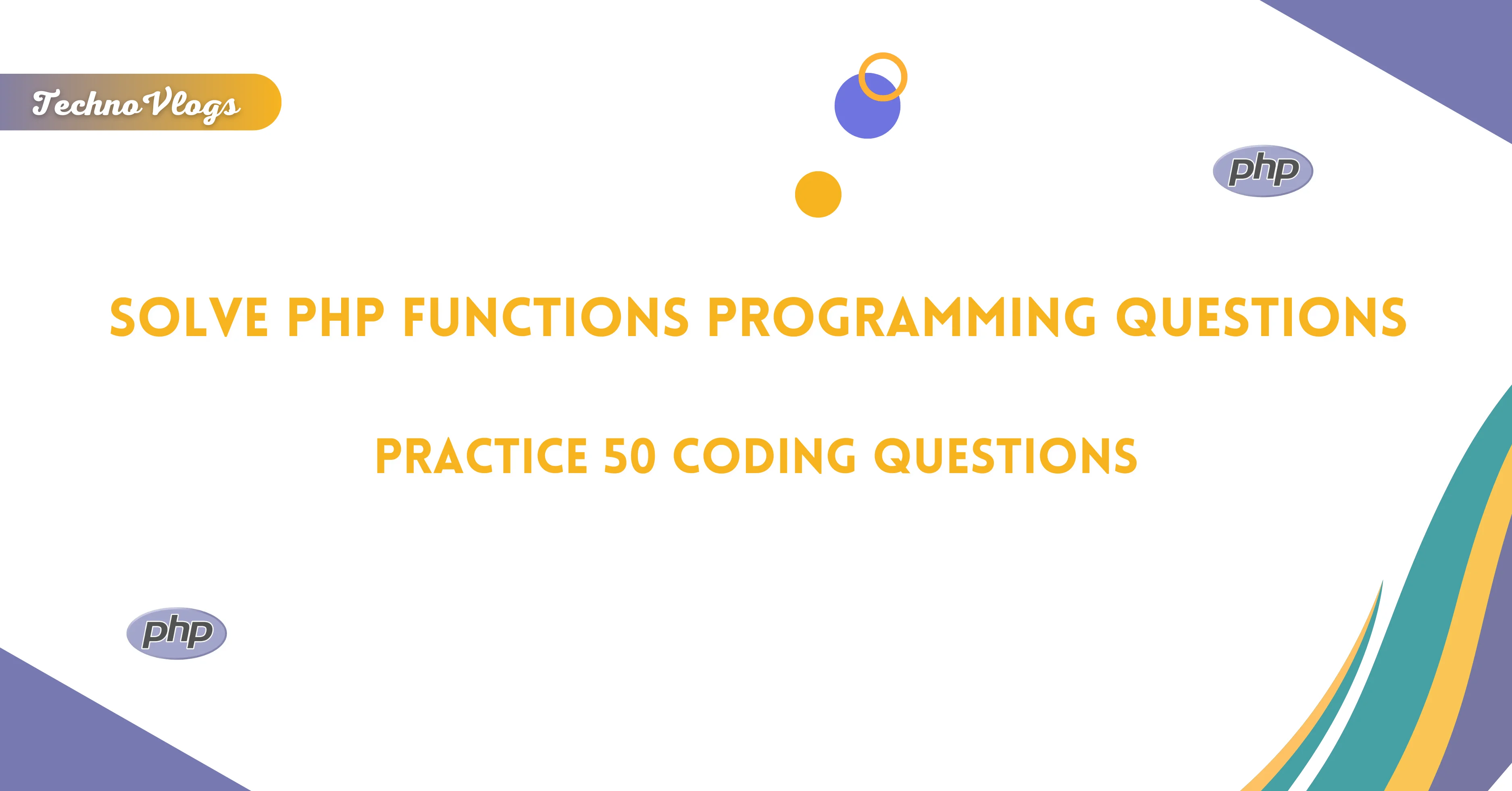
Practice 50 PHP Functions Programming Questions
Q1: Write a function to add two numbers and return the result.
Input: add(5, 10)
Expected Output:
15
Q2: Write a function to check if a number is even or odd.
Input: checkEvenOdd(7)
Expected Output:
Odd
Q3: Write a function to calculate the square of a number.
Input: square(4)
Expected Output:
16
Q4: Write a function to return the larger of two numbers.
Input: maxNumber(8, 3)
Expected Output:
8
Q5: Write a function to reverse a string.
Input: reverseString("Hello")
Expected Output:
olleH
Q6: Write a function to check if a string is a palindrome.
Input: isPalindrome("radar")
Expected Output:
True
Q7: Write a function to find the factorial of a number.
Input: factorial(5)
Expected Output:
120
Q8: Write a function to calculate the power of a number.
Input: power(2, 3)
Expected Output:
8
Q9: Write a function to convert Celsius to Fahrenheit.
Input: celsiusToFahrenheit(25)
Expected Output:
77
Q10: Write a function to check if a number is prime.
Input: isPrime(13)
Expected Output:
True
Q11: Write a function to find the sum of all elements in an array.
Input: sumArray([1, 2, 3, 4, 5])
Expected Output:
15
Q12: Write a function to find the largest number in an array.
Input: maxArray([3, 5, 7, 2, 8])
Expected Output:
8
Q13: Write a function to count the vowels in a string.
