
Practice Top C Programming Questions on Searching and Sorting
Q1. Write a program to search for an element in an array using the linear search method.
Input: Array: [2, 5, 8, 1, 3], Search: 8
Output: Element found at index 2
Q2. Write a program to search for an element in a sorted array using binary search.
Input: Array: [1, 3, 5, 7, 9], Search: 7
Output: Element found at index 3
Q3. Write a program to find the first occurrence of an element in an array.
Input: Array: [4, 5, 6, 5, 7], Search: 5
Output: First occurrence at index 1
Q4. Write a program to find the last occurrence of an element in an array.
Input: Array: [4, 5, 6, 5, 7], Search: 5
Output: Last occurrence at index 3
Q5. Write a program to count how many times an element appears in an array.
Input: Array: [3, 3, 5, 3, 7], Search: 3
Output: Element occurs 3 times
Q6. Write a program to find the starting and ending positions of a target element in a sorted array.
Input: Array: [2, 3, 3, 3, 4], Search: 3
Output: Range: 1 to 3
Q7. Write a program to find the smallest element in an array.
Input: Array: [9, 3, 5, 2, 7]
Output: Smallest element: 2
Q8. Write a program to find the largest element in an array.
Input: Array: [9, 3, 5, 2, 7]
Output: Largest element: 9
Q9. Write a program to find the missing number in an array of 1 to n.
Input: Array: [1, 2, 4, 5], n = 5
Output: Missing number: 3
Q10. Write a program to find a duplicate element in an array.
Input: Array: [1, 2, 3, 4, 2]
Output: Duplicate element: 2
Q11. Write a program to sort an array using bubble sort.
Input: Array: [5, 2, 9, 1, 5]
Output: Sorted Array: [1, 2, 5, 5, 9]
Q12. Write a program to sort an array using selection sort.
Input: Array: [8, 4, 2, 9]
Output: Sorted Array: [2, 4, 8, 9]
Q13. Write a program to sort an array using insertion sort.
Input: Array: [7, 3, 5, 2]
Output: Sorted Array: [2, 3, 5, 7]
Q14. Write a program to merge two sorted arrays into one sorted array.
Input: Arrays: [1, 3, 5] and [2, 4, 6]
Output: Merged Array: [1, 2, 3, 4, 5, 6]
Q15. Write a program to implement quick sort.
Input: Array: [10, 7, 8, 9, 1, 5]
Output: Sorted Array: [1, 5, 7, 8, 9, 10]
Q16. Write a program to implement count sort for a range of integers.
Input: Array: [4, 2, 2, 8, 3, 3, 1]
Output: Sorted Array: [1, 2, 2, 3, 3, 4, 8]
Q17. Write a program to sort an array in descending order.
Input: Array: [4, 7, 1, 9]
Output: Sorted Array: [9, 7, 4, 1]
Q18. Write a program to sort an array of strings alphabetically.
Input: ["banana", "apple", "cherry"]
Output: ["apple", "banana", "cherry"]
Q19. Write a program to sort even numbers and odd numbers separately in an array.
Input: Array: [9, 3, 4, 8, 1]
Output: Even: [4, 8], Odd: [1, 3, 9]
Q20. Write a program to find the Kth smallest element in an array.
Input: Array: [7, 10, 4, 3, 20, 15], K = 3
Output: Kth smallest element: 7
Q21. Write a program to sort an array using pointers.
Input: Array: [5, 2, 9]
Output: Sorted Array: [2, 5, 9]
Q22. Write a program to implement binary search for a descending sorted array.
Input: Array: [9, 7, 5, 3], Search: 7
Output: Element found at index 1
Q23. Write a program to search for an element in a rotated sorted array.
Input: Array: [4, 5, 6, 7, 0, 1, 2], Search: 0
Output: Element found at index 4
Q24. Write a program to sort elements by frequency in an array.
Input: [4, 4, 5, 6, 6, 6, 5]
Output: [6, 6, 6, 5, 5, 4, 4]
Q25. Write a program to sort an array by absolute values.
Input: [-4, 2, -1, 3]
Output: [2, -1, 3, -4]
Q26. Write a program to find the median of a sorted array.
Input: [1, 2, 3, 4, 5]
Output: Median: 3
Q27. Write a program to sort each row of a matrix.
