
Practice Top 30 C++ Programming Questions on Stack
Q1. Perform push and pop operations on a stack.
Input:
Push: 10, 20, 30
Pop: 1
Expected Output:
Stack after operations: 20 -> 10
Q2. Get the top element of the stack without removing it.
Input:
Stack: 10 -> 20 -> 30
Expected Output:
Top Element: 30
Q3. Check whether the stack is empty.
Input:
Stack: Empty
Expected Output:
Is Empty: Yes
Q4. Find the number of elements in the stack.
Input:
Stack: 10 -> 20 -> 30
Expected Output:
Size: 3
Q5. Reverse the elements of a stack.
Input:
Stack: 10 -> 20 -> 30
Expected Output:
Reversed Stack: 30 -> 20 -> 10
Q6. Sort the elements of a stack in ascending order.
Input:
Stack: 30 -> 10 -> 20
Expected Output:
Sorted Stack: 10 -> 20 -> 30
Q7. Implement two stacks using a single array.
Input:
Operations: Push(1) to Stack1, Push(2) to Stack2
Expected Output:
Stack1: 1
Stack2: 2
Q8. Evaluate a postfix expression using a stack.
Input:
Expression: 5 3 + 8
Expected Output:
Result: 64
Q9. Check if an expression has balanced parentheses.
Input:
Expression: (a+b) (c-d)
Expected Output:
Balanced: Yes
Q10. Create a stack that supports retrieving the minimum element in constant time.
Input:
Operations: Push(10), Push(5), Push(8), GetMin()
Expected Output:
Minimum Element: 5
Q11. Convert an infix expression to postfix using a stack.
Input:
Expression: (a+b)(c-d)
Expected Output:
Postfix: ab+cd-
Q12. Check if a string is a palindrome using a stack.
Input:
String: racecar
Expected Output:
Is Palindrome: Yes
Q13. Simulate a stack using two queues.
Input:
Operations: Push(10), Push(20), Pop()
Expected Output:
Top Element: 10
Q14. Find the nearest greater element to the right for each element in an array using a stack.
Input:
Array: [4, 5, 2, 10, 8]
Expected Output:
Result: [5, 10, 10, -1, -1]
Q15. Find the span of stock prices for a given array.
Input:
Prices: [100, 80, 60, 70, 60, 75, 85]
Expected Output:
Span: [1, 1, 1, 2, 1, 4, 6]
Q16. Simulate stack overflow and underflow conditions.
Input:
Stack Size: 3
Push: 10, 20, 30, 40
Expected Output:
Overflow: Cannot push 40
Q17. Reverse a string using a stack.
Input:
String: Hello
Expected Output:
Reversed String: olleH
Q18. Convert a decimal number to binary using a stack.
Input:
Number: 10
Expected Output:
Binary: 1010
Q19. Sort a stack recursively without using extra space.
Input:
Stack: 30 -> 10 -> 20
Expected Output:
Sorted Stack: 10 -> 20 -> 30
Q20. Find the next smaller element for each element in an array using a stack.
Input:
Array: [4, 5, 2, 10, 8]
Expected Output:
Result: [2, 2, -1, 8, -1]
Q21. Implement k stacks using a single array.
Input:
Operations: Push(10, Stack1), Push(20, Stack2), Push(30, Stack3)
Expected Output:
Stack1: 10
Stack2: 20
Stack3: 30
Q22. Implement undo and redo functionality using stacks.
Input:
Operations: Write("Hello"), Write("World"), Undo(), Redo()
Expected Output:
After Undo: Hello
After Redo: World
Q23. Find the maximal rectangle area in a histogram using a stack.
Input:
Histogram: [2, 1, 5, 6, 2, 3]
Expected Output:
Max Area: 10
Q24. For each element in an array, find the previous greater element using a stack.
Input:
Array: [4, 5, 2, 10, 8]
Expected Output:
Result: [-1, 5, 5, -1, 10]
Q25. Check if a given expression has redundant parentheses using a stack.
Input:
Expression: ((a+b))
Expected Output:
Redundant: Yes
Q26. Evaluate an infix expression using stacks.
Input:
Expression: 3 + 5 (2 - 8)
Expected Output:
Result: -13
Q27. Remove adjacent duplicates from a string using a stack.
