
Practice Top 30 C++ Programming Questions on Object-Orineted Programming
Q1. Write a class Student with attributes name and age. Create an object of the class and display its attributes.
Input:
cpp
name = "Alice"
age = 20
Expected Output:
Name: Alice
Age: 20
Q2. Write a class Circle with a constructor that accepts the radius and calculates the area.
Input:
cpp
radius = 5
Expected Output:
Area: 78.54
Q3. Create a class Car with a default constructor initializing model as "Sedan" and speed as 120.
Expected Output:
Model: Sedan
Speed: 120
Q4. Write a class Rectangle with a parameterized constructor that calculates the area.
Input:
cpp
length = 5, width = 4
Expected Output:
Area: 20
Q5. Write a class BankAccount with private members balance and public methods to deposit, withdraw, and check the balance.
Input:
cpp
Deposit 1000
Withdraw 500
Expected Output:
Balance: 500
Q6. Create a base class Shape with a method area() and derive a class Square to override area().
Input:
cpp
side = 4
Expected Output:
Area of Square: 16
Q7. Write a base class Animal with a virtual method sound(). Derive classes Dog and Cat that override sound().
Expected Output:
Dog: Bark
Cat: Meow
Q8. Write a class Box and a friend function to access its private members.
Input:
cpp
Length = 5, Width = 3
Expected Output:
Volume: 15
Q9. Create a class Counter with a static variable to count the number of objects created.
Expected Output:
Objects Created: 3
Q10. Write a class Book with a copy constructor that duplicates the attributes of one object into another.
Expected Output:
Original Title: C++ Basics
Copied Title: C++ Basics
Q11. Create a class Logger that logs a message when an object is destroyed.
Expected Output:
Object Created
Object Destroyed
Q12. Write a class Person with private members name and age. Provide getter and setter methods to access and modify these members.
Input:
cpp
Set Name: John, Age: 30
Get Name and Age
Expected Output:
Name: John
Age: 30
Q13. Create two base classes A and B. Derive a class C that inherits both and demonstrate the use of inherited methods.
Expected Output:
Class A Method
Class B Method
Q14. Create a base class Employee with a derived class Manager and another derived class Engineer. Display details of both using a common function.
Expected Output:
Manager: Alice
Engineer: Bob
Q15. Write a class Test with members under public, private, and protected. Demonstrate access using inheritance.
Expected Output:
Public Member Accessible
Protected Member Accessible
Private Member Not Accessible
Q16. Overload the + operator for a class Complex to add two complex numbers.
Input:
cpp
Complex1: 3 + 4i
Complex2: 1 + 2i
Expected Output:
Sum: 4 + 6i
Q17. Write a class A and make another class B its friend. Allow B to access private members of A.
Expected Output:
Class B accessing Class A's private member
Q18. Create an abstract class Shape with a pure virtual method draw(). Derive Circle and Rectangle to implement draw().
Expected Output:
Drawing Circle
Drawing Rectangle
Q19. Write a class Math with overloaded methods add(int, int) and add(double, double).
Input:
cpp
add(3, 4)
add(2.5, 1.5)
Expected Output:
Integer Addition: 7
Double Addition: 4.0
Q20. Create a class Point with overloaded constructors for different initializations.
Input:
cpp
Constructor 1: x = 0, y = 0
Constructor 2: x = 5, y = 7
Expected Output:
Point 1: (0, 0)
Point 2: (5, 7)
Q21. Write a class Outer that contains a nested class Inner. Show how to create and use objects of the nested class.
Expected Output:
Outer Class Method
Inner Class Method
Q22. Write a class Array to allocate memory dynamically for an integer array.
Input:
cpp
Array Size = 5
Array Elements = 1, 2, 3, 4, 5
Expected Output:
Sum of Elements: 15
Q23. Demonstrate the use of a virtual destructor with base and derived classes.
Expected Output:
Base Destructor Called
Derived Destructor Called
Q24. Create a template class Box that works with both integers and doubles.
Input:
cpp
Box<int>: 10
Box<double>: 15.5
Expected Output:
Integer Box: 10
Double Box: 15.5
Q25. Write a program to create an array of Student objects and display their details.
Input:
cpp
Student 1: Alice, 20
Student 2: Bob, 22
Expected Output:
Alice, 20
Bob, 22
Q26. Create a class Math with a static method square(int).
