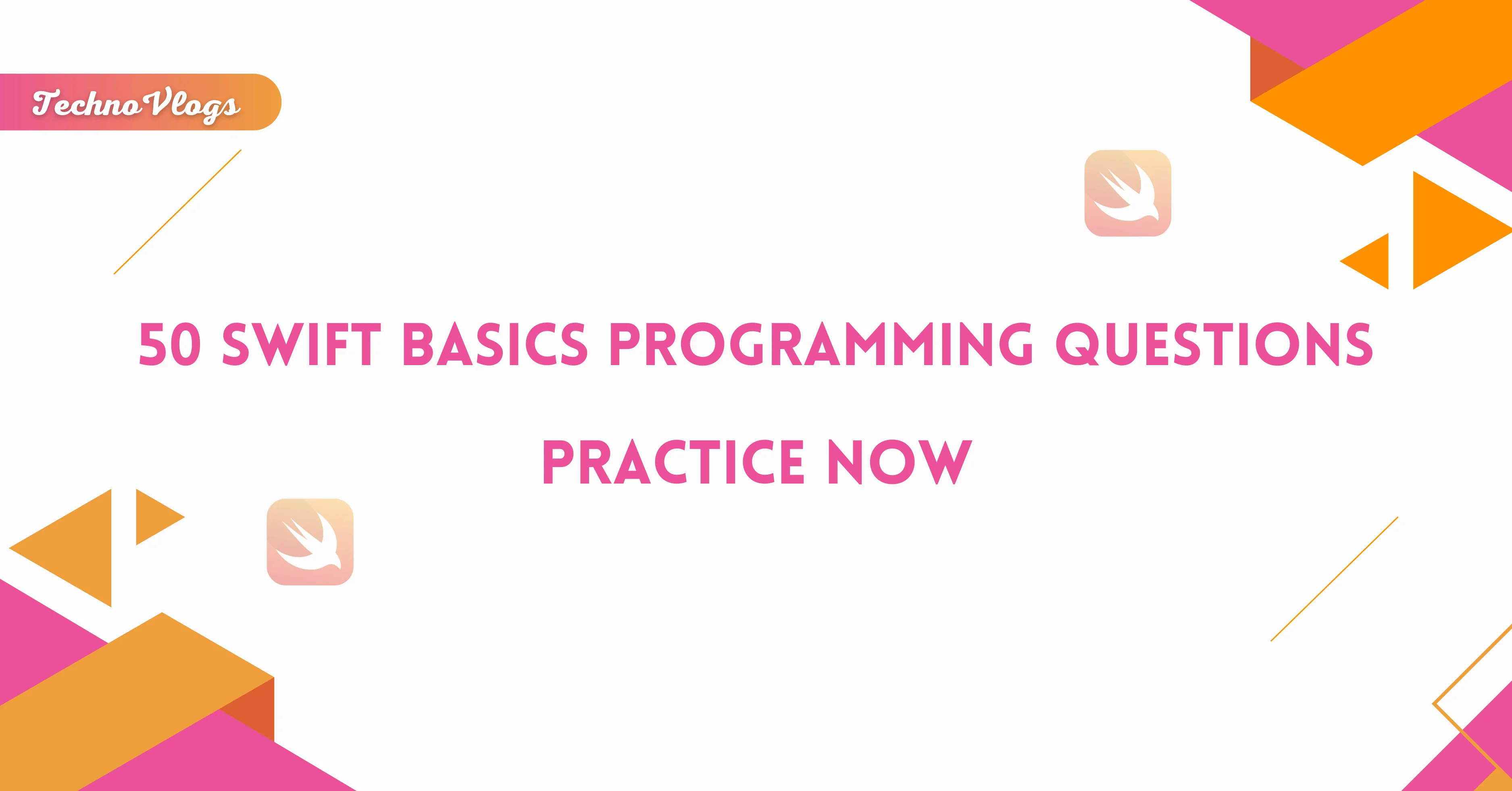
Practice 50 Swift Basics Programming Questions
Q1. Write a Swift program to print "Hello, World!" to the console.
Expected Output:
Hello, World!
Q2. Write a Swift program to declare a constant and print its value.
Input:
let x = 10
Expected Output:
10
Q3. Write a Swift program to declare a variable and modify its value.
Input:
var y = 5
y = 10
Expected Output:
10
Q4. Write a Swift program to calculate the sum of two integers and print the result.
Input:
let a = 5, b = 7
Expected Output:
12
Q5. Write a Swift program to find the product of two numbers.
Input:
let a = 3, b = 4
Expected Output:
12
Q6. Write a Swift program to check if a number is positive, negative, or zero.
Input:
let num = -5
Expected Output:
Negative
Q7. Write a Swift program to perform basic arithmetic operations (addition, subtraction, multiplication, division).
Input:
let a = 8, b = 4
Expected Output:
Addition: 12
Subtraction: 4
Multiplication: 32
Division: 2.0
Q8. Write a Swift program to calculate the area of a circle given its radius.
Input:
let radius = 5
Expected Output:
Area: 78.54
Q9. Write a Swift program to check if a number is even or odd.
Input:
let num = 6
Expected Output:
Even
Q10. Write a Swift program to find the maximum of two numbers.
Input:
let a = 5, b = 10
Expected Output:
10
Q11. Write a Swift program to find the minimum of two numbers.
Input:
let a = 5, b = 10
Expected Output:
5
Q12. Write a Swift program to check if a number is divisible by 3 and 5.
Input:
let num = 15
Expected Output:
Divisible by 3 and 5
Q13. Write a Swift program to print the Fibonacci series up to the 10th term.
