
30 Basic Programming Questions in C++ on While loops
Q 1. Write a program to print numbers from 1 to N using a while loop.
Input: 5
Expected Output: 1 2 3 4 5
Q 2. Write a program to find the sum of numbers from 1 to N using a while loop.
Input: 5
Expected Output: 15
Q 3. Write a program to print all even numbers between 1 and N using a while loop.
Input: 10
Expected Output: 2 4 6 8 10
Q 4. Write a program to calculate the sum of all even numbers between 1 and N using a while loop.
Input: 6
Expected Output: 12
Q 5. Write a program to reverse the digits of a given number using a while loop.
Input: 12345
Expected Output: 54321
Q 6. Write a program to count the number of digits in a given number using a while loop.
Input: 12345
Expected Output: 5
Q 7. Write a program to check if a number is a palindrome using a while loop.
Input: 121
Expected Output: Palindrome
Q 8. Write a program to print the multiplication table of a given number using a while loop.
Input: 3
Expected Output:
3 x 1 = 3
3 x 2 = 6
3 x 3 = 9
...
3 x 10 = 30
Q 9. Write a program to calculate the sum of digits of a number using a while loop.
Input: 1234
Expected Output: 10
Q 10. Write a program to calculate the factorial of a number using a while loop.
Input: 5
Expected Output: 120
Q 11. Write a program to print all odd numbers between 1 and N using a while loop.
Input: 10
Expected Output: 1 3 5 7 9
Q 12. Write a program to check if a number is prime using a while loop.
Input: 7
Expected Output: Prime
Q 13. Write a program to find the greatest common divisor (GCD) of two numbers using a while loop.
Input: 18, 24
Expected Output: 6
Q 14. Write a program to find the least common multiple (LCM) of two numbers using a while loop.
Input: 4, 5
Expected Output: 20
Q 15. Write a program to find the power of a number using a while loop.
Input: 2 3
Expected Output: 8
Q 16. Write a program to print the Fibonacci series up to N terms using a while loop.
Input: 5
Expected Output: 0 1 1 2 3
Q 17. Write a program to calculate the sum of odd numbers between 1 and N using a while loop.
Input: 10
Expected Output: 25
Q 18. Write a program to count the number of even numbers between 1 and N using a while loop.
Input: 10
Expected Output: 5
Q 19. Write a program to find the largest digit in a given number using a while loop.
Input: 5729
Expected Output: 9
Q 20. Write a program to find the smallest digit in a number using a while loop.
Input: 5729
Expected Output: 2
Q 21. Write a program to count how many prime numbers are between 1 and N using a while loop.
Input: 10
Expected Output: 4 (Prime numbers are 2, 3, 5, 7)
Q 22. Write a program to print powers of 2 (2^0, 2^1, 2^2, ...) until the result is less than or equal to N using a while loop.
Input: 32
Expected Output: 1 2 4 8 16 32
Q 23. Write a program to check if a number is an Armstrong number (e.g., 153 = 1^3 + 5^3 + 3^3) using a while loop.
Input: 153
Expected Output: Armstrong Number
Q 24. Write a program to calculate the sum of squares of digits of a number using a while loop.
Input: 123
Expected Output: 14
Q 25. Write a program to check if a number is a perfect number (sum of divisors equals the number) using a while loop.
Input: 6
Expected Output: Perfect Number
Q 26. Write a program to calculate the sum of the series 1 + 1/2 + 1/3 + ... + 1/N using a while loop.
Input: 5
Expected Output: 2.28333
Q 27. Write a program to print the first N Fibonacci numbers using a while loop.
Input: 7
Expected Output: 0 1 1 2 3 5 8
Q 28. Write a recursive function to find the factorial of a number using a while loop.
Input: 5
Expected Output: 120
Q 29. Write a program to print all prime numbers between two given numbers using a while loop.
