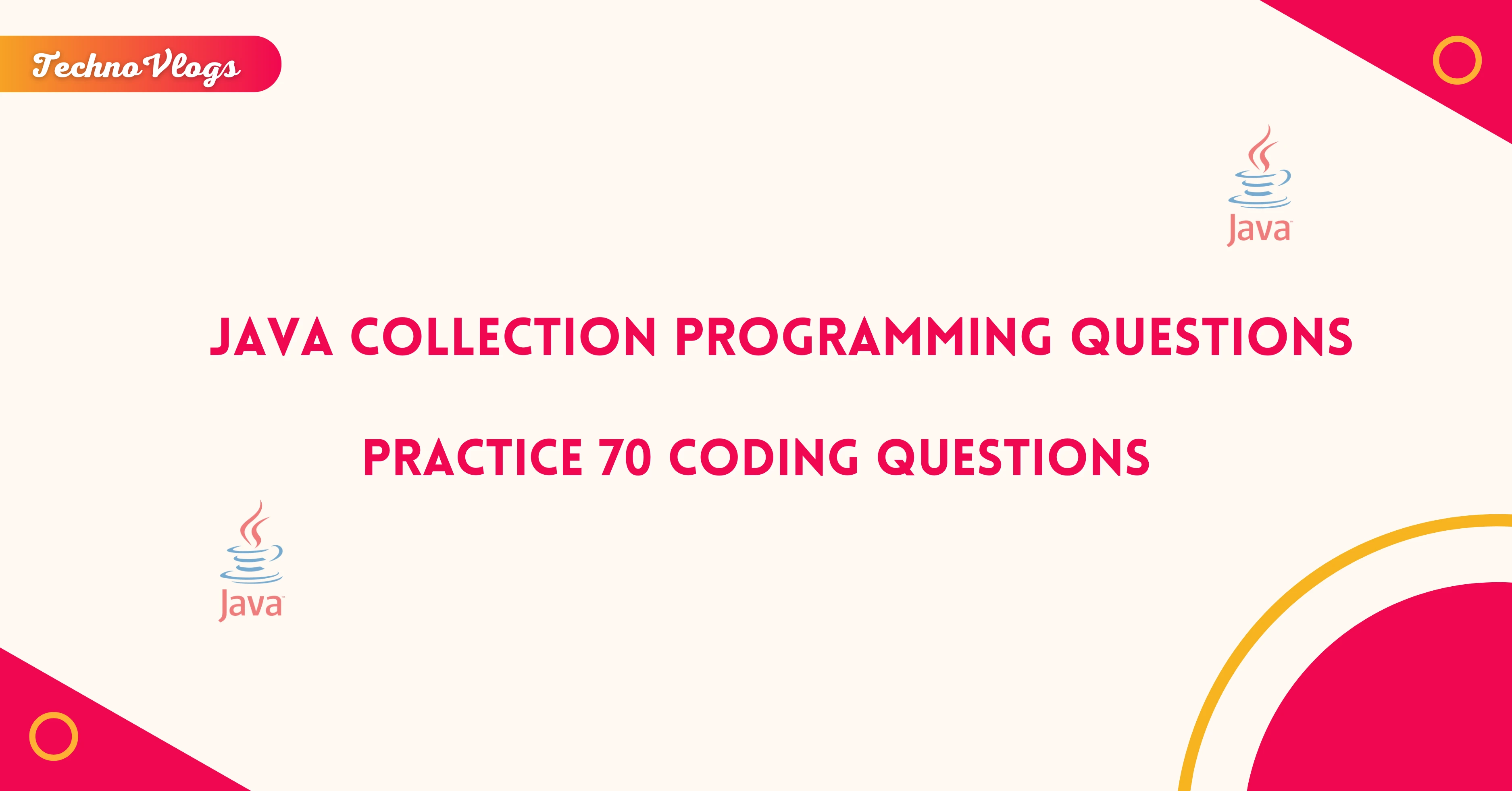
Practice 70 Java Collection Programming Questions
Q1. Write a Java program to demonstrate the use of ArrayList.
- Input: Add(10), Add(20), Remove(10)
- Expected Output: [20]
Q2. Write a Java program to find the size of an ArrayList.
- Input: Add(10), Add(20)
- Expected Output: 2
Q3. Write a Java program to add elements to a LinkedList and print them.
- Input: Add(10), Add(20), Add(30)
- Expected Output: [10, 20, 30]
Q4. Write a Java program to remove the first element of a LinkedList.
- Input: Add(10), Add(20), RemoveFirst()
- Expected Output: [20]
Q5. Write a Java program to remove the last element of a LinkedList.
- Input: Add(10), Add(20), RemoveLast()
- Expected Output: [10]
Q6. Write a Java program to demonstrate the use of HashSet.
- Input: Add(10), Add(20), Add(20)
- Expected Output: [10, 20]
Q7. Write a Java program to check if a HashSet contains a specific element.