Expected Output:
0, 1, 1, 2, 3, 5, 8, 13, 21, 34
Q14. Write a Swift program to print the first 10 multiples of a number.
Input:
let num = 3
Expected Output:
3, 6, 9, 12, 15, 18, 21, 24, 27, 30
Q15. Write a Swift program to reverse a string.
Input:
let str = "Swift"
Expected Output:
tfiwS
Q16. Write a Swift program to check if a string is a palindrome.
Input:
let str = "madam"
Expected Output:
Palindrome
Q17. Write a Swift program to count the number of vowels in a string.
Input:
let str = "Hello, World!"
Expected Output:
3
Q18. Write a Swift program to find the length of a string.
Input:
let str = "Hello"
Expected Output:
5
Q19. Write a Swift program to convert a string to uppercase.
Input:
let str = "hello"
Expected Output:
HELLO
Q20. Write a Swift program to convert a string to lowercase.
Input:
let str = "HELLO"
Expected Output:
hello
Q21. Write a Swift program to concatenate two strings.
Input:
let str1 = "Hello", str2 = "World"
Expected Output:
HelloWorld
Q22. Write a Swift program to check if a character exists in a string.
Input:
let str = "Hello", char = "e"
Expected Output:
Character exists
Q23. Write a Swift program to remove whitespace from a string.
Input:
let str = " Hello World "
Expected Output:
Hello World
Q24. Write a Swift program to find the index of a character in a string.
Input:
let str = "Hello", char = "e"
Expected Output:
1
Q25. Write a Swift program to split a string into an array of words.
Input:
let str = "Swift programming"
Expected Output:
["Swift", "programming"]
Q26. Write a Swift program to find the sum of an array of integers.
Input:
let arr = [1, 2, 3, 4]
Expected Output:
10
Q27. Write a Swift program to find the average of an array of integers.
Input:
let arr = [1, 2, 3, 4]
Expected Output:
2.5
Q28. Write a Swift program to sort an array of integers in ascending order.
Input:
let arr = [4, 1, 3, 2]
Expected Output:
[1, 2, 3, 4]
Q29. Write a Swift program to check if an array contains a specific number.
Input:
let arr = [1, 2, 3, 4], num = 3
Expected Output:
Array contains the number
Q30. Write a Swift program to find the largest number in an array.
Input:
let arr = [1, 2, 3, 4]
Expected Output:
4
Q31. Write a Swift program to find the smallest number in an array.
Input:
let arr = [1, 2, 3, 4]
Expected Output:
1
Q32. Write a Swift program to remove duplicates from an array.
Input:
let arr = [1, 2, 2, 3]
Expected Output:
[1, 2, 3]
Q33. Write a Swift program to join an array of strings into a single string.
Input:
let arr = ["Hello", "World"]
Expected Output:
Hello World
Q34. Write a Swift program to check if an array is empty.
Input:
let arr = [1, 2, 3]
Expected Output:
Not empty
Q35. Write a Swift program to count the number of elements in an array.
Input:
let arr = [1, 2, 3, 4]
Expected Output:
4
Q36. Write a Swift program to add an element to the end of an array.
Input:
let arr = [1, 2, 3], num = 4
Expected Output:
[1, 2, 3, 4]
Q37. Write a Swift program to find the index of an element in an array.
Input:
let arr = [1, 2, 3, 4], num = 3
Expected Output:
2
Q38. Write a Swift program to find the first element in an array.
Input:
let arr = [1, 2, 3, 4]
Expected Output:
1
Q39. Write a Swift program to reverse an array.
Input:
let arr = [1, 2, 3, 4]
Expected Output:
[4, 3, 2, 1]
Q40. Write a Swift program to find the middle element of an array.
Input:
let arr = [1, 2, 3, 4, 5]
Expected Output:
3
Q41. Write a Swift program to create and print a dictionary.
Input:
let dict = ["name": "John", "age": "30"]
Expected Output:
["name": "John", "age": "30"]
Q42. Write a Swift program to add a key-value pair to a dictionary.
Input:
let dict = ["name": "John"], newKey = "age", newValue = "30"
Expected Output:
["name": "John", "age": "30"]
Q43. Write a Swift program to remove a key-value pair from a dictionary.
Input:
let dict = ["name": "John", "age": "30"], key = "age"
Expected Output:
["name": "John"]
Q44. Write a Swift program to check if a dictionary contains a specific key.
Input:
let dict = ["name": "John", "age": "30"], key = "name"
Expected Output:
Contains key "name"
Q45. Write a Swift program to get the value for a given key in a dictionary.
Input:
let dict = ["name": "John", "age": "30"], key = "name"
Expected Output:
John
Q46. Write a Swift program to merge two dictionaries.
Input:
let dict1 = ["name": "John"], dict2 = ["age": "30"]
Expected Output:
["name": "John", "age": "30"]
Q47. Write a Swift program to print all keys and values of a dictionary.
Input:
let dict = ["name": "John", "age": "30"]
Expected Output:
name: John
age: 30
Q48. Write a Swift program to find if a number is present in a dictionary value.
Input:
let dict = ["name": "John", "age": "30"], value = "30"
Expected Output:
Found value 30
Q49. Write a Swift program to create and access a tuple.
Input:
let tuple = (1, "Hello")
Expected Output:
1, Hello
Q50. Write a Swift program to return multiple values from a function using a tuple.
Input:
func getDetails() -> (Int, String) { return (1, "John") }
Expected Output:
1, John

Bikki Singh
Hi, I am the instructor of TechnoVlogs. I have a strong love for programming and enjoy teaching through practical examples. I made this site to help people improve their coding skills by solving real-world problems. With years of experience, my goal is to make learning programming easy and fun for everyone. Let's learn and grow together!