
Best 50 Programming Questions on Variable Type in C
Q 1. Declare an integer variable and print its value.
Expected Output:
Value of a: 10
Q 2. Declare a float variable and print its value.
Expected Output:
Value of b: 5.25
Q 3. Declare a character variable and print its value.
Expected Output:
Value of c: A
Q 4. Declare a double variable and print its value.
Expected Output:
Value of d: 12.345678
Q 5. Declare an integer variable, assign it a value using a mathematical expression, and print its value.
Expected Output:
Value of a: 15
Q 6. Declare a float variable, assign it a value using a division expression, and print its value.
Expected Output:
Value of b: 3.50
Q 7. Declare a character variable and print its ASCII value.
Expected Output:
ASCII value of c: 65
Q 8. Declare an integer variable and print its value in hexadecimal format.
Expected Output:
Hexadecimal value of a: ff
Q 9. Declare a float variable and print its value in exponential notation.
Expected Output:
Exponential notation of b: 1.234560e+02
Q 10. Declare a short integer variable and print its value.
Expected Output:
Value of short integer a: 100
Q 11. Declare a long integer variable and print its value.
Expected Output:
Value of long integer b: 123456789
Q 12. Declare an unsigned integer variable and print its value.
Expected Output:
Value of unsigned integer a: 300
Q 13. Declare a signed char variable and print its value.
Expected Output:
Value of signed char c: -50
Q 14. Declare an unsigned char variable and print its value.
Expected Output:
Value of unsigned char c: 200
Q 15. Declare an integer and a float variable, perform addition, and print the result.
Expected Output:
Result: 8.50
Q 16. Declare a double and a float variable, perform subtraction, and print the result.
Expected Output:
Result: 8.00
Q 17. Declare an integer variable and print its value after incrementing it by 1.
Expected Output:
Value of a after increment: 6
Q 18. Declare an integer variable and print its value after decrementing it by 1.
Expected Output:
Value of a after decrement: 9
Q 19. Declare a constant integer and try to modify its value. Print the error message.
Expected Output:
(If the line is uncommented) error: assignment of read-only variable 'a'
Q 20. Declare an integer variable, assign it a value, and print the address of the variable using the `&` operator.
Expected Output:
Address of a: 0x7ffeedaa6bb4 (Note: The actual address will vary)
Q 21. Declare a pointer to an integer, assign it the address of an integer variable, and print the value of the integer using the pointer.
Expected Output:
Value of a through pointer: 15
Q 22. (Continued) Declare an integer variable and a pointer to it. Modify the value of the integer using the pointer and print the new value.
Expected Output:
New value of a: 30
Q 23. Declare two integer variables, swap their values using a pointer, and print the swapped values.
Expected Output:
Swapped values: a = 10, b = 5
Q 24. Declare a float array of size 5, initialize it, and print its elements.
Expected Output:
arr[0] = 1.1
arr[1] = 2.2
arr[2] = 3.3
arr[3] = 4.4
arr[4] = 5.5
Q 25. Declare a character array (string) and print its elements using a loop.
Expected Output:
str[0] = H
str[1] = e
str[2] = l
str[3] = l
str[4] = o
Q 26. Declare a structure `Point` with two integer members `x` and `y`, and print the values of its members.
Expected Output:
Point p1: x = 10, y = 20
Q 27. Declare an integer, a float, and a character variable in a union, assign values to each, and print the final value stored in the union.
Expected Output:
Final value in union: A
Q 28. Declare an array of integers, pass it to a function, and print its elements in the function.
Expected Output:
arr[0] = 10
arr[1] = 20
arr[2] = 30
Q 29. Declare a static integer variable in a function and demonstrate how its value persists across function calls.
Expected Output:
Count = 1
Count = 2
Count = 3
Q 30. Declare a global integer variable, modify it in a function, and print its value in `main()`.
Expected Output:
Global variable before modification: 5
Global variable after modification: 20
Q 31. Declare an array of characters (string) and find its length without using any built-in functions.
Expected Output:
Length of the string: 13
Q 32. Declare a structure to store a person's details (name, age, salary) and print the details.
Expected Output:
Name: John Doe, Age: 30, Salary: 55000.50
Q 33. Declare a pointer to a structure and use it to access and modify the structure's members.
Expected Output:
Modified Point: x = 30, y = 40
Q 34. Declare a 2D array of integers and print its elements using nested loops.
Expected Output:
arr[0][0] = 1
arr[0][1] = 2
arr[0][2] = 3
arr[1][0] = 4
arr[1][1] = 5
arr[1][2] = 6
Q 35. Declare a constant float variable and try to modify its value. Print the error message.
Expected Output:
(If the line is uncommented) error: assignment of read-only variable 'pi'
Q 36. Declare a volatile integer variable and explain its significance.
Expected Output:
Flag value: 1
Q 37. Demonstrate the use of an `enum` type to define days of the week and print a specific day.
Expected Output:
Today is: 3
Q 38. Declare a macro to square a number and use it in a program.
Expected Output:
Square of 5: 25
Q 39. Declare a bit field structure and demonstrate its use.
Expected Output:
BitField: a = 1, b = 3, c = 7
Q 40. Declare a variable with the `register` keyword and print its value.
Expected Output:
Count: 10
Q 41. Declare an array of pointers to integers and demonstrate its use.