- Input: Add(10), Add(20), Contains(10)
- Expected Output: True
Q8. Write a Java program to remove an element from a HashSet.
- Input: Add(10), Add(20), Remove(10)
- Expected Output: [20]
Q9. Write a Java program to demonstrate the use of TreeSet.
- Input: Add(10), Add(20), Add(5)
- Expected Output: [5, 10, 20]
Q10. Write a Java program to check if a TreeSet is empty.
- Input: Add(10), Add(20), IsEmpty()
- Expected Output: False
Q11. Write a Java program to demonstrate the use of HashMap.
- Input: Put("a", 10), Put("b", 20)
- Expected Output: {a=10, b=20}
Q12. Write a Java program to get a value from a HashMap.
- Input: Put("a", 10), Get("a")
- Expected Output: 10
Q13. Write a Java program to remove a key-value pair from a HashMap.
- Input: Put("a", 10), Remove("a")
- Expected Output: {}
Q14. Write a Java program to check if a HashMap contains a specific key.
- Input: Put("a", 10), ContainsKey("a")
- Expected Output: True
Q15. Write a Java program to demonstrate the use of TreeMap.
- Input: Put("a", 10), Put("b", 20)
- Expected Output: {a=10, b=20}
Q16. Write a Java program to check if a TreeMap contains a specific value.
- Input: Put("a", 10), ContainsValue(10)
- Expected Output: True
Q17. Write a Java program to iterate over the elements of a HashSet.
- Input: Add(10), Add(20), Add(30)
- Expected Output: 10 20 30
Q18. Write a Java program to iterate over the elements of an ArrayList.
- Input: Add(10), Add(20), Add(30)
- Expected Output: 10 20 30
Q19. Write a Java program to check if a List contains a specific element.
- Input: Add(10), Add(20), Contains(20)
- Expected Output: True
Q20. Write a Java program to reverse the elements of an ArrayList.
- Input: Add(10), Add(20), Add(30)
- Expected Output: [30, 20, 10]
Q21. Write a Java program to clear all elements from a LinkedList.
- Input: Add(10), Add(20), Clear()
- Expected Output: []
Q22. Write a Java program to add an element at a specific position in a List.
- Input: Add(10), Add(20), Add(1, 15)
- Expected Output: [10, 15, 20]
Q23. Write a Java program to convert a List to an Array.
- Input: Add(10), Add(20), ToArray()
- Expected Output: [10, 20]
Q24. Write a Java program to create a sublist from an ArrayList.
- Input: Add(10), Add(20), Add(30), Sublist(0, 2)
- Expected Output: [10, 20]
Q25. Write a Java program to demonstrate the use of PriorityQueue.
- Input: Add(10), Add(20), Add(15)
- Expected Output: [10, 15, 20]
Q26. Write a Java program to poll (remove) an element from a PriorityQueue.
- Input: Add(10), Add(20), Poll()
- Expected Output: 10
Q27. Write a Java program to add all elements of one List to another List.
- Input: Add(10), Add(20), AddAll([30, 40])
- Expected Output: [10, 20, 30, 40]
Q28. Write a Java program to check if a List is empty.
- Input: Add(10), IsEmpty()
- Expected Output: False
Q29. Write a Java program to create a List from an array.
- Input: Array([10, 20, 30])
- Expected Output: [10, 20, 30]
Q30. Write a Java program to add a collection to a List.
- Input: Add(10), Add(20), AddAll([30, 40])
- Expected Output: [10, 20, 30, 40]
Q31. Write a Java program to find the common elements between two sets.
- Input: Set1([10, 20, 30]), Set2([20, 30, 40])
- Expected Output: [20, 30]
Q32. Write a Java program to merge two lists.
- Input: List1([10, 20]), List2([30, 40])
- Expected Output: [10, 20, 30, 40]
Q33. Write a Java program to remove all elements from one List that are present in another List.
- Input: List1([10, 20, 30]), List2([20])
- Expected Output: [10, 30]
Q34. Write a Java program to find the index of an element in an ArrayList.
- Input: Add(10), Add(20), IndexOf(20)
- Expected Output: 1
Q35. Write a Java program to find the first and last occurrence of an element in a List.
- Input: Add(10), Add(20), Add(10), IndexOf(10), LastIndexOf(10)
- Expected Output: 0, 2
Q36. Write a Java program to add an element at the beginning of a LinkedList.
- Input: Add(10), AddFirst(5)
- Expected Output: [5, 10]
Q37. Write a Java program to find the sum of all elements in a List of Integers.
- Input: Add(10), Add(20), Add(30)
- Expected Output: 60
Q38. Write a Java program to create a HashSet and remove a specific element.
- Input: Add(10), Add(20), Remove(10)
- Expected Output: [20]
Q39. Write a Java program to check if a Map contains a specific value.