Input:
cpp
Value = 5
Expected Output:
Square: 25
Q27. Write a class Item and use a pointer to an object to access its methods.
Expected Output:
Item Name: Laptop
Price: 50000
Q28. Write a base class Vehicle with a method start(). Override it in a derived class Bike.
Expected Output:
Vehicle Starting
Bike Starting
Q29. Write a class TrafficLight with an enum for Red, Yellow, and Green.
Expected Output:
Light: Red
Q30. Create a class Author and another class Book. Use aggregation to display book details along with the author's name.
Input:
cpp
Author: Alice
Book: C++ Basics
Expected Output:
Book: C++ Basics
Author: Alice
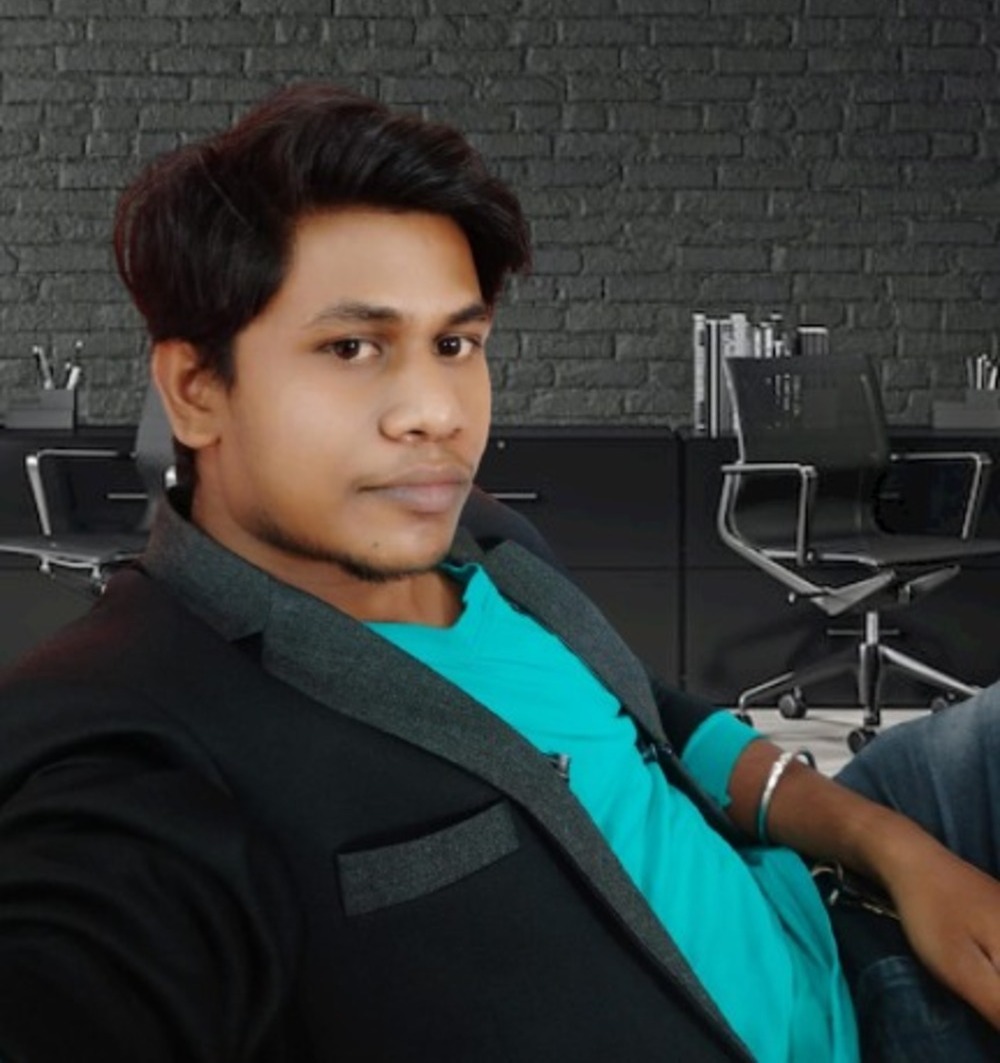
Bikki Singh
Hi, I am the instructor of TechnoVlogs. I have a strong love for programming and enjoy teaching through practical examples. I made this site to help people improve their coding skills by solving real-world problems. With years of experience, my goal is to make learning programming easy and fun for everyone. Let's learn and grow together!