Input:
String: "aabbcc"
Expected Output:
After Removal: ""
Q28. Find the next greater element for each element in an array using a stack.
Input:
Array: [4, 5, 2, 10, 8]
Expected Output:
Result: [5, 10, 10, -1, -1]
Q29. Check if a given expression is balanced in terms of brackets.
Input:
Expression: (a+b) {c/d}
Expected Output:
Balanced: Yes
Q30. Find the maximum element in a stack using additional operations.
Input:
Operations: Push(10), Push(20), Push(15), GetMax()
Expected Output:
Maximum Element: 20
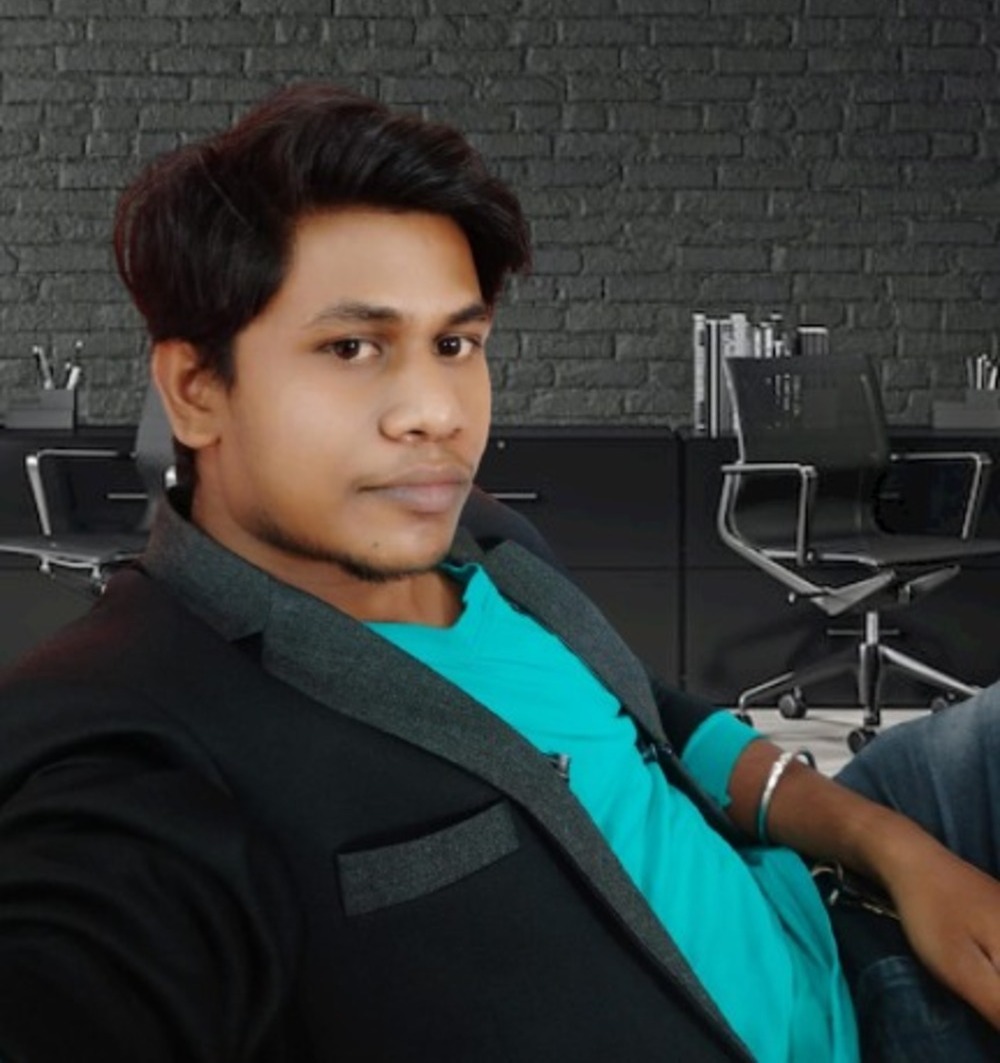
Bikki Singh
Hi, I am the instructor of TechnoVlogs. I have a strong love for programming and enjoy teaching through practical examples. I made this site to help people improve their coding skills by solving real-world problems. With years of experience, my goal is to make learning programming easy and fun for everyone. Let's learn and grow together!