
30 Important C programming questions on the while loop
Q 1. Write a program to print numbers from 1 to 10 using a `while` loop.
Expected Output:
1 2 3 4 5 6 7 8 9 10
Q 2. Write a program to print even numbers between 1 and 20 using a `while` loop.
Expected Output:
2 4 6 8 10 12 14 16 18 20
Q 3. Write a program to find the sum of the first 10 natural numbers using a `while` loop.
Expected Output:
Sum = 55
Q 4. Write a program to reverse the digits of a given number using a `while` loop. For example, if the number is 12345, the output should be 54321.
Expected Output:
Enter a number: 12345
Reversed Number = 54321
Q 5. Write a program to count the number of digits in a given number using a `while` loop.
Expected Output:
Enter a number: 12345
Number of digits = 5
Q 6. Write a program to find the factorial of a given number using a `while` loop.
Expected Output:
Enter a number: 5
Factorial = 120
Q 7. Write a program to check if a given number is prime using a `while` loop.
Expected Output:
Enter a number: 7
7 is a prime number
Q 8. Write a program to print the multiplication table of a given number using a `while` loop.
Expected Output:
Enter a number: 5
5 x 1 = 5
5 x 2 = 10
5 x 3 = 15
...
5 x 10 = 50
Q 9. Write a program to find the GCD (Greatest Common Divisor) of two numbers using a `while` loop.
Expected Output:
Enter two numbers: 56 98
GCD = 14
Q 10. Write a program to calculate the sum of the digits of a given number using a `while` loop.
Expected Output:
Enter a number: 12345
Sum of digits = 15
Q 11. Write a program to calculate the power of a number using a `while` loop.
Expected Output:
Enter base and exponent: 2 3
2^3 = 8
Q 12. Write a program to find the LCM (Least Common Multiple) of two numbers using a `while` loop.
Expected Output:
Enter two numbers: 15 20
LCM = 60
Q 13. Write a program to print the Fibonacci series up to `n` terms using a `while` loop.
Expected Output:
Enter number of terms: 10
0 1 1 2 3 5 8 13 21 34
Q 14. Write a program to check if a given number is a palindrome using a `while` loop.
Expected Output:
Enter a number: 12321
12321 is a palindrome
Q 15. Write a program to find the sum of the first `n` natural numbers using a `while` loop.
Expected Output:
Enter a number: 10
Sum = 55
Q 16. Write a program to print all odd numbers between 1 and 50 using a `while` loop.
Expected Output:
1 3 5 7 9 11 13 15 17 19 21 23 25 27 29 31 33 35 37 39 41 43 45 47 49
Q 17. Write a program to calculate the average of `n` numbers using a `while` loop.
Expected Output:
Enter the number of elements: 5
Enter 5 numbers: 10 20 30 40 50
Average = 30
Q 18. Write a program to find the sum of squares of the first `n` natural numbers using a `while` loop.
Expected Output:
Enter a number: 3
Sum of squares = 14
Q 19. Write a program to generate and print the first `n` prime numbers using a `while` loop.
Expected Output:
Enter the number of prime numbers: 5
2 3 5 7 11
Q 20. Write a program to print the following pattern using a `while` loop:
*
**
***
****
*****
Q 21. Write a program to check if a given number is an Armstrong number using a `while` loop. (An Armstrong number is an integer such that the sum of the cubes of its digits is equal to the number itself.)
Expected Output:
Enter a number: 153
153 is an Armstrong number
Q 22. Write a program to find the sum of the first `n` terms of an arithmetic progression (AP) using a `while` loop. The first term and common difference should be provided by the user.
Expected Output:
Enter first term, common difference, and number of terms: 2 3 5
Sum = 40
Q 23. Write a program to check if a given number is a perfect number using a `while` loop. (A perfect number is a number that is equal to the sum of its divisors, excluding itself.)
Expected Output:
Enter a number: 6
6 is a perfect number
Q 24. Write a program to convert a given decimal number to binary using a `while` loop.
Expected Output:
Enter a decimal number: 10
Binary = 1010
Q 25. Write a program to calculate compound interest using a `while` loop. The formula for compound interest is `A=P(1+rn)nt`, where `P` is the principal amount, `r` is the annual interest rate, `n` is the number of times interest is compounded per year, and `t` is the number of years.
Expected Output:
Enter principal, rate, time, and number of times compounded: 1000 5 2 4
Compound Interest = 1104.94
Q 26. Write a program to print all prime numbers between 1 and 100 using a `while` loop.