Input: Matrix [[3, 2], [1, 4]]
Output: [[2, 3], [1, 4]]
Q28. Write a program to count distinct elements in an array.
Input: [1, 2, 2, 3]
Output: Distinct Count: 3
Q29. Write a program to find the intersection of two arrays.
Input: [1, 2, 3] and [2, 3, 4]
Output: [2, 3]
Q30. Write a program to sort an array based on a custom comparator function.
Input: [1, 4, 3] (Sort by even first)
Output: [4, 1, 3]
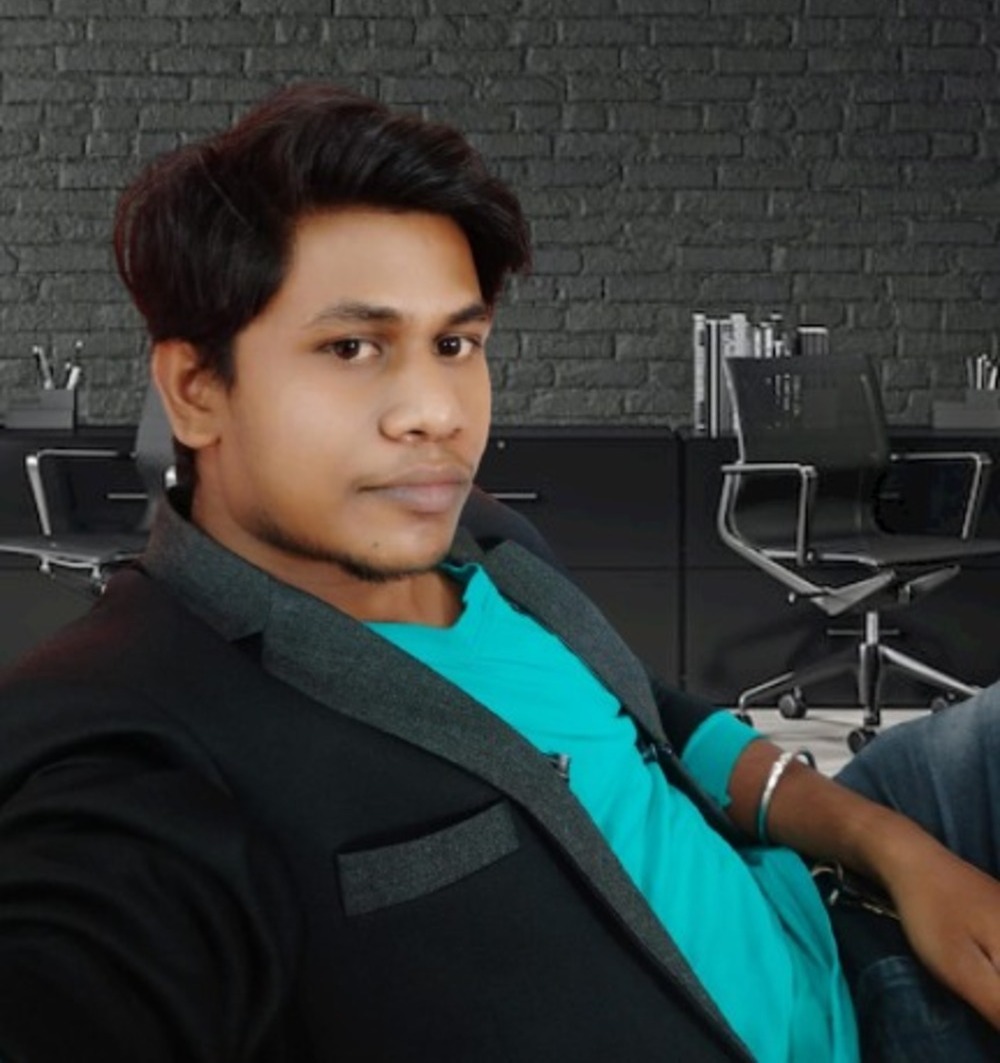
Bikki Singh
Hi, I am the instructor of TechnoVlogs. I have a strong love for programming and enjoy teaching through practical examples. I made this site to help people improve their coding skills by solving real-world problems. With years of experience, my goal is to make learning programming easy and fun for everyone. Let's learn and grow together!