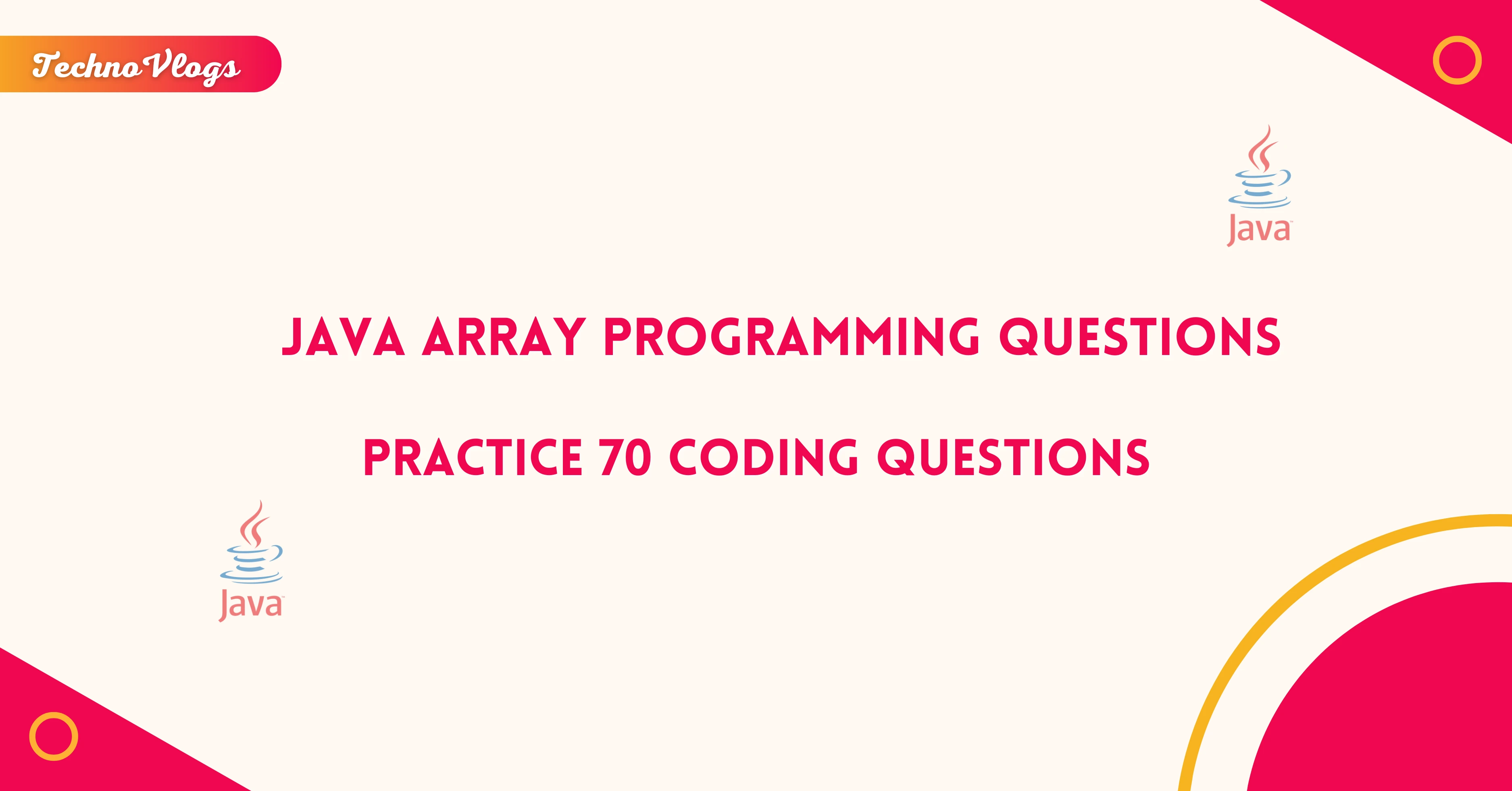
Practice 70 Java Array Programming Questions
Q1. Write a Java program to find the largest element in an array.
- Input: [5, 7, 2, 8, 3]
- Expected Output: 8
Q2. Write a Java program to find the smallest element in an array.
- Input: [12, 4, 9, 15, 6]
- Expected Output: 4
Q3. Write a Java program to calculate the sum of all elements in an array.
- Input: [3, 5, 7, 2]
- Expected Output: 17
Q4. Write a Java program to calculate the average of elements in an array.
- Input: [10, 20, 30, 40]
- Expected Output: 25
Q5. Write a Java program to reverse an array.
- Input: [1, 2, 3, 4, 5]
- Expected Output: [5, 4, 3, 2, 1]
Q6. Write a Java program to check if an array is sorted.
- Input: [1, 2, 3, 4, 5]
- Expected Output: Sorted
Q7. Write a Java program to find the second largest element in an array.