Input: 10 20
Expected Output: 11 13 17 19
Q 30. Write a program to find the sum of the first N natural numbers using recursion with a while loop.
Input: 5
Expected Output: 15
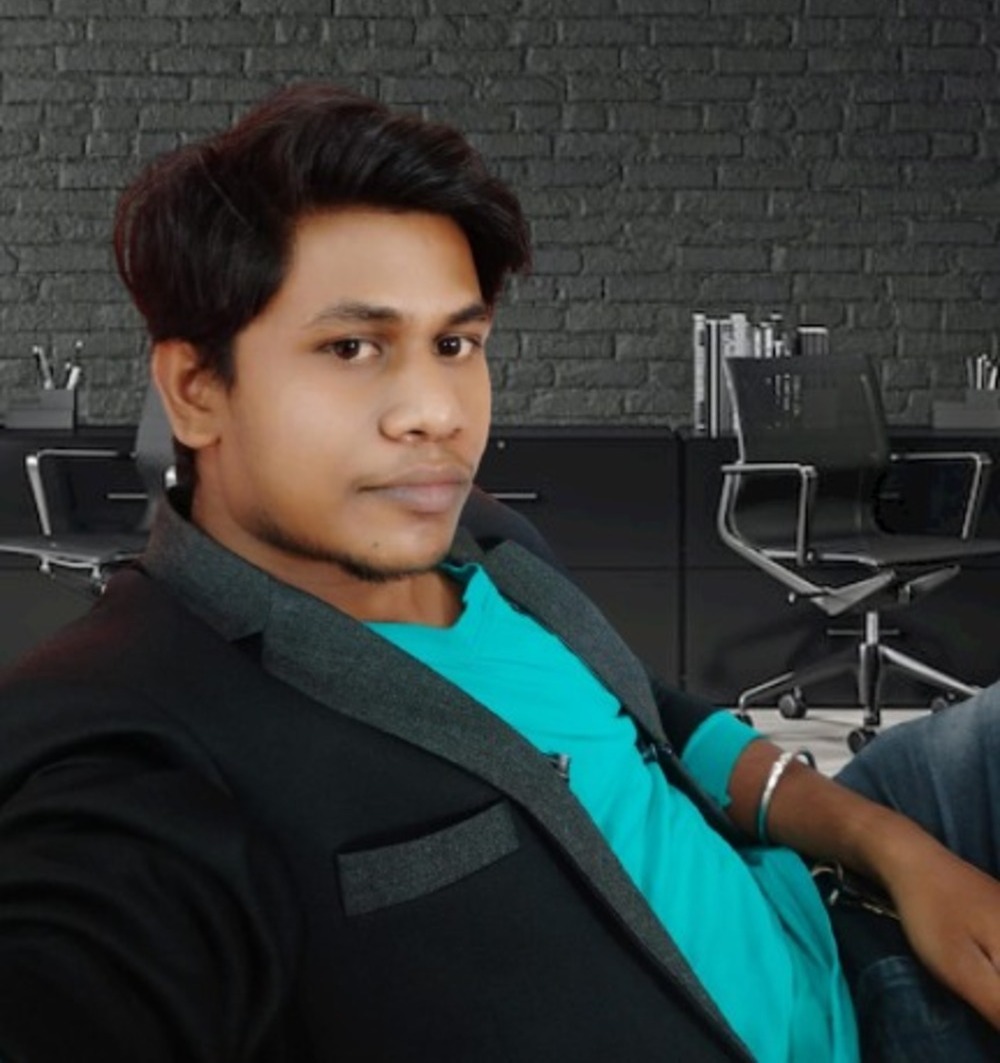
Bikki Singh
Hi, I am the instructor of TechnoVlogs. I have a strong love for programming and enjoy teaching through practical examples. I made this site to help people improve their coding skills by solving real-world problems. With years of experience, my goal is to make learning programming easy and fun for everyone. Let's learn and grow together!