
Practice Top 30 C Programming Questions on Recursion
Q1. Write a recursive function to calculate the factorial of a number.
Input: 5
Output: 120
Q2. Write a recursive function to calculate the sum of the first N natural numbers.
Input: 10
Output: 55
Q3. Write a recursive function to print the first N Fibonacci numbers.
Input: 5
Output: 0 1 1 2 3
Q4. Write a recursive function to find the greatest common divisor (GCD) of two numbers.
Input: 36, 60
Output: 12
Q5. Write a recursive function to calculate \( a^b \) (a raised to the power b).
Input: 2, 3
Output: 8
Q6. Write a recursive function to reverse a string.
Input: "hello"
Output: olleh
Q7. Write a recursive function to check if a string is a palindrome.
Input: "madam"
Output: Palindrome
Q8. Write a recursive function to calculate the sum of the digits of a number.
Input: 1234
Output: 10
Q9. Write a recursive function to find the maximum element in an array.
Input: 1, 7, 3, 9, 5
Output: 9
Q10. Implement a recursive binary search function to find an element in a sorted array.
Input: Sorted array: [1, 3, 5, 7, 9], Target: 7
Output: Element found at index 3
Q11. Write a recursive function to reverse an array.
Input: [1, 2, 3, 4, 5]
Output: [5, 4, 3, 2, 1]
Q12. Write a recursive function to count the number of digits in a number.
Input: 4567
Output: 4
Q13. Write a recursive function to check if a number is even or odd.
Input: 7
Output: Odd
Q14. Write a recursive function to calculate the product of all elements in an array.
Input: [2, 3, 4]
Output: 24
Q15. Write a recursive function to find the least common multiple (LCM) of two numbers.
Input: 4, 6
Output: 12
Q16. Write a recursive function to print numbers from 1 to N.
Input: 5
Output: 1 2 3 4 5
Q17. Write a recursive function to print numbers from N to 1.
Input: 5
Output: 5 4 3 2 1
Q18. Write a recursive function to count the number of vowels in a string.
Input: "education"
Output: 5
Q19. Write a recursive function to check if a number is prime.
Input: 7
Output: Prime
Q20. Write a recursive function to check if a number is an Armstrong number.
Input: 153
Output: Armstrong
Q21. Write a recursive function to find the smallest element in an array.
Input: [6, 3, 8, 2]
Output: 2
Q22. Write a recursive function to calculate the sum of even numbers up to N.
Input: 10
Output: 30
Q23. Write a recursive function to calculate the sum of odd numbers up to N.
Input: 9
Output: 25
Q24. Write a recursive function to print all factors of a number.
Input: 12
Output: 1 2 3 4 6 12
Q25. Write a recursive function to calculate \( 2^n \).
Input: 4
Output: 16
Q26. Write a recursive function to calculate the sum of elements in an array.
Input: [1, 2, 3, 4, 5]
Output: 15
Q27. Write a recursive function to find the nth Fibonacci number.
Input: 6
Output: 8
Q28. Write a recursive function to calculate \( nCr \) (combination).
Input: n=5, r=2
Output: 10
Q29. Write a recursive function to generate Pascal’s Triangle for N rows.
Input: 4
Output:
1
1 1
1 2 1
1 3 3 1
Q30. Write a recursive function to calculate the product of the digits of a number.
Input: 123
Output: 6
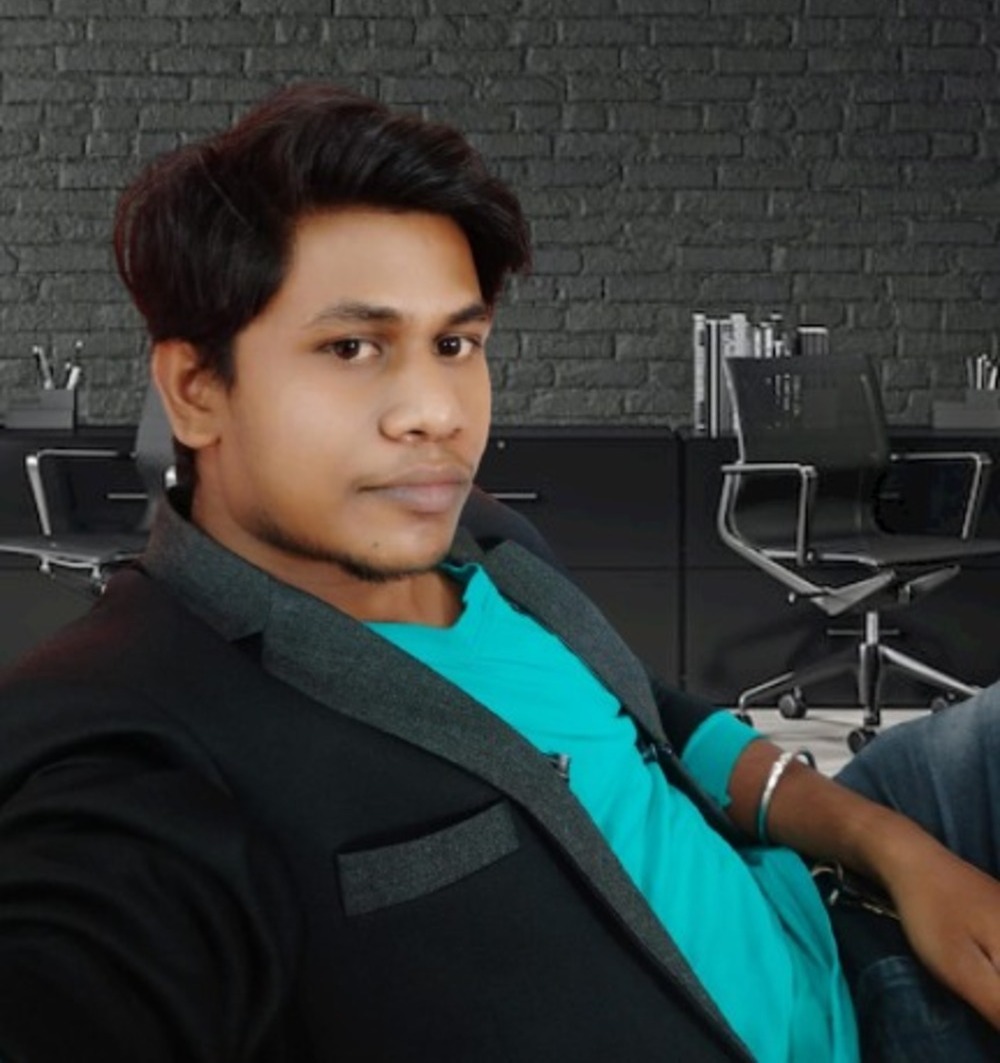
Bikki Singh
Hi, I am the instructor of TechnoVlogs. I have a strong love for programming and enjoy teaching through practical examples. I made this site to help people improve their coding skills by solving real-world problems. With years of experience, my goal is to make learning programming easy and fun for everyone. Let's learn and grow together!