Expected Output:
Value at arr[0]: 1
Value at arr[1]: 2
Value at arr[2]: 3
Q 42. Demonstrate the use of the `sizeof` operator with an integer, a float, and a double variable.
Expected Output:
Size of int: 4
Size of float: 4
Size of double: 8
Q 43. Demonstrate the use of the `typedef` keyword to create an alias for a structure and use it to declare a variable.
Expected Output:
Point: x = 10, y = 20
Q 44. Declare an integer array, use pointer arithmetic to access its elements, and print them.
Expected Output:
Element 0: 10
Element 1: 20
Element 2: 30
Q 45. Declare a variable in a header file, include the header in a program, and print the variable's value.
Expected Output:
Value of myVar: 10
Q 46. Use a preprocessor directive to include a system header file and demonstrate its use.
Expected Output:
Hello, World!
Q 47. Demonstrate the use of command-line arguments to pass and print an integer value.
Expected Output:
(When run with a command-line argument like `5`)
You entered: 5
Q 48. Demonstrate how to compile a program with multiple source files.
Expected Output:
(After compiling with `gcc main.c file1.c -o program`)
Hello from file1!
Q 49. Write a simple program to demonstrate the use of `malloc` and `free` for dynamic memory allocation.
Expected Output:
Value at ptr[0]: 1
Value at ptr[1]: 2
Value at ptr[2]: 3
Q 50. Write a program that reads an integer from the user, allocates memory dynamically for an array of that size, and fills it with random numbers. Print the array and free the memory.
Expected Output:
(Example output for an input size of 5)
Random array: 45 23 67 89 12
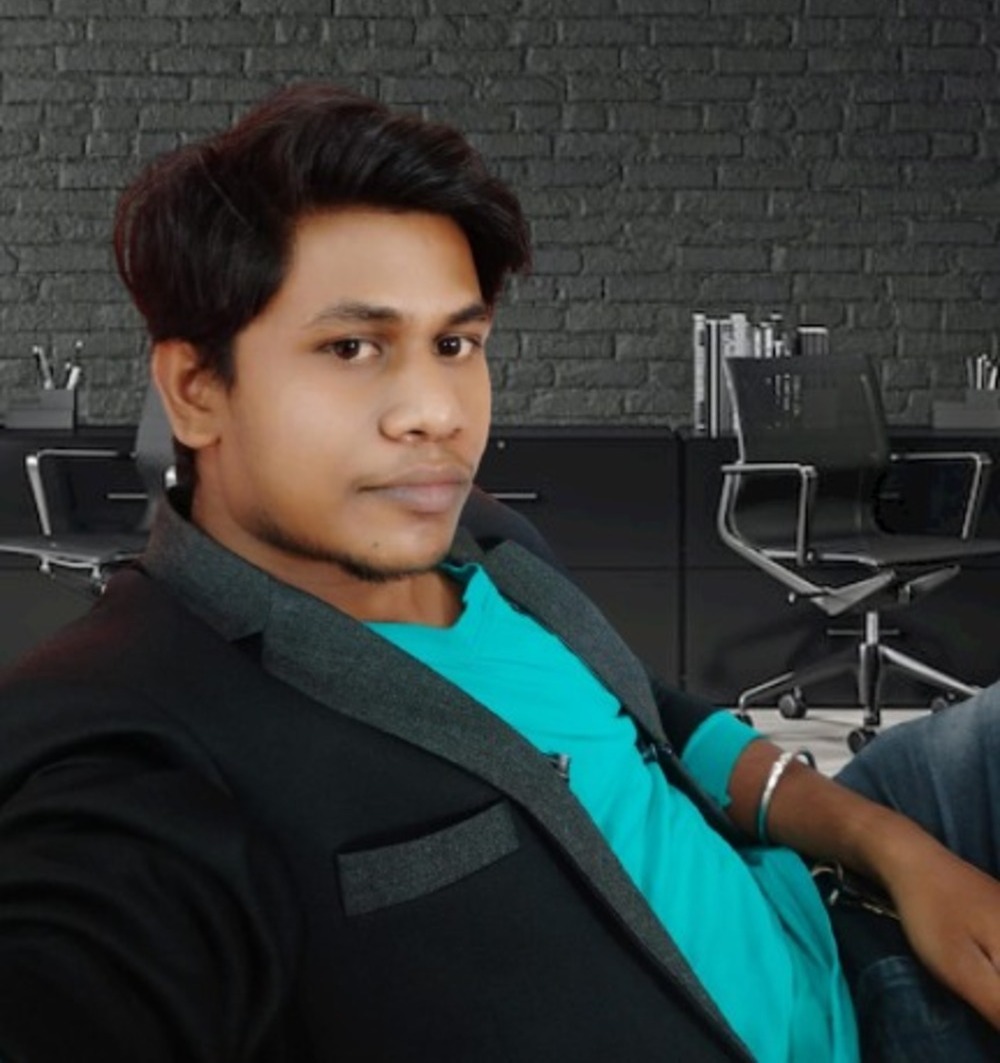
Bikki Singh
Hi, I am the instructor of TechnoVlogs. I have a strong love for programming and enjoy teaching through practical examples. I made this site to help people improve their coding skills by solving real-world problems. With years of experience, my goal is to make learning programming easy and fun for everyone. Let's learn and grow together!