
Practice Top 30 C++ Programming Questions on Vector
Q1. Write a program to initialize a vector with five integers and display its elements.
Input: None
Output: Vector: [1, 2, 3, 4, 5]
Q2. Write a program to add integers to a vector and display its elements.
Input: 10 20 30
Output: Vector: [10, 20, 30]
Q3. Write a program to access the first and last elements of a vector.
Input: Vector: [5, 10, 15]
Output: First: 5, Last: 15
Q4. Write a program to display the size of a vector.
Input: Vector: [7, 8, 9]
Output: Size: 3
Q5. Write a program to iterate through a vector using a for loop.
Input: Vector: [1, 2, 3, 4]
Output: 1 2 3 4
Q6. Write a program to clear all elements from a vector and display its size.
Input: Vector: [10, 20, 30]
Output: Size after clear: 0
Q7. Write a program to check if a vector is empty.
Input: Vector: []
Output: Vector is empty.
Q8. Write a program to modify specific elements in a vector.
Input: Vector: [1, 2, 3] (Change 2 to 20)
Output: Modified Vector: [1, 20, 3]
Q9. Write a program to insert an element at a specific position in a vector.
Input: Vector: [1, 3, 4], Insert 2 at position 1
Output: Vector: [1, 2, 3, 4]
Q10. Write a program to erase an element from a vector.
Input: Vector: [10, 20, 30], Erase element at position 1
Output: Vector: [10, 30]
Q11. Write a program to find the maximum element in a vector.
Input: Vector: [2, 5, 1, 7]
Output: Max: 7
Q12. Write a program to find the minimum element in a vector.
Input: Vector: [3, 9, 4]
Output: Min: 3
Q13. Write a program to reverse the elements of a vector.
Input: Vector: [1, 2, 3, 4]
Output: Reversed Vector: [4, 3, 2, 1]
Q14. Write a program to sort the elements of a vector in ascending order.
Input: Vector: [9, 4, 7, 3]
Output: Sorted Vector: [3, 4, 7, 9]
Q15. Write a program to sort the elements of a vector in descending order.
Input: Vector: [2, 5, 1]
Output: Sorted Vector: [5, 2, 1]
Q16. Write a program to find the sum of all elements in a vector.
Input: Vector: [2, 4, 6]
Output: Sum: 12
Q17. Write a program to check if a specific element exists in a vector.
Input: Vector: [1, 2, 3], Search: 2
Output: Element found.
Q18. Write a program to remove duplicate elements from a vector.
Input: Vector: [1, 2, 2, 3]
Output: Vector: [1, 2, 3]
Q19. Write a program to concatenate two vectors.
Input: Vectors: [1, 2], [3, 4]
Output: Concatenated Vector: [1, 2, 3, 4]
Q20. Write a program to replace all occurrences of a specific value in a vector.
Input: Vector: [1, 2, 2, 3], Replace 2 with 5
Output: Vector: [1, 5, 5, 3]
Q21. Write a program to remove the last element from a vector.
Input: Vector: [4, 5, 6]
Output: Vector: [4, 5]
Q22. Write a program to resize a vector to a given size.
Input: Vector: [1, 2, 3], Resize to 5
Output: Vector: [1, 2, 3, 0, 0]
Q23. Write a program to store and display a vector of strings.
Input: ["apple", "banana", "cherry"]
Output: apple banana cherry
Q24. Write a program to create and display a 2D vector.
Input: [[1, 2], [3, 4]]
Output: 1 2\n3 4
Q25. Write a program to sort a vector in custom order using a comparator.
Input: Vector: [10, 5, 20] (Sort by descending order)
Output: Vector: [20, 10, 5]
Q26. Write a program to copy elements of one vector to another.
Input: Vector: [1, 2, 3]
Output: Copied Vector: [1, 2, 3]
Q27. Write a program to find the intersection of two vectors.
Input: [1, 2, 3] and [2, 3, 4]
Output: Intersection: [2, 3]
Q28. Write a program to erase a range of elements from a vector.