- Input: Put("a", 10), Put("b", 20), ContainsValue(20)
- Expected Output: True
Q40. Write a Java program to find the keys in a HashMap.
- Input: Put("a", 10), Put("b", 20)
- Expected Output: [a, b]
Q41. Write a Java program to find the values in a HashMap.
- Input: Put("a", 10), Put("b", 20)
- Expected Output: [10, 20]
Q42. Write a Java program to clone a HashMap.
- Input: Put("a", 10), Put("b", 20)
- Expected Output: {a=10, b=20}
Q43. Write a Java program to merge two HashMaps.
- Input: Map1({a=10}), Map2({b=20})
- Expected Output: {a=10, b=20}
Q44. Write a Java program to find the entry set of a HashMap.
- Input: Put("a", 10), Put("b", 20)
- Expected Output: [(a=10), (b=20)]
Q45. Write a Java program to sort a HashMap by keys.
- Input: Put("c", 30), Put("a", 10), Put("b", 20)
- Expected Output: {a=10, b=20, c=30}
Q46. Write a Java program to sort a HashMap by values.
- Input: Put("a", 30), Put("b", 10), Put("c", 20)
- Expected Output: {b=10, c=20, a=30}
Q47. Write a Java program to demonstrate the use of ArrayDeque.
- Input: AddFirst(10), AddLast(20), RemoveFirst()
- Expected Output: [20]
Q48. Write a Java program to check if a LinkedList contains an element.
- Input: Add(10), Add(20), Contains(20)
- Expected Output: True
Q49. Write a Java program to compare two ArrayLists.
- Input: List1([10, 20]), List2([10, 20])
- Expected Output: True
Q50. Write a Java program to find the maximum element in a List of Integers.
- Input: Add(10), Add(20), Add(30)
- Expected Output: 30
Q51. Write a Java program to shuffle elements of a List.
- Input: Add(10), Add(20), Add(30)
- Expected Output: Random order of elements
Q52. Write a Java program to convert a List to a Set.
- Input: Add(10), Add(20), Add(20)
- Expected Output: [10, 20]
Q53. Write a Java program to perform union of two sets.
- Input: Set1([10, 20]), Set2([20, 30])
- Expected Output: [10, 20, 30]
Q54. Write a Java program to perform intersection of two sets.
- Input: Set1([10, 20]), Set2([20, 30])
- Expected Output: [20]
Q55. Write a Java program to remove all elements from a HashSet that are not in another HashSet.
- Input: Set1([10, 20, 30]), Set2([20, 30])
- Expected Output: [20, 30]
Q56. Write a Java program to find the difference between two sets.
- Input: Set1([10, 20, 30]), Set2([20, 30])
- Expected Output: [10]
Q57. Write a Java program to find the first element in a List.
- Input: Add(10), Add(20)
- Expected Output: 10
Q58. Write a Java program to check if a list is a sublist of another list.
- Input: List1([10, 20]), List2([10])
- Expected Output: True
Q59. Write a Java program to create an empty ArrayList and add elements to it.
- Input: Add(10), Add(20)
- Expected Output: [10, 20]
Q60. Write a Java program to remove elements from a List by index.
- Input: Add(10), Add(20), Remove(0)
- Expected Output: [20]
Q61. Write a Java program to check if a LinkedList is a palindrome.
- Input: Add(10), Add(20), Add(10)
- Expected Output: True
Q62. Write a Java program to concatenate two Lists.
- Input: List1([10, 20]), List2([30, 40])
- Expected Output: [10, 20, 30, 40]
Q63. Write a Java program to find the first and last elements in a List.
- Input: Add(10), Add(20)
- Expected Output: 10, 20
Q64. Write a Java program to remove elements in a List by value.
- Input: Add(10), Add(20), Remove(10)
- Expected Output: [20]
Q65. Write a Java program to count the frequency of each element in a List.
- Input: Add(10), Add(20), Add(10)
- Expected Output: 10 -> 2, 20 -> 1
Q66. Write a Java program to create a LinkedHashSet and demonstrate its functionality.
- Input: Add(10), Add(20)
- Expected Output: [10, 20]
Q67. Write a Java program to compare two sets.
- Input: Set1([10, 20]), Set2([10, 20])
- Expected Output: True
Q68. Write a Java program to find the union of two Lists.
- Input: List1([10, 20]), List2([30, 40])
- Expected Output: [10, 20, 30, 40]
Q69. Write a Java program to convert a Set to a List.
- Input: Set([10, 20, 30])
- Expected Output: [10, 20, 30]
Q70. Write a Java program to find the first element in a HashSet.
- Input: Add(10), Add(20)
- Expected Output: 10

Bikki Singh
Hi, I am the instructor of TechnoVlogs. I have a strong love for programming and enjoy teaching through practical examples. I made this site to help people improve their coding skills by solving real-world problems. With years of experience, my goal is to make learning programming easy and fun for everyone. Let's learn and grow together!