- Input: [10, 5, 8, 12, 7]
- Expected Output: 10
Q8. Write a Java program to count the number of even numbers in an array.
- Input: [1, 2, 3, 4, 5, 6]
- Expected Output: 3
Q9. Write a Java program to count the number of odd numbers in an array.
- Input: [1, 2, 3, 4, 5, 6]
- Expected Output: 3
Q10. Write a Java program to merge two arrays.
- Input: [1, 2, 3], [4, 5, 6]
- Expected Output: [1, 2, 3, 4, 5, 6]
Q11. Write a Java program to remove duplicates from an array.
- Input: [1, 2, 2, 3, 4, 4]
- Expected Output: [1, 2, 3, 4]
Q12. Write a Java program to find the frequency of each element in an array.
- Input: [1, 2, 2, 3, 3, 3]
- Expected Output: 1 -> 1, 2 -> 2, 3 -> 3
Q13. Write a Java program to rotate an array to the right by one position.
- Input: [1, 2, 3, 4, 5]
- Expected Output: [5, 1, 2, 3, 4]
Q14. Write a Java program to find the common elements between two arrays.
- Input: [1, 2, 3], [2, 3, 4]
- Expected Output: [2, 3]
Q15. Write a Java program to find the union of two arrays.
- Input: [1, 2, 3], [3, 4, 5]
- Expected Output: [1, 2, 3, 4, 5]
Q16. Write a Java program to find the intersection of two arrays.
- Input: [1, 2, 3], [2, 3, 4]
- Expected Output: [2, 3]
Q17. Write a Java program to print all subarrays of an array.
- Input: [1, 2, 3]
- Expected Output: [1], [1, 2], [1, 2, 3], [2], [2, 3], [3]
Q18. Write a Java program to find the missing number in an array of 1 to N.
- Input: [1, 2, 4, 5]
- Expected Output: 3
Q19. Write a Java program to sort an array in ascending order.
- Input: [5, 2, 9, 1, 5, 6]
- Expected Output: [1, 2, 5, 5, 6, 9]
Q20. Write a Java program to sort an array in descending order.
- Input: [5, 2, 9, 1, 5, 6]
- Expected Output: [9, 6, 5, 5, 2, 1]
Q21. Write a Java program to find the largest contiguous subarray sum.
- Input: [-2, 1, -3, 4, -1, 2, 1, -5, 4]
- Expected Output: 6
Q22. Write a Java program to print the array elements in reverse order.
- Input: [1, 2, 3, 4]
- Expected Output: [4, 3, 2, 1]
Q23. Write a Java program to find the first non-repeating element in an array.
- Input: [4, 5, 2, 4, 5, 7, 8]
- Expected Output: 2
Q24. Write a Java program to find the sum of the diagonal elements of a square matrix.
- Input: [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
- Expected Output: 15
Q25. Write a Java program to find the index of an element in an array.
- Input: [10, 20, 30, 40], Element: 30
- Expected Output: 2
Q26. Write a Java program to check if two arrays are equal.
- Input: [1, 2, 3], [1, 2, 3]
- Expected Output: True
Q27. Write a Java program to check if an array contains a given number.
- Input: [10, 20, 30], Number: 20
- Expected Output: True
Q28. Write a Java program to find the sum of odd-indexed elements in an array.
- Input: [1, 2, 3, 4, 5]
- Expected Output: 6
Q29. Write a Java program to find the sum of even-indexed elements in an array.
- Input: [1, 2, 3, 4, 5]
- Expected Output: 9
Q30. Write a Java program to find the product of elements in an array.
- Input: [1, 2, 3, 4]
- Expected Output: 24
Q31. Write a Java program to check if an array contains duplicate elements.
- Input: [1, 2, 3, 1]
- Expected Output: True
Q32. Write a Java program to move all zeroes to the end of an array.
- Input: [0, 1, 0, 3, 12]
- Expected Output: [1, 3, 12, 0, 0]
Q33. Write a Java program to find the element that appears most in an array.
- Input: [3, 3, 2, 1, 3, 4]
- Expected Output: 3
Q34. Write a Java program to find the median of an array.
- Input: [1, 2, 3, 4, 5]
- Expected Output: 3
Q35. Write a Java program to find the sum of all positive numbers in an array.
- Input: [-1, 2, 3, -4, 5]
- Expected Output: 10
Q36. Write a Java program to find the sum of all negative numbers in an array.
- Input: [-1, 2, 3, -4, 5]
- Expected Output: -5
Q37. Write a Java program to find the difference between the largest and smallest elements in an array.
- Input: [7, 2, 9, 4, 3]
- Expected Output: 7
Q38. Write a Java program to count the number of positive numbers in an array.
- Input: [-1, 2, 3, -4, 5]
- Expected Output: 3
Q39. Write a Java program to check if an array is a palindrome.
