create a method greet() to print a greeting message.
Input:
Name: Maria, Age: 29, City: Paris
Expected Output:
Hello, Maria from Paris!
Q42. Write a Java program to create a class Product with properties name, price, and quantity. Write a method to calculate the total price of a product based on quantity.
Input:
Product Name: T-shirt, Price: 500, Quantity: 2
Expected Output:
Total Price: 1000
Q43. Write a Java program to create a class Circle with properties radius. Implement a method calculateArea() to calculate the area of the circle.
Input:
Radius: 8
Expected Output:
Area of Circle: 201.06
Q44. Write a Java program to create a class Book with properties title, author, and price. Write a method to calculate the discounted price.
Input:
Title: Learn Java, Author: John, Price: 300, Discount: 15%
Expected Output:
Discounted Price: 255
Q45. Write a Java program to create a class BankAccount with properties accountNumber and balance. Implement a method deposit() to deposit an amount into the account.
Input:
Account Number: 1001, Initial Balance: 15000, Deposit: 5000
Expected Output:
Balance after deposit: 20000
Q46. Write a Java program to create a class Student with properties name, age, and marks. Create a method isPassed() to check if the student has passed.
Input:
Name: Oliver, Marks: 40
Expected Output:
Oliver has passed.
Q47. Write a Java program to create a class Employee with properties employeeId and salary. Write a method displayInfo() to display the employee's information.
Input:
Employee ID: E789, Salary: 40000
Expected Output:
Employee ID: E789
Salary: 40000
Q48. Write a Java program to create a class Circle with a method calculateCircumference(). Use a constructor to initialize the radius.
Input:
Radius: 5
Expected Output:
Circumference of Circle: 31.42
Q49. Write a Java program to create a class Book with properties title, author, and price. Write a method to calculate the total price after applying a discount and tax.
Input:
Title: Advanced Java, Author: Emily, Price: 400, Discount: 20%, Tax: 15%
Expected Output:
Total Price: 380
Q50. Write a Java program to create a class Student with properties name, marks, and status. Create a method determineStatus() based on marks (pass or fail).
Input:
Name: Nora, Marks: 45
Expected Output:
Nora has passed.
Q51. Write a Java program to create a class Person with properties firstName, lastName, and age. Implement a method isMinor() to check if the person is under 18.
Input:
First Name: Max, Last Name: Stone, Age: 16
Expected Output:
Max Stone is a minor.
Q52. Write a Java program to create a class Employee with properties name, age, and salary. Create a method calculateBonus() based on salary.
Input:
Name: James, Salary: 50000, Bonus Percentage: 10%
Expected Output:
Bonus: 5000
Q53. Write a Java program to create a class Rectangle with properties length and width. Create a method to calculate the perimeter of the rectangle.
Input:
Length: 6, Width: 4
Expected Output:
Perimeter of Rectangle: 20
Q54. Write a Java program to create a class Course with properties courseName and duration. Create a method to display the course information.
Input:
Course Name: Java Programming, Duration: 6 months
Expected Output:
Course Name: Java Programming
Duration: 6 months
Q55. Write a Java program to create a class Product with properties productName and price. Create a method to calculate the total price after applying a discount.
Input:
Product Name: Phone, Price: 20000, Discount: 10%
Expected Output:
Total Price after Discount: 18000
Q56. Write a Java program to create a class Car with properties brand, model, and year. Create a method to display the car's details.
Input:
Brand: BMW, Model: X5, Year: 2021
Expected Output:
Car Brand: BMW
Model: X5
Year: 2021
Q57. Write a Java program to create a class Teacher with properties name and subject. Implement a method teach() to display the teacher's lesson.
Input:
Name: Mr. Brown, Subject: Physics
Expected Output:
Mr. Brown teaches Physics.
Q58. Write a Java program to create a class Circle with properties radius. Implement a method to calculate the area.
Input:
Radius: 12
Expected Output:
Area of Circle: 452.38
Q59. Write a Java program to create a class BankAccount with properties accountNumber and balance. Create a method checkBalance() to display the account balance.
Input:
Account Number: 123456, Balance: 15000
Expected Output:
Account Balance: 15000
Q60. Write a Java program to create a class Employee with properties name and age. Create a method to display employee details.
Input:
Name: Brian, Age: 32
Expected Output:
Employee Name: Brian
Age: 32
Q61. Write a Java program to create a class Person with properties firstName, lastName, and city. Create a method displayDetails() to display the person's information.
Input:
First Name: Susan, Last Name: Clark, City: Los Angeles
Expected Output:
Name: Susan Clark
City: Los Angeles
Q62. Write a Java program to create a class Rectangle with properties length and width. Write a method isSquare() to check if the rectangle is a square.
Input:
Length: 6, Width: 6
Expected Output:
It is a square.
Q63. Write a Java program to create a class Person with properties firstName, lastName, and address. Implement a method fullName() to return the full name.
Input:
First Name: Mary, Last Name: Adams
Expected Output:
Full Name: Mary Adams
Q64. Write a Java program to create a class Course with properties courseName and duration. Implement a method to display the course duration.
Input:
Course Name: Python Programming, Duration: 4 months
Expected Output:
Course Duration: 4 months
Q65. Write a Java program to create a class Student with properties name, rollNo, and marks. Create a method passOrFail() to determine whether the student passed or failed.
Input:
Name: Chris, Marks: 30
Expected Output:
Chris has failed.
Q66. Write a Java program to create a class Product with properties productName and price. Write a method to display the product details.
Input:
Product Name: Television, Price: 30000
Expected Output:
Product Name: Television
Price: 30000
Q67. Write a Java program to create a class Movie with properties title, genre, and rating. Create a method displayInfo() to show the movie information.
Input:
Title: Interstellar, Genre: Sci-Fi, Rating: 9.5
Expected Output:
Movie Title: Interstellar
Genre: Sci-Fi
Rating: 9.5
Q68. Write a Java program to create a class Employee with properties name, age, and salary. Create a method increaseSalary() to increase the salary.
Input:
Name: John, Salary: 30000, Increment: 4000
Expected Output:
Salary after increment: 34000
Q69. Write a Java program to create a class Teacher with properties name and subject. Implement a method to introduce the teacher.
Input:
Name: Mrs. Green, Subject: Chemistry
Expected Output:
I am Mrs. Green, a Chemistry teacher.
Q70. Write a Java program to create a class Book with properties title, author, and price. Write a method to apply a discount on the book price.
Input:
Title: Data Science, Author: Alex, Price: 1000, Discount: 15%
Expected Output:
Discounted Price: 850

Bikki Singh
Hi, I am the instructor of TechnoVlogs. I have a strong love for programming and enjoy teaching through practical examples. I made this site to help people improve their coding skills by solving real-world problems. With years of experience, my goal is to make learning programming easy and fun for everyone. Let's learn and grow together!