Input: Vector: [1, 2, 3, 4], Range: 1 to 3
Output: Vector: [1, 4]
Q29. Write a program to count the occurrences of a specific element in a vector.
Input: Vector: [1, 2, 2, 3], Count 2
Output: Occurrences: 2
Q30. Write a program to generate a vector of n elements with values from 1 to n.
Input: n = 5
Output: Vector: [1, 2, 3, 4, 5]
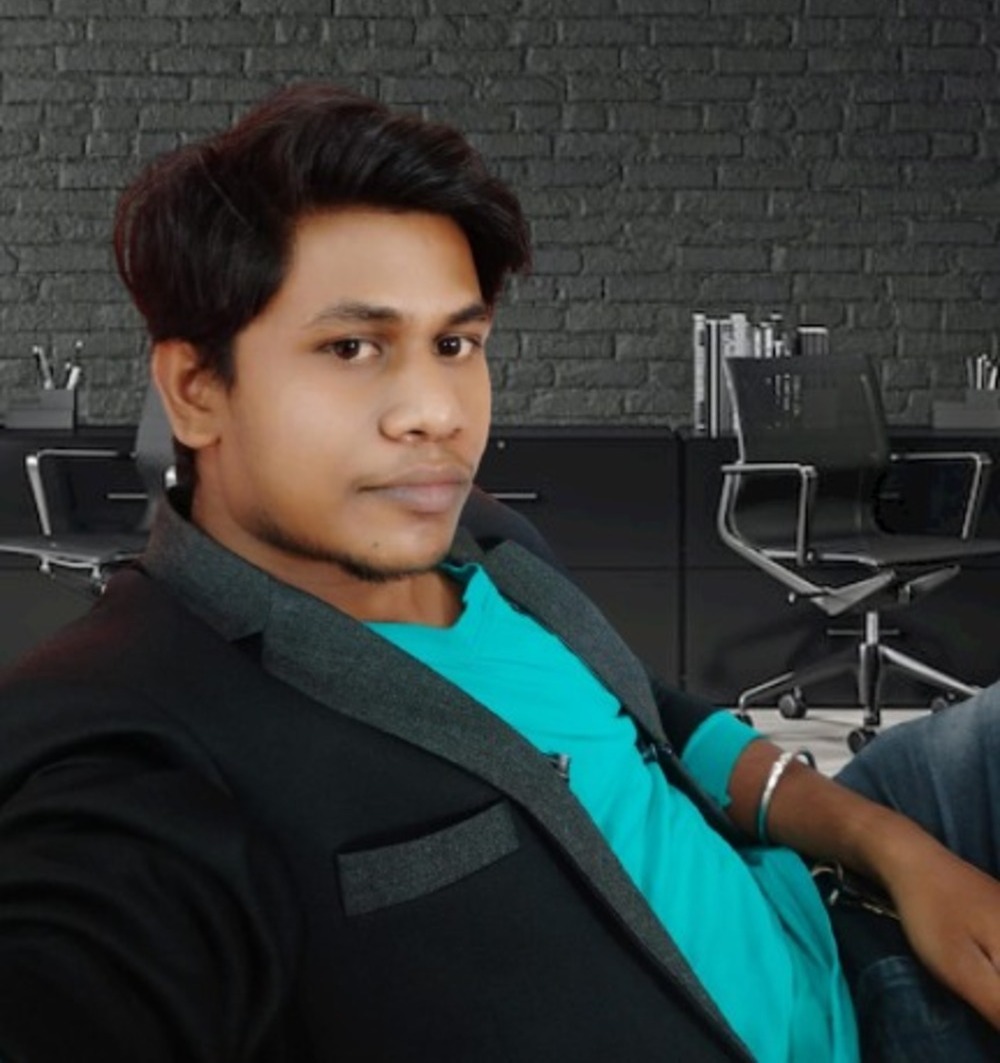
Bikki Singh
Hi, I am the instructor of TechnoVlogs. I have a strong love for programming and enjoy teaching through practical examples. I made this site to help people improve their coding skills by solving real-world problems. With years of experience, my goal is to make learning programming easy and fun for everyone. Let's learn and grow together!