Input: countVowels("Hello World")
Expected Output:
3
Q14: Write a function to generate the Fibonacci series up to N terms.
Input: fibonacci(5)
Expected Output:
0 1 1 2 3
Q15: Write a function to return the length of a string.
Input: stringLength("Programming")
Expected Output:
11
Q16: Write a function to check if a number is positive, negative, or zero.
Input: checkNumber(-5)
Expected Output:
Negative
Q17: Write a function to calculate the area of a circle.
Input: circleArea(7)
Expected Output:
153.93804002589985
Q18: Write a function to find the second largest number in an array.
Input: secondLargest([10, 20, 15, 30, 25])
Expected Output:
25
Q19: Write a function to check if a string contains only alphabets.
Input: isAlphabet("Hello123")
Expected Output:
False
Q20: Write a function to return the sum of digits of a number.
Input: sumOfDigits(12345)
Expected Output:
15
Q21: Write a function to return the maximum and minimum elements in an array.
Input: findMinMax([4, 7, 2, 9, 1])
Expected Output:
Max = 9, Min = 1
Q22: Write a function to calculate the GCD of two numbers.
Input: gcd(12, 18)
Expected Output:
6
Q23: Write a function to calculate the LCM of two numbers.
Input: lcm(4, 6)
Expected Output:
12
Q24: Write a function to capitalize the first letter of each word in a string.
Input: capitalizeWords("hello world")
Expected Output:
Hello World
Q25: Write a function to check if a number is an Armstrong number.
Input: isArmstrong(153)
Expected Output:
True
Q26: Write a function to sort an array in ascending order.
Input: sortArray([5, 3, 8, 1, 2])
Expected Output:
[1, 2, 3, 5, 8]
Q27: Write a function to merge two arrays into one.
Input: mergeArrays([1, 2], [3, 4])
Expected Output:
[1, 2, 3, 4]
Q28: Write a function to find the first N prime numbers.
Input: firstNPrimes(5)
Expected Output:
[2, 3, 5, 7, 11]
Q29: Write a function to replace all occurrences of a word in a string.
Input: replaceWord("Hello World", "World", "PHP")
Expected Output:
Hello PHP
Q30: Write a function to count the frequency of elements in an array.
Input: countFrequency([1, 2, 2, 3, 3, 3])
Expected Output:
1: 1, 2: 2, 3: 3
Q31: Write a function to remove duplicates from an array.
Input: removeDuplicates([1, 2, 2, 3, 4, 4, 5])
Expected Output:
[1, 2, 3, 4, 5]
Q32: Write a function to check if a string starts with a specific substring.
Input: startsWith("Hello World", "Hello")
Expected Output:
True
Q33: Write a function to check if a string ends with a specific substring.
Input: endsWith("Hello World", "World")
Expected Output:
True
Q34: Write a function to calculate the sum of squares of elements in an array.
Input: sumOfSquares([1, 2, 3, 4])
Expected Output:
30
Q35: Write a function to count the number of words in a string.
Input: wordCount("PHP is fun")
Expected Output:
3
Q36: Write a function to find the index of an element in an array.
Input: findIndex([10, 20, 30, 40], 30)
Expected Output:
2
Q37: Write a function to calculate the average of numbers in an array.
Input: average([10, 20, 30, 40, 50])
Expected Output:
30
Q38: Write a function to determine if a year is a leap year.
Input: isLeapYear(2024)
Expected Output:
True
Q39: Write a function to concatenate two strings.
Input: concatStrings("PHP", "Programming")
Expected Output:
PHPProgramming
Q40: Write a function to convert a string to uppercase.
Input: toUpperCase("hello")
Expected Output:
HELLO
Q41: Write a function to convert a string to lowercase.
Input: toLowerCase("HELLO")
Expected Output:
hello
Q42: Write a function to check if an array is sorted in ascending order.
Input: isSorted([1, 2, 3, 4, 5])
Expected Output:
True
Q43: Write a function to find the median of numbers in an array.
Input: median([5, 3, 8, 1, 2])
Expected Output:
3
Q44: Write a function to count the number of occurrences of a character in a string.
Input: countCharacter("Hello World", "o")
Expected Output:
2
Q45: Write a function to check if a number is a perfect number.
Input: isPerfect(28)
Expected Output:
True
Q46: Write a function to return the first N elements of an array.
Input: firstNElements([10, 20, 30, 40, 50], 3)
Expected Output:
[10, 20, 30]
Q47: Write a function to check if an array contains a specific value.
Input: containsValue([1, 2, 3, 4], 3)
Expected Output:
True
Q48: Write a function to split a string into an array of words.
Input: splitString("Learn PHP Functions")
Expected Output:
["Learn", "PHP", "Functions"]
Q49: Write a function to find the intersection of two arrays.
Input: findIntersection([1, 2, 3], [2, 3, 4])
Expected Output:
[2, 3]
Q50: Write a function to find the union of two arrays.
Input: findUnion([1, 2, 3], [2, 3, 4])
Expected Output:
[1, 2, 3, 4]

Bikki Singh
Hi, I am the instructor of TechnoVlogs. I have a strong love for programming and enjoy teaching through practical examples. I made this site to help people improve their coding skills by solving real-world problems. With years of experience, my goal is to make learning programming easy and fun for everyone. Let's learn and grow together!