Expected Output:
2 3 5 7 11 13 17 19 23 29 31 37 41 43 47 53 59 61 67 71 73 79 83 89 97
Q 27. Write a program to calculate the product of the digits of a given number using a `while` loop.
Expected Output:
Enter a number: 123
Product of digits = 6
Q 28. Write a program to print the first `n` multiples of a given number using a `while` loop.
Expected Output:
Enter a number: 3
Enter the number of multiples: 5
3 6 9 12 15
Q 29. Write a program to find the average of the digits in a given number using a `while` loop.
Expected Output:
Enter a number: 12345
Average of digits = 3.00
Q 30. Write a program to check if a given number is a strong number using a `while` loop. (A strong number is a number in which the sum of the factorial of its digits is equal to the number itself.)
Expected Output:
Enter a number: 145
145 is a strong number
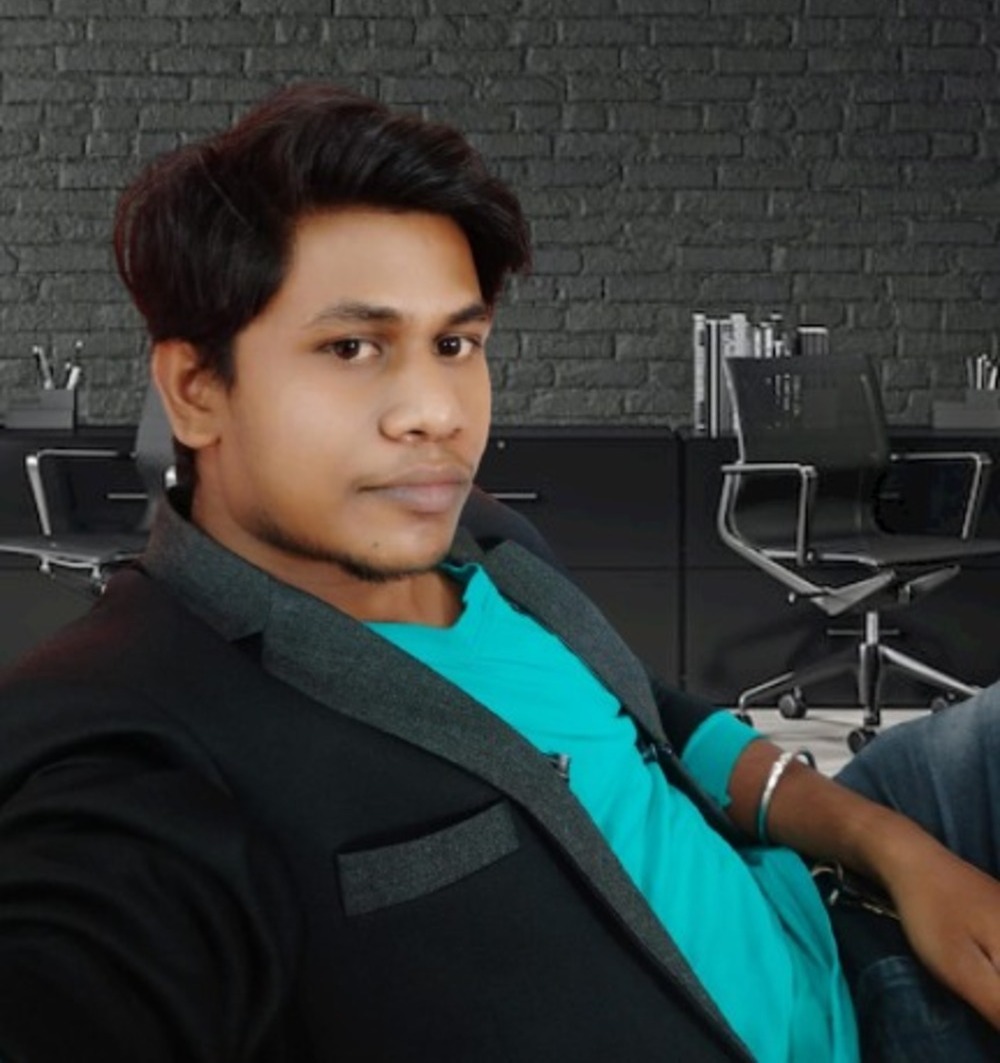
Bikki Singh
Hi, I am the instructor of TechnoVlogs. I have a strong love for programming and enjoy teaching through practical examples. I made this site to help people improve their coding skills by solving real-world problems. With years of experience, my goal is to make learning programming easy and fun for everyone. Let's learn and grow together!