- Input: [1, 2, 3, 2, 1]
- Expected Output: True
Q40. Write a Java program to remove an element from an array.
- Input: [10, 20, 30, 40], Remove: 20
- Expected Output: [10, 30, 40]
Q41. Write a Java program to check if an array contains only positive numbers.
- Input: [1, 2, 3, 4]
- Expected Output: True
Q42. Write a Java program to check if an array contains only negative numbers.
- Input: [-1, -2, -3, -4]
- Expected Output: True
Q43. Write a Java program to find the sum of the squares of all elements in an array.
- Input: [1, 2, 3]
- Expected Output: 14
Q44. Write a Java program to find the product of all elements in an array.
- Input: [1, 2, 3, 4]
- Expected Output: 24
Q45. Write a Java program to find the length of an array.
- Input: [10, 20, 30, 40]
- Expected Output: 4
Q46. Write a Java program to find the index of the smallest element in an array.
- Input: [4, 5, 1, 2]
- Expected Output: 2
Q47. Write a Java program to find the index of the largest element in an array.
- Input: [4, 5, 1, 2]
- Expected Output: 1
Q48. Write a Java program to concatenate two arrays.
- Input: [1, 2, 3], [4, 5, 6]
- Expected Output: [1, 2, 3, 4, 5, 6]
Q49. Write a Java program to find the element at a specific index in an array.
- Input: [1, 2, 3, 4], Index: 2
- Expected Output: 3
Q50. Write a Java program to print the unique elements in an array.
- Input: [1, 2, 3, 1, 2, 4]
- Expected Output: [3, 4]
Q51. Write a Java program to find the largest number in each row of a 2D array.
- Input: [[1, 2], [3, 4], [5, 6]]
- Expected Output: 2, 4, 6
Q52. Write a Java program to count the occurrences of a specific number in an array.
- Input: [1, 2, 2, 3, 4, 2], Number: 2
- Expected Output: 3
Q53. Write a Java program to find the even-indexed elements in an array.
- Input: [1, 2, 3, 4, 5]
- Expected Output: [1, 3, 5]
Q54. Write a Java program to find the odd-indexed elements in an array.
- Input: [1, 2, 3, 4, 5]
- Expected Output: [2, 4]
Q55. Write a Java program to replace all elements in an array with 0.
- Input: [1, 2, 3, 4]
- Expected Output: [0, 0, 0, 0]
Q56. Write a Java program to count the number of vowels in an array of characters.
- Input: ['a', 'b', 'c', 'e', 'i']
- Expected Output: 3
Q57. Write a Java program to find the maximum product of two numbers in an array.
- Input: [1, 5, 3, 7, 9]
- Expected Output: 63
Q58. Write a Java program to find the minimum product of two numbers in an array.
- Input: [1, 5, 3, 7, 9]
- Expected Output: 3
Q59. Write a Java program to find the sum of the elements at odd positions in an array.
- Input: [1, 2, 3, 4, 5]
- Expected Output: 6
Q60. Write a Java program to find the sum of the elements at even positions in an array.
- Input: [1, 2, 3, 4, 5]
- Expected Output: 9
Q61. Write a Java program to perform binary search on a sorted array.
- Input: [1, 2, 3, 4, 5], Search: 3
- Expected Output: Index 2
Q62. Write a Java program to count the number of elements greater than a given number in an array.
- Input: [1, 2, 3, 4, 5], Number: 3
- Expected Output: 2
Q63. Write a Java program to check if an array is a subset of another array.
- Input: [1, 2, 3], [2, 3]
- Expected Output: True
Q64. Write a Java program to check if an array is a superset of another array.
- Input: [1, 2, 3], [2, 3]
- Expected Output: True
Q65. Write a Java program to swap two arrays.
- Input: [1, 2], [3, 4]
- Expected Output: [3, 4], [1, 2]
Q66. Write a Java program to check if an array is symmetric.
- Input: [1, 2, 3, 2, 1]
- Expected Output: True
Q67. Write a Java program to print the sum of all elements in a 2D array.
- Input: [[1, 2], [3, 4], [5, 6]]
- Expected Output: 21
Q68. Write a Java program to find the product of all elements in a 2D array.
- Input: [[1, 2], [3, 4], [5, 6]]
- Expected Output: 720
Q69. Write a Java program to find the transpose of a matrix.
- Input: [[1, 2], [3, 4]]
- Expected Output: [[1, 3], [2, 4]]
Q70. Write a Java program to check if an array is a magic square.
- Input: [[8, 1, 6], [3, 5, 7], [4, 9, 2]]
- Expected Output: True

Bikki Singh
Hi, I am the instructor of TechnoVlogs. I have a strong love for programming and enjoy teaching through practical examples. I made this site to help people improve their coding skills by solving real-world problems. With years of experience, my goal is to make learning programming easy and fun for everyone. Let's learn and grow together!