
Top 30 Programming Questions on Arrays in C Programming
Q 1. Initialize an array of size 5 with values 1, 2, 3, 4, 5 and print them.
Expected Output:
1 2 3 4 5
Q 2. Find the sum of elements in an array `[10, 20, 30, 40, 50]`.
Expected Output:
Sum = 150
Q 3. Reverse the array `[1, 2, 3, 4, 5]` and print it.
Expected Output:
5 4 3 2 1
Q 4. Find the largest element in the array `[5, 10, 15, 2, 20, 25]`.
Expected Output:
Largest element = 25
Q 5. Count the number of even numbers in the array `[1, 2, 3, 4, 5, 6, 7, 8, 9, 10]`.
Expected Output:
Even numbers count = 5
Q 6. Copy elements from one array to another.
Input: arr1 = [1, 2, 3, 4, 5]
Expected Output:
arr2 = 1 2 3 4 5
Q 7. Find the smallest element in the array [9, 5, 3, 7, 1].
Expected Output:
Smallest element = 1
Q 8. Sort the array `[5, 2, 9, 1, 7]` in ascending order.
Expected Output:
1 2 5 7 9
Q 9. Merge two arrays [1, 2, 3] and [4, 5, 6].
Expected Output:
Merged array = 1 2 3 4 5 6
Q 10. Insert an element into the array `[1, 2, 4, 5]` at position 3.
Expected Output:
Array after insertion: 1 2 3 4 5
Q 11. Delete an element at position 2 from array `[1, 2, 3, 4, 5]`.
Expected Output:
Array after deletion: 1 2 4 5
Q 12. Check if a given element (say 3) exists in the array `[1, 2, 3, 4, 5]`.
Expected Output:
Element found at index 2
Q 13. Shift all elements of the array `[1,2, 3, 4, 5]` one position to the right.
Expected Output:
5 1 2 3 4
Q 14. Find the second largest element in the array `[12, 35, 1, 10, 34, 1]`.
Expected Output:
Second largest = 34
Q 15. Count the occurrences of an element (say 3) in the array `[1, 2, 3, 4, 3, 5, 3]`.
Expected Output:
Occurrences of 3 = 3
Q 16. Find the sum of all odd numbers in the array `[1, 2, 3, 4, 5, 6, 7]`.
Expected Output:
Sum of odd numbers = 16
Q 17. Check if an array `[1, 2, 3, 4, 5]` is sorted in ascending order.
Expected Output:
Array is sorted
Q 18. Find the average of elements in the array `[10, 20, 30, 40, 50]`.
Expected Output:
Average = 30
Q 19. Count the number of negative numbers in the array `[1, -2, 3, -4, 5]`.
Expected Output:
Negative numbers count = 2
Q 20. Multiply each element of the array `[1, 2, 3, 4, 5]` by 2 and print the new array.
Expected Output:
2 4 6 8 10
Q 21. Find the difference between the largest and smallest element in the array `[15, 22, 8, 19, 31]`.
Expected Output:
Difference = 23
Q 22. Find the product of all elements in the array `[1, 2, 3, 4, 5]`.
Expected Output:
Product = 120
Q 23. Find the frequency of each element in the array `[1, 2, 3, 2, 4, 1, 3, 5]`.
Expected Output:
Element 1 occurs 2 times
Element 2 occurs 2 times
Element 3 occurs 2 times
Element 4 occurs 1 times
Element 5 occurs 1 times
Q 24. Replace every negative number in an array with 0. Example: `[1, -2, 3, -4, 5]`.
Expected Output:
1 0 3 0 5
Q 25. Find the index of the first occurrence of the number 4 in the array `[3, 4, 5, 4, 6]`.
Expected Output:
Index of first occurrence of 4 = 1
Q 26. Split the array `[1, 2, 3, 4, 5, 6]` into two equal halves and print them.
Expected Output:
First half: 1 2 3
Second half: 4 5 6
Q 27. Check if two arrays `[1, 2, 3]` and `[1, 2, 3]` are identical.
Expected Output:
Arrays are identical
Q 28. Rotate the array `[1, 2, 3, 4, 5]` by two positions to the left.
Expected Output:
3 4 5 1 2
Q 29. Count the number of prime numbers in the array `[2, 3, 4, 5, 6, 7, 8]`.
Expected Output:
Prime numbers count = 4
Q 30. Find the maximum sum of a subarray in [3, -1, -1, 2, 4, -2].
Expected Output:
Maximum sum of subarray = 8
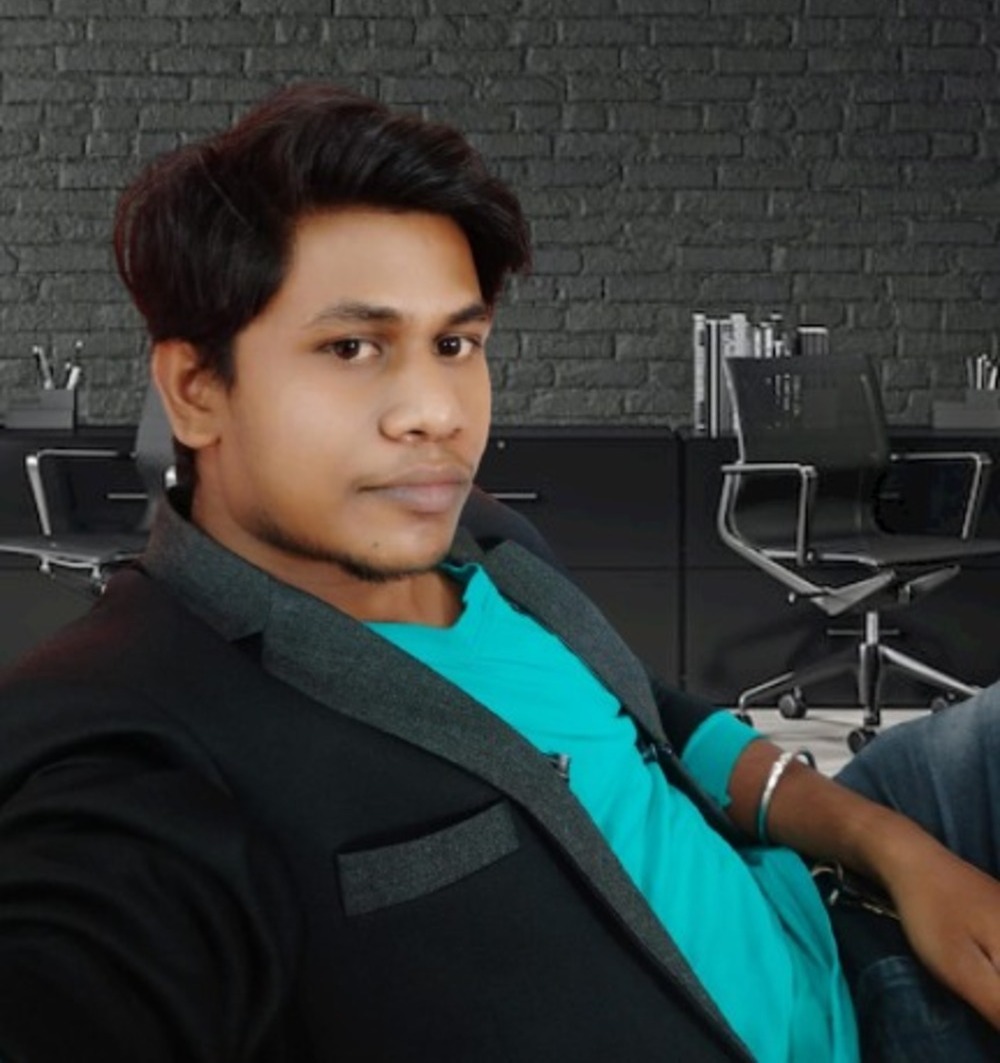
Bikki Singh
Hi, I am the instructor of TechnoVlogs. I have a strong love for programming and enjoy teaching through practical examples. I made this site to help people improve their coding skills by solving real-world problems. With years of experience, my goal is to make learning programming easy and fun for everyone. Let's